How to rename a dictionary key in C#?
Rename a dictionary key in C#
To rename a key in a dictionary in C#, you can first remove the key-value pair with the old key, and then add a new key-value pair with the new key and the same value.
Here's an example code of how you can do this:
Dictionary<string, string> dictionary = new Dictionary<string, string>();
dictionary.Add("oldKey", "value");
string value = dictionary["oldKey"];
dictionary.Remove("oldKey");
dictionary.Add("newKey", value);
Alternatively, you can use the TryGetValue method to get the value associated with the old key, and then use the Add method to add the new key-value pair:
Dictionary<string, string> dictionary = new Dictionary<string, string>();
dictionary.Add("oldKey", "value");
string value;
if (dictionary.TryGetValue("oldKey", out value))
{
dictionary.Remove("oldKey");
dictionary.Add("newKey", value);
}
Keep in mind that dictionaries do not allow duplicate keys, so if you try to add a new key-value pair with a key that already exists in the dictionary, the Add method will throw an exception.
Extension method to rename dictionary key
You can create an extension method to rename the key in a dictionary. Here's an example:
public static class DictionaryExtensions
{
public static void RenameKey<TKey, TValue>(this IDictionary<TKey, TValue> dictionary, TKey oldKey, TKey newKey)
{
if (dictionary == null)
{
throw new ArgumentNullException(nameof(dictionary));
}
if (EqualityComparer<TKey>.Default.Equals(oldKey, newKey))
{
return;
}
TValue value;
if (dictionary.TryGetValue(oldKey, out value))
{
if (dictionary.ContainsKey(newKey))
{
throw new ArgumentException("The new key already exists in the dictionary.");
}
dictionary.Remove(oldKey);
dictionary.Add(newKey, value);
}
}
}
The RenameKey
method first checks whether the dictionary is null and throws an exception if it is. It also checks whether the old key and the new key are equal and does nothing if they are. It then uses the TryGetValue method to get the value associated with the old key and throws an exception if the new key already exists in the dictionary. Finally, it removes the old key-value pair and adds a new key-value pair with the new key and the same value.
Rename dictionary key in multi-thread applications
You can use a lock or other synchronization mechanism to make the RenameKey
extension method thread-safe.
Here's an example of how you can rename a dictionary key in multi-threaded applications using a lock statement:
public static class DictionaryExtensions
{
private static readonly object SyncRoot = new object();
public static void RenameKey<TKey, TValue>(this IDictionary<TKey, TValue> dictionary, TKey oldKey, TKey newKey)
{
if (dictionary == null)
{
throw new ArgumentNullException(nameof(dictionary));
}
if (EqualityComparer<TKey>.Default.Equals(oldKey, newKey))
{
return;
}
lock (SyncRoot)
{
TValue value;
if (dictionary.TryGetValue(oldKey, out value))
{
if (dictionary.ContainsKey(newKey))
{
throw new ArgumentException("The new key already exists in the dictionary.");
}
dictionary.Remove(oldKey);
dictionary.Add(newKey, value);
}
}
}
}
This version of the RenameKey method uses a private static SyncRoot object to synchronize access to the dictionary. The entire rename operation is enclosed in a lock statement that acquires a lock on the SyncRoot object, ensuring that only one thread can execute the operation at a time. This makes the method thread-safe.
Keep in mind that using a lock statement can have a performance impact, especially if the dictionary is being accessed frequently by multiple threads. If you need to optimize for performance, you may want to consider using a different synchronization mechanism, such as a ReaderWriterLockSlim
or a ConcurrentDictionary
.
FAQ: Rename dictionary key in C#
Can I rename a key in a dictionary in place, without creating a new key-value pair?
No, C# dictionaries currently do not provide a way to rename a key in place.
The only way to rename a key is to remove the key-value pair with the old key and add a new key-value pair with the new key and the same value.
Can I rename a key in a dictionary using LINQ?
Yes, you can use LINQ to rename a key in a dictionary. Here's an example of how you can do this:
Dictionary<string, string> dictionary = new Dictionary<string, string>();
dictionary.Add("oldKey", "value");
dictionary = dictionary
.Select(kvp => new KeyValuePair<string, string>(kvp.Key == "oldKey" ? "newKey" : kvp.Key, kvp.Value))
.ToDictionary(kvp => kvp.Key, kvp => kvp.Value);
This code creates a new dictionary by converting the original dictionary to a list of key-value pairs, using LINQ to transform the key-value pairs by replacing the old key with the new key, and then converting the list back to a dictionary. This approach can be more efficient than the previous approaches if you have a large number of keys to rename, as it only requires a single pass over the dictionary. However, it may not be as readable as the other approaches.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
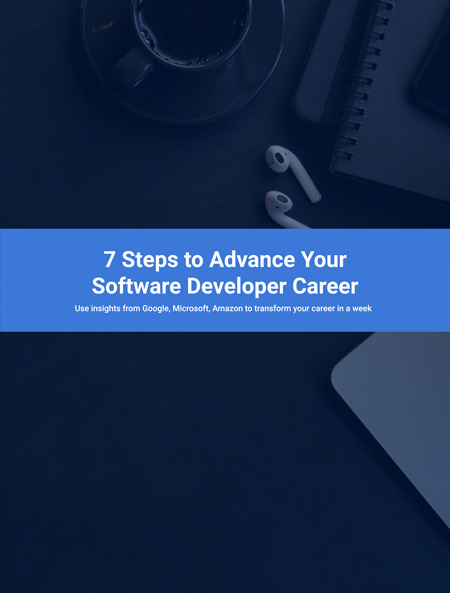
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.