C# Exception Filters: Everything you need to know [2023]
In C#, an exception filter is a feature that lets us
handle exceptions based on a condition. Exception filters are used with
the combination of catch
and when
keywords. In the when
block, we
specify a boolean expression that determines whether the exception should be
caught. If the expression evaluates to true
, the exception is caught and the
code in the catch block is executed. Otherwise, the exception is passed to the
next catch block.
Microsoft introduced exception filters in 2015 with the release of C# 6.0.
Exception Filters Examples
Catch multiple exceptions
Exception filters let us catch multiple exception types in a single catch block:
try
{
// code that might throw an exception
}
catch (Exception ex) when (ex is ArgumentException || ex is FormatException || ex is OverflowException)
{
// handle ArgumentException, FormatException, and OverflowException exceptions
}
catch (Exception)
{
// handles everything else
}
Complex exception filters example
Complex exception filters example from Microsoft.DotNet.Helix library.
public sealed override bool Execute()
{
try
{
HelixApi = GetHelixApi();
AnonymousApi = ApiFactory.GetAnonymous(BaseUri);
System.Threading.Tasks.Task.Run(() => ExecuteCore(_cancel.Token)).GetAwaiter().GetResult();
}
catch (RestApiException ex) when (ex.Response.Status == (int)HttpStatusCode.Unauthorized)
{
Log.LogError(FailureCategory.Build, "Helix operation returned 'Unauthorized'. Did you forget to set HelixAccessToken?");
}
catch (RestApiException ex) when (ex.Response.Status == (int)HttpStatusCode.Forbidden)
{
Log.LogError(FailureCategory.Build, "Helix operation returned 'Forbidden'.");
}
catch (OperationCanceledException ocex) when (ocex.CancellationToken == _cancel.Token)
{
// Canceled
return false;
}
catch (ArgumentException argEx) when (argEx.Message.StartsWith("Helix API does not contain an entry "))
{
if (FailOnMissingTargetQueue)
{
Log.LogError(FailureCategory.Build, argEx.Message);
}
else
{
Log.LogWarning($"{argEx.Message} (FailOnMissingTargetQueue is false, so this is just a warning.)");
}
}
catch (Exception ex)
{
Log.LogErrorFromException(FailureCategory.Helix, ex, true, true, null);
}
return !Log.HasLoggedErrors;
}
Is it required to reference the exception object in the condition?
It is not required to reference the exception object in the condition inside the when
clause. The condition in the when clause can be any boolean expression.
Here is an example of using an exception filter with a condition that does not reference the exception object:
bool isSkyBlue = true;
try
{
throw new Exception();
}
catch (Exception) when (isSkyBlue)
{
Console.WriteLine("Blue Sky");
}
Output: Blue Sky
Can exception filters be used with custom exceptions?
Exception filters can be used with custom exceptions. Exception filters can handle any exception that is derived from the .NET Exception
class, which includes custom exceptions.
You can specify the type of the custom exception in the catch block and use an exception filter to determine whether to catch the exception. For example:
try
{
// code that might throw a custom exception
}
catch (CustomException ex) when (ex.HttpStatusCode == 404)
{
// handle CustomException exceptions with an HTTP code of 404
}
Exception filters are especially useful with a custom exception because custom exceptions contain more data that we want to check.
Exception Filters Cheat Sheet
The table below summarizes the most important concepts of C# exceptions:
Concept | Description |
---|---|
Exception filters | A feature of the C# language that lets you to specify a boolean
expression that will be evaluated to determine whether an exception
should be caught. Exception filters are used with the |
Syntax | The syntax for using exception filters is
|
Handling different types of exceptions | You can use exception filters to handle multiple types of exceptions in the same way. |
Performing additional checks | Exception filters can be used to perform additional checks before catching an exception. For example, you might want to check the value of a property of the exception object before deciding whether to catch the exception. |
Making exception handling code more concise | The biggest advantage of exception filters is making your exception-handling code more concise by
allowing you to specify multiple conditions in a single
|
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →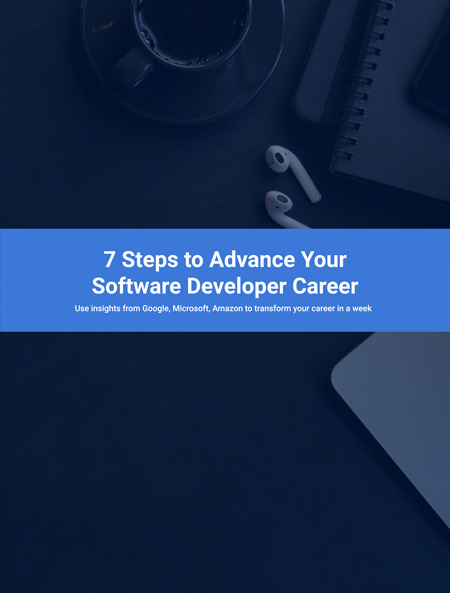
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.