Next.js: Global CSS cannot be imported from files other than your Custom <App>
When migrating to Next.js 9+, I was running into an issue where I could not import global styles from any file other than the Next JS _app.js
. I would get the following error:
Error
Global CSS cannot be imported from files other than your Custom <App\>
. Please move all global CSS imports to pages/_app.js.
Using Global CSS was not working because since Next.js 9.2 we must import global CSS from Custom <App>
component.
Why the error happens
There are a few things that cause this error to happen in Next.js framework:
- Importing Global CSS from a file other than pages/_app.js,
- Using custom CSS/SASS loaders instead of Next.js' built-in ones,
- Next.config.js misconfiguration
The styles (styles.css) defined in these files will apply to all pages and components in your application. Because stylesheets are global, you may only include them in pages/app.js to avoid overlap between components.
The built-in CSS support bundles global styles into a minified file that will automatically be included on pages.
Separating global and component-level styles allows you to share a common external CSS file between components while moving the shared styles into a single, reusable file that is not included on each component page.
Modular CSS imports allow for advanced optimizations with style splitting and caching that help with page speed.
How to fix the Global CSS error
There are several ways to fix this CSS error in Next.js.
Import global CSS from _app.js
To add a stylesheet to your Next.js app, include the CSS file in pages/_app.js
.
import '../styles.css'
export default function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />
}
The styles (styles.css) in your application will apply to all web pages and components. So it's important to only include them in pages/_app.js to avoid conflict with other components.
Note: If you are using typescript, include the CSS file in ./pages/_app.tsx
.
Use built-in CSS loader
Since, version 9.2, Next.js allows you to import Sass files with both the .scss and .sass file extensions.
If you were using custom loaders such as @zeit/next-css
and @zeit/next-sass
, you'll need to remove them.
Remove the custom loader from your next.config.js file which takes care of importing the stylesheet:
Before:
// next.config.js
const withCSS = require('@zeit/next-css')
module.exports = withCSS({/* my next config */})
After:
// next.config.js
module.exports = {/* my next config */}
Before you can use Next.js' built-in Sass support, install npm package sass
:
npm install sass
Under the hood, Next.js will use sass
to compile your stylesheets into css files which will be included in the app bundle.
Fix Next misconfiguration
Global CSS errors can happen because of the improper configuration of next.config.js file.
If you are using next.config.js
, make sure it is configured with the proper module exports.
Make sure there are no:
Syntax errors
Multiple module.exports
Custom babel processors that impact style files. Check babel.config.js
or .babelrc.json
Use custom CSS loader
You can use custom CSS loader as well. To do that, make sure to overwrite the default style loader with your own in next.config.js file:
module.exports = {
webpack: (config, { buildId, dev, isServer, defaultLoaders, webpack }) => {
// Filter out default Next.js CSS rules:
config.module.rules = config.module.rules.filter(r => !r.oneOf);
// Add your own rules
config.module.rules.push({
test: /\.scss$/i,
use: ['style-loader', 'css-loader'],
})
return config
},
}
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
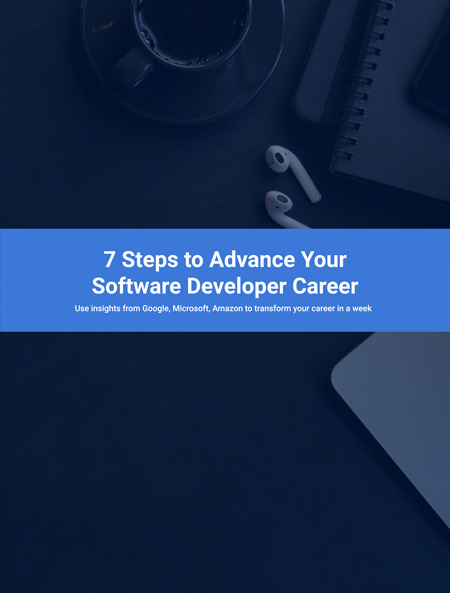
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.