Difference between GraphQL and REST
The main difference between GraphQL and REST is that GraphQL is focused on being a utility for data access, while REST is a design principle for scalable web services.
REST API principles originate from the late 1990s, while GraphQL was introduced in 2012 to address the limitations of REST.
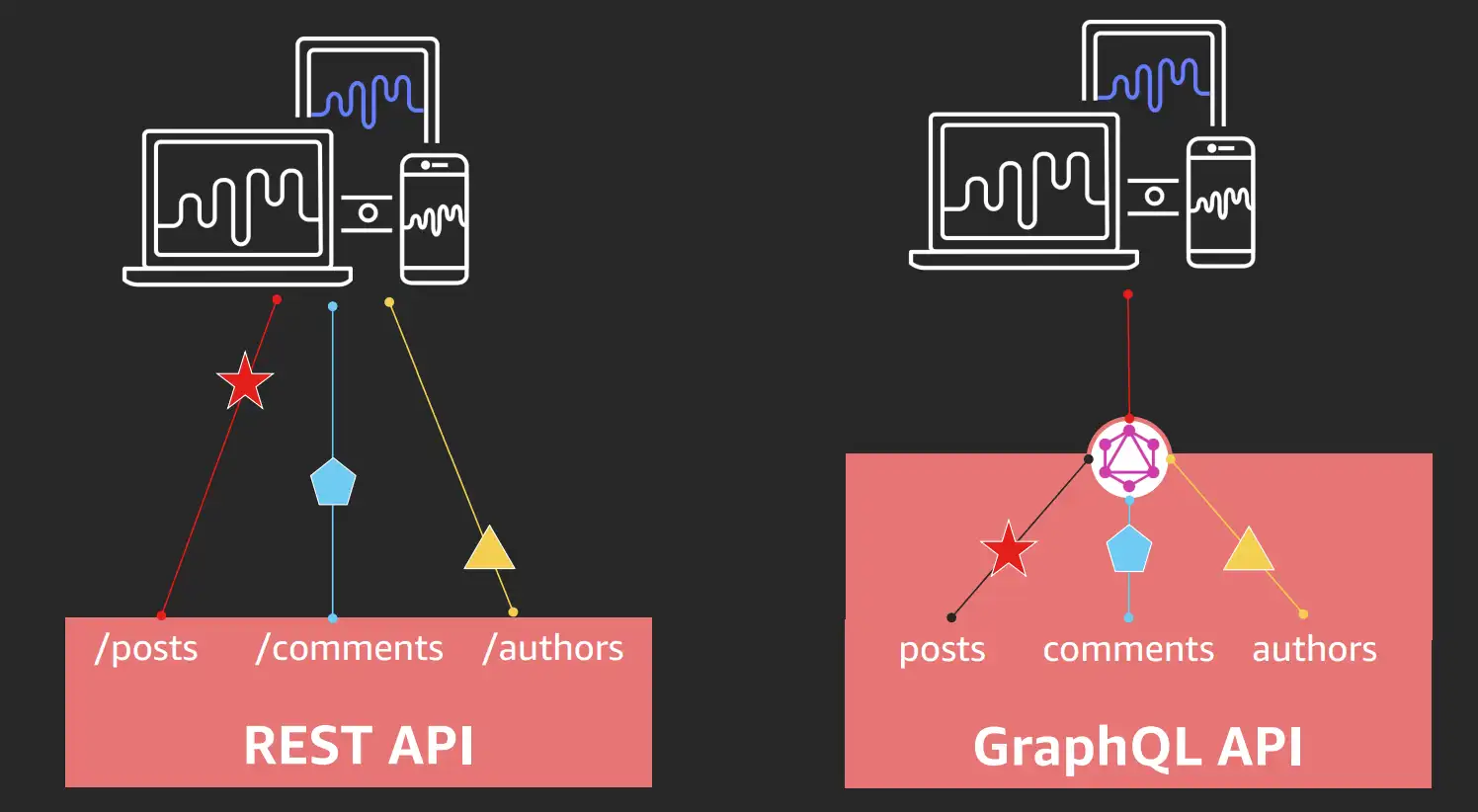
GraphQL is more efficient than REST because it gets the exact information the client needs while REST includes the entire resource.
Feature | GraphQL | REST |
---|---|---|
Purpose | Query language | Design principle |
Data fetching | Pulls data from a database | Works with domain-level resources |
API structure | Client can request exactly what it needs | Fixed structure with defined endpoints for each resource |
# of requests | Single request | Multiple round trips |
Popularity | 24% 1 | 91% 1 |
Introduced in | 2012 | 2000 |
Bandwidth usage | Low | High |
Caching | Difficult | Easy |
Versioning | Versionless | Versioned |
Media type | Limited to JSON | Can use all HTTP media types |
Client-server coupling | More coupled | Less coupled |
Data control | Gives more control over returned data | Over-fetching or under-fetching issues |
Error handling | Communicated through the response structure | Uses HTTP Status codes |
- What is a REST API?
- What is GraphQL?
- How does GraphQL work?
- How does REST work?
- Disadvantages of REST that inspired GraphQL
- Differences between GraphQL and REST
- Bandwidth
- Caching
- Versioning
- Data Types
- Client-server coupling
- Complexity
- Performance
- Error Handling
- Popularity
- Should I pick REST or GraphQL?
- REST vs GraphQL: Differences Summary
What is a REST API?
REST is an architecture style for designing APIs.
Roy Fielding defined RESTful API design in 2000 in his Ph.D. dissertation at the University of California, Irvine.
The key components that define RESTf are:
- Identification of resources: Unique URIs.
- Manipulation of resources through representations: Resources can be represented in different formats as defined by Media Types (e.g. JSON, XML, image)
- Self-descriptive messages: Client-server communication uses features of the HTTP protocol to provide metadata (e.g. HTTP Methods, Headers, Content Negotiation)
- Hypermedia as the engine of application state: The client navigates through the API using links included in the resource representations.
What is GraphQL?
GraphQL is an open-source query language for retrieving data through APIs.
It was designed to present data in a more concise and usable format, using objects and properties, instead of relying on individual REST API calls.
Facebook engineers Lee Byron, Dan Schafer and Nick Schrock created GraphQL in 2012 to address the limitations of REST API in delivering data to their mobile app.
In 2015, it was open-sourced and quickly adopted by other companies such as Airbnb and GitHub.
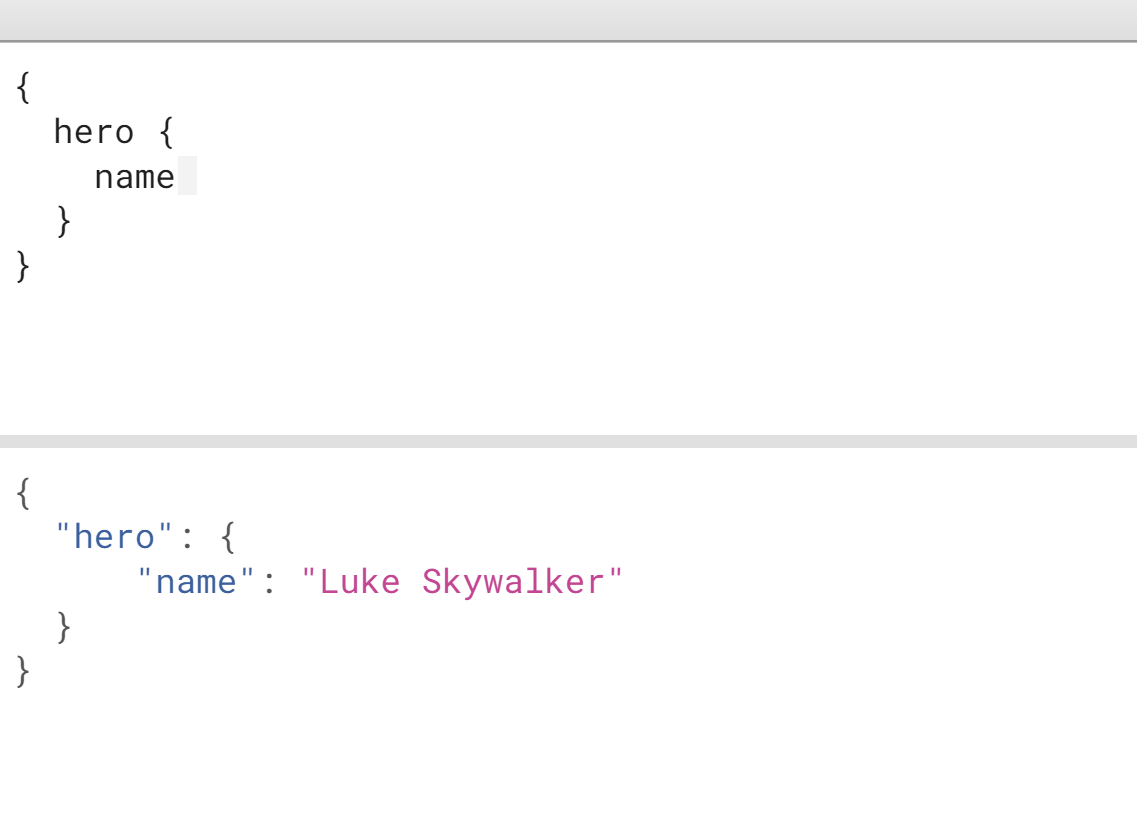
How does GraphQL work?
From the client's perspective, GraphQL works as follows:
- Building a schema: Schema is a description of all the possible queries and types that a GraphQL API returns. The schema requires strong typing using the Schema Definition Language (SDL).
- Validating queries: Before making a query, clients can validate their queries against the schema to ensure that the server can respond to it.
- Sending a single query: The client sends a single query to the server.
- Interpreting operations: The server interprets the query against the entire schema.
- Resolving data: GraphQL operation gathers the requested data from various data sources.
- JSON response: The API returns a JSON response with exactly the shape of the data requested.
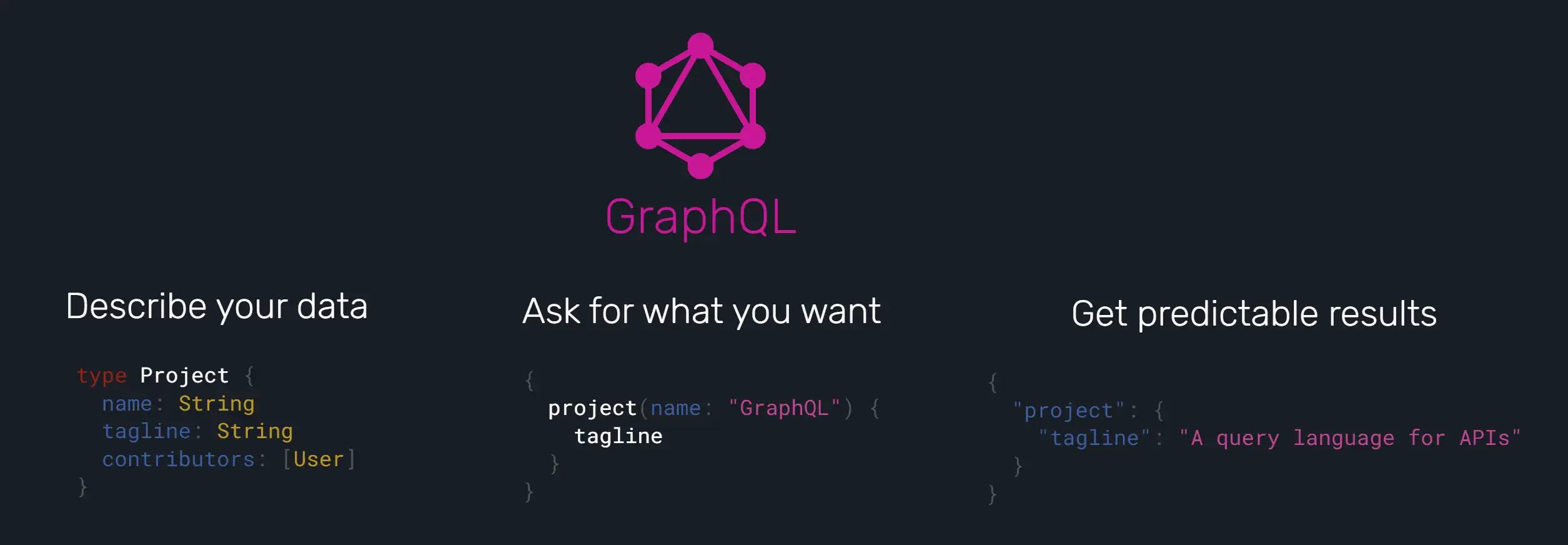
How does REST work?
From the client's perspective, REST works as follows:
- Identify the resource that needs to be accessed or manipulated
- Choose an appropriate HTTP method (e.g. GET, POST, PUT, DELETE) based on the desired action to be performed on the resource
- Construct the request URL based on the resource identifier and any query parameters needed
- Send the request to the server
- Parse the response received from the server which is in the form of an HTTP response with a status code, headers and the response body (usually in JSON or XML format)
Disadvantages of REST that inspired GraphQL
The main disadvantage of REST design is a standardized form of representing resources.
Even Roy Fielding recognized it in his dissertation:
The trade-off, though, is that a uniform interface degrades efficiency, since information is transferred in a standardized form rather than one which is specific to an application’s needs. - Roy Thomas Fielding
This standardized form leads to over-fetching or under-fetching of data.
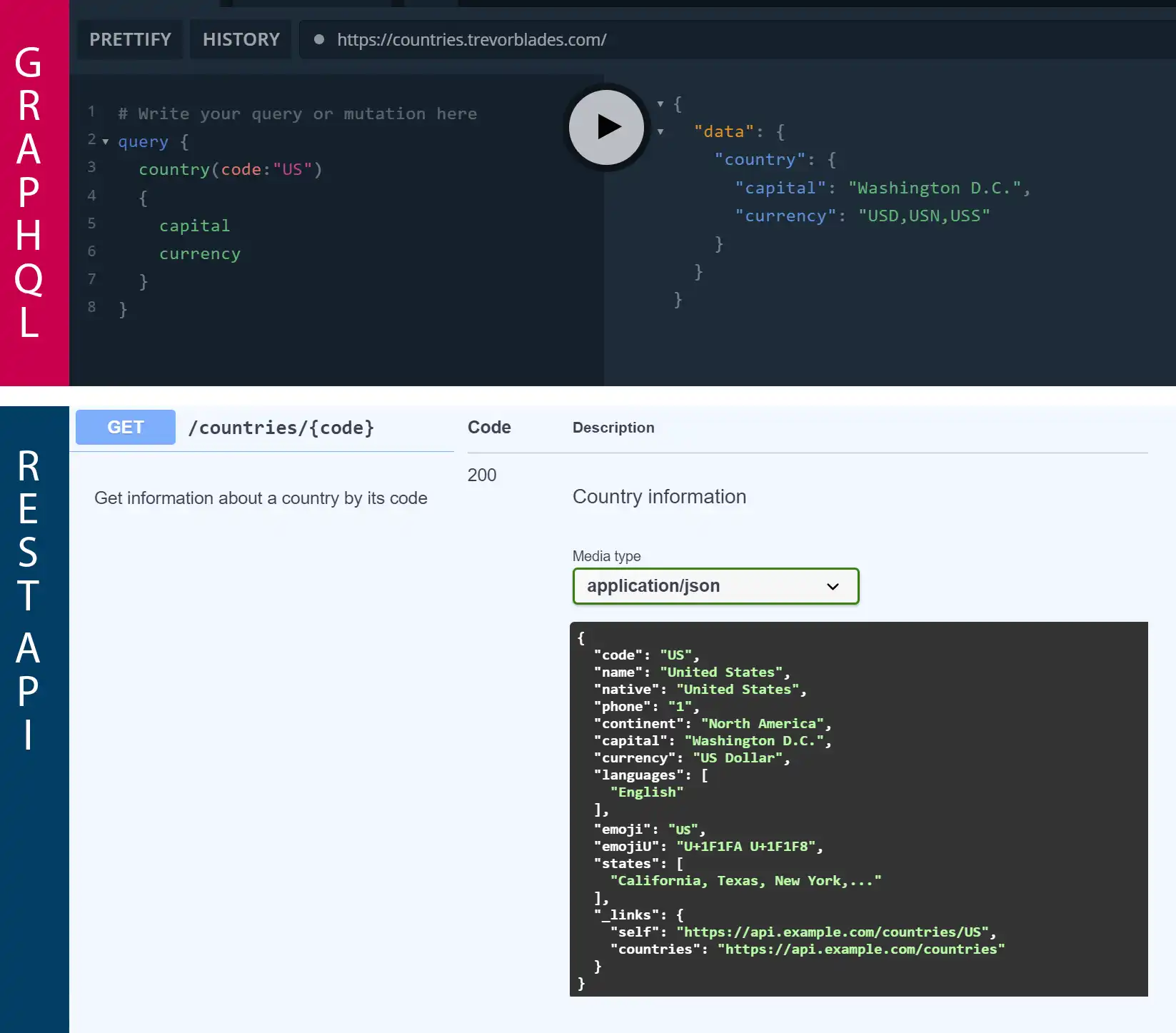
Differences between GraphQL and REST
Can you even compare REST and GraphQL?
GraphQL is focused on being a utility for data access, while REST is a design principle for scalable web services.
Roy T. Fielding's take on GraphQL:
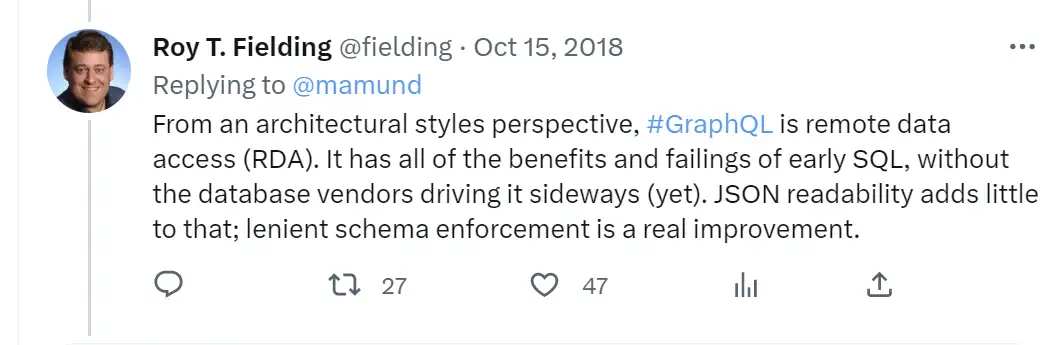
Detailed comparison of GraphQL and REST:
Bandwidth
REST has high bandwidth usage because of the chatty interface. That's exactly why Facebook engineers created GraphQL. They need to reduce bandwidth usage for the mobile app.
GraphQL can reduce the size of the returned JSON documents by 94% in terms of the number of fields and 99% in terms of bytes, according to the study of 24 queries performed by seven open-source clients of two popular REST APIs (GitHub and arXiv) and 14 queries performed by seven empirical papers.
The study also shows that it is not straightforward to refactor API clients to use complex GraphQL queries due to the tendency of developers to organize their code around small functions that consume small amounts of data.
Caching
The implementation of caching in GraphQL requires more effort and complexity compared to REST.
Caching in REST is relatively simple as it uses a uniform resource representation. This means that the resources requested can easily be cached and reused without requiring any additional processing.
On the other hand, caching in GraphQL is more complex due to the nature of the queries and representations. GraphQL queries are more complex and can include multiple fields, making it difficult to cache the results of these queries.
Versioning
REST API versioning is a complex topic. Some argue that REST API shouldn't be versioned. But that's far from reality. Most REST APIs use some kind of versioning -- URL, query params, headers, etc.
Versioning is more important for REST than GraphQL APIs because in REST we request the resource and not the individual fields.
GraphQL only returns the data that you explicitly request, so new capabilities can be added through new types and fields. This allows for a common practice of avoiding breaking changes and serving a versionless API.
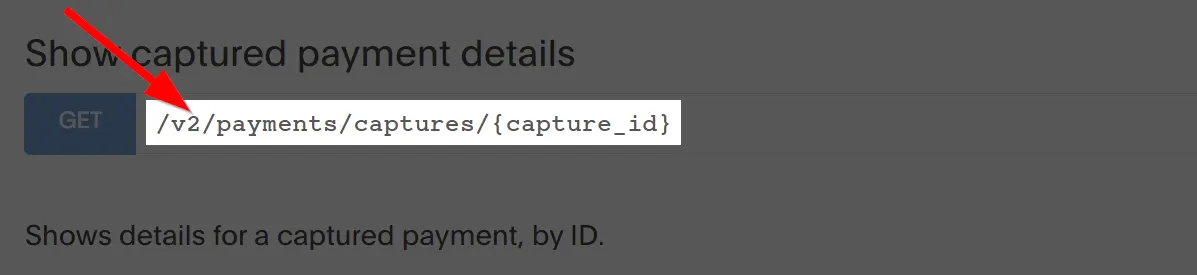
Data Types
GraphQL is limited to JSON as the media type, while REST can use all HTTP media types.
Do you need more than JSON?
Many systems do. Let's say you wanted to transfer an image file.
With REST, you can just respond with the image/jpeg
media type.
On the other hand, GraphQL is more complicated. You can send an image by encoding it as a base64 string and including it as a string in your GraphQL query. Then the client needs to interpret the encoded string to figure out it's an image.
Imagine how poor the Web would have been if we had limited HTML to what was needed by an FTP client. That's what most JSON APIs are today.
— Roy T. Fielding (@fielding) August 26, 2016
Client-server coupling
GraphQL has more coupling between the client and the server because the client needs to understand the schema to make a query.
One of the key principles of REST is Hypermedia as the Engine of Application State (HATEOAS).
HATEOAS is a principle of REST service that adds hypermedia links to REST responses to help discover all available resources.
The advantage of HATEOAS is that you don’t need to know all URIs up front. The API provides them with the rest of data so you can discover actions and resources by inspecting the API’s responses.
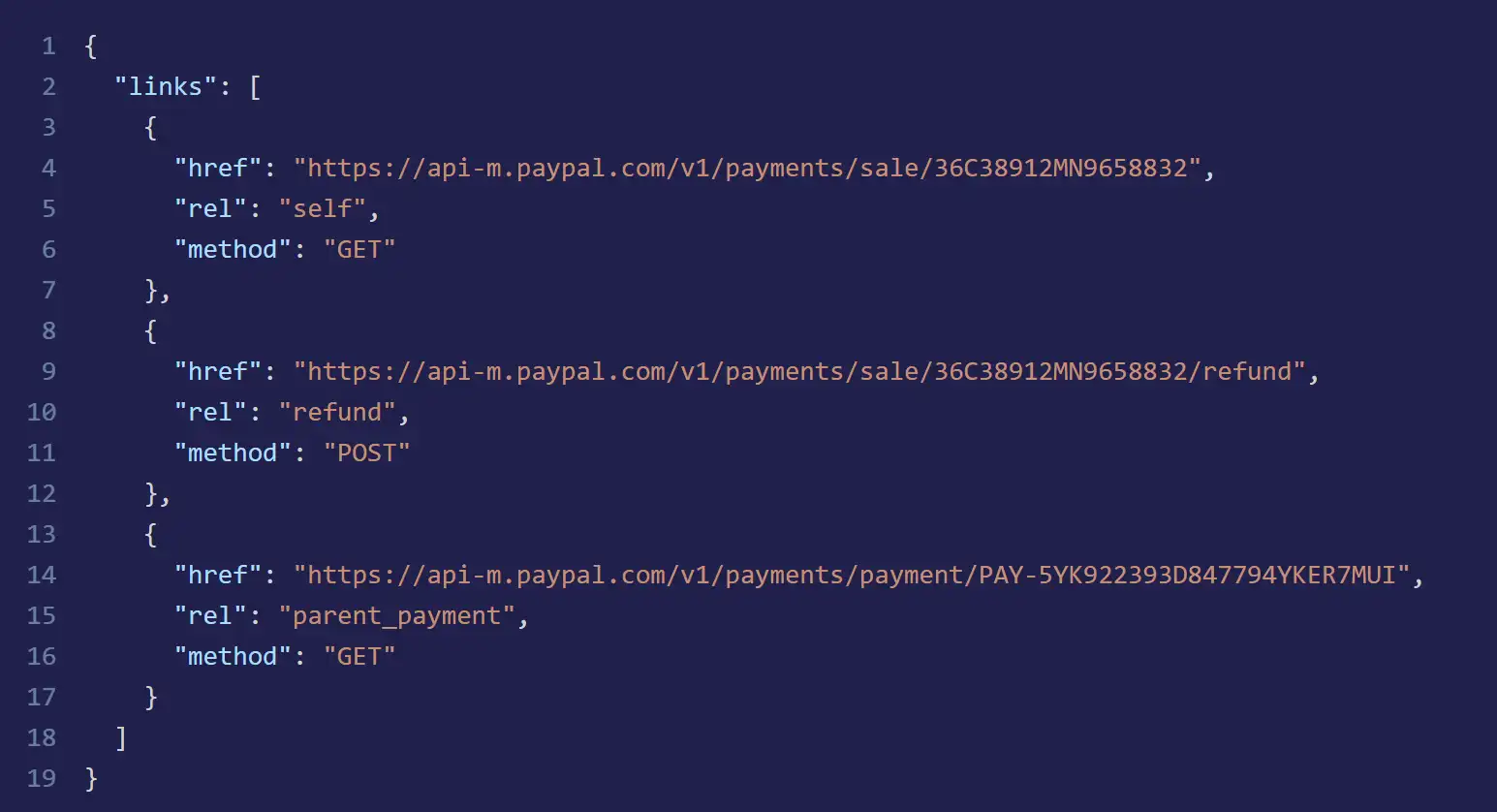
Complexity
With GraphQL, there is a single point of interaction between the client and the server, whereas, with REST, there are multiple endpoints for different operations.
This difference in design can impact the complexity of the API and the way it's used by client applications.
For smaller APIs, GraphQL may seem more complex due to its need for a schema, while REST uses endpoints. But, as the number of endpoints increases, GraphQL's simplicity shines as it allows for querying all data from a single endpoint, reducing the need for multiple requests and endpoints.
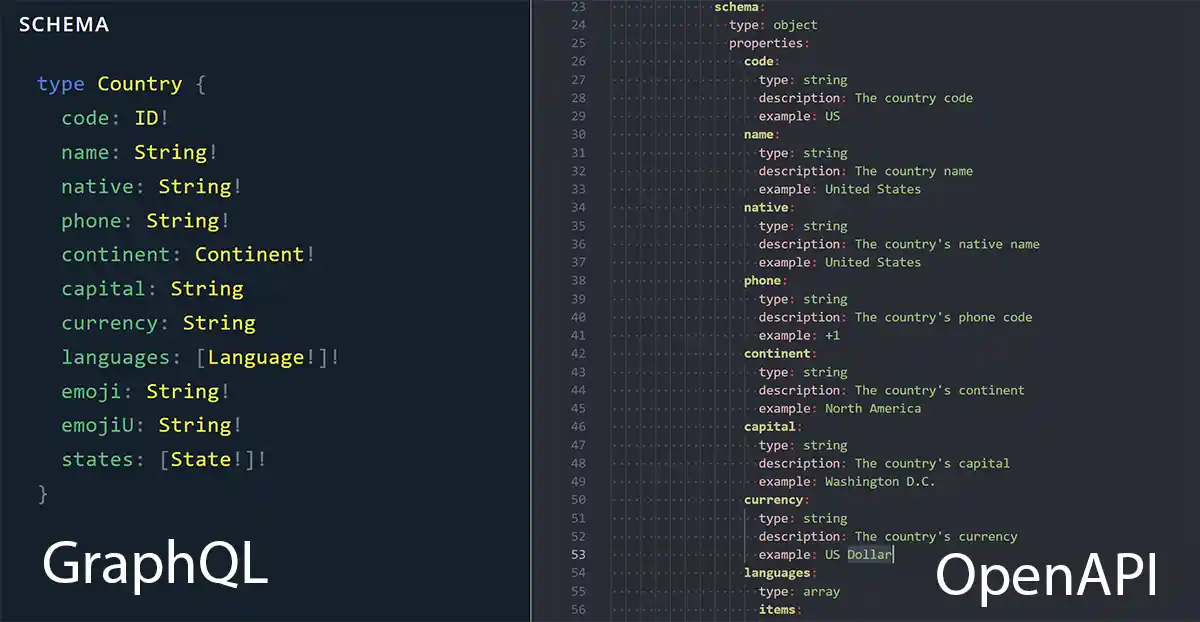
Performance
We can make general conclusions on performance differences because performance depends on the individual system.
But keep this in mind...
GraphQL can be prone to performance issues because it allows for more complex queries, which can be time-consuming and increase the load on the server.
On the other hand, REST has a fixed structure and limits the amount of data that can be requested in a single call, which can help to mitigate performance problems.
Error Handling
REST API error handling is usually done by returning specific HTTP status codes (such as 400 for Bad Request or 500 for Internal Server Error) along with error details in the response body. This makes it easy to map errors to specific categories and monitor them using tools that rely on HTTP status codes.
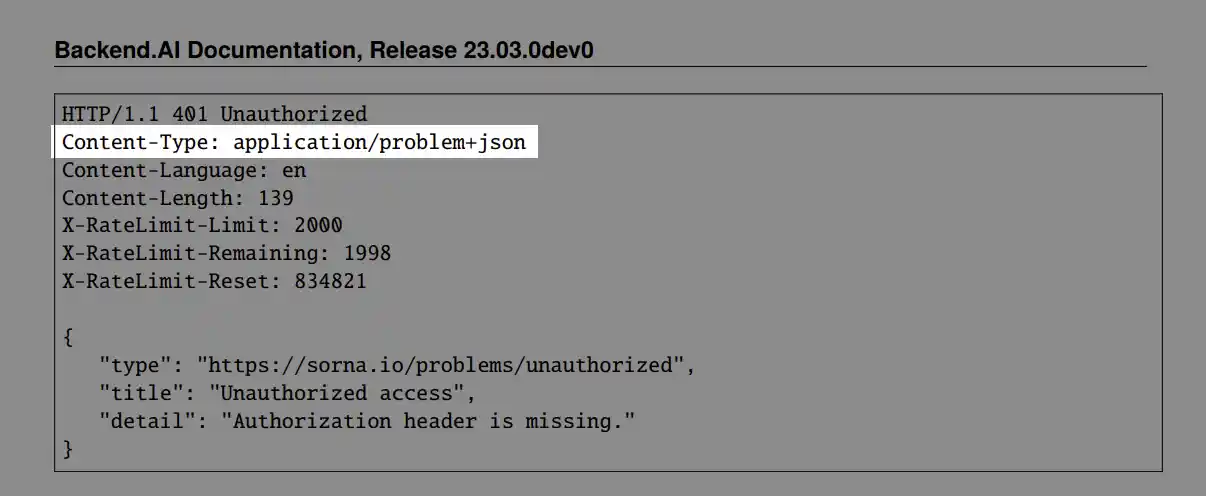
With GraphQL, all responses have a 200 HTTP status code regardless of success or failure.
To indicate errors, the response body includes a "errors" field, which holds an array of error objects. This can make it harder for existing monitoring tools that rely on HTTP status codes to correctly categorize and monitor errors.
Popularity
According to the 2021 survey from Smartbear, 91% of APIs use REST design, compared to 24% that use GraphQL (some systems use both).
REST still dominates with 91% saying they deliver APIs using that style. Perhaps surprisingly, SOAP is still holding strong at number two (57%), which sees an increase from 54% in 2019. Both GraphQL and gRPC have doubled in usage compared to 2019, from 12% to 24% and 4% to 8%, respectively.
Who uses GraphQL?
Several large companies have adopted GraphQL including Airbnb, GitHub, Netflix, and Twitter.
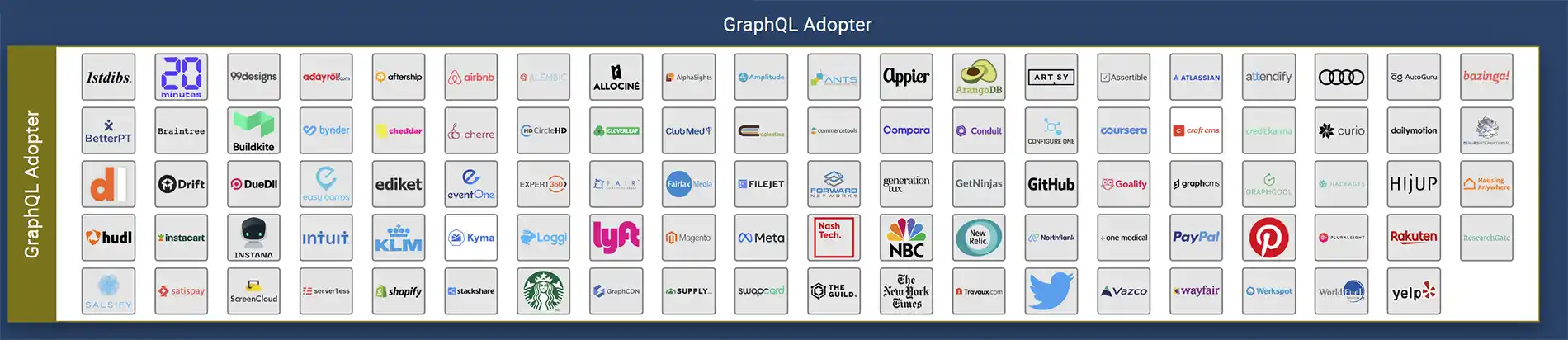
Should I pick REST or GraphQL?
GraphQL is a better fit for complex systems that:
- have nested relational (graph) data,
- pull data from multiple APIs,
- need efficient data loading (e.g. 3G Mobile Apps, PWAs)
The study shows a drastic reduction in the number of fields and size of returned JSON documents when using GraphQL instead of REST-based APIs.
REST vs GraphQL: Differences Summary
-
GraphQL is a query language, while REST is a design principle.
-
GraphQL is more about pulling data from a database, while REST works with domain-level resources that could be different from the underlying data structure.
-
REST APIs typically have a fixed structure, with a defined set of endpoints for each resource, while in GraphQL, the client can request exactly what it needs, making it more flexible.
-
REST APIs may require multiple round trips to fetch all the required data, while GraphQL allows to fetch all the required data in a single request, reducing the number of round trips and improving performance.
-
REST can have over or under-fetching issues, while GraphQL avoids that.
-
GraphQL is more prone to performance issues because it allows for more complex queries, which can be time-consuming and increase the load on the server. On the other hand, REST has a fixed structure and limits the amount of data that can be requested in a single call, which can help to mitigate performance problems.
-
GraphQL has more coupling between client and the server because the client needs to understand the schema to make a query.
-
GraphQL generates developer documentation using a standard schema definition, while REST APIs have various non-standard options such as OpenAPI, RAML, Postman, Blueprint, etc.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →