C# String Length: How to use it?
How to get string length in C#?
To get the string length in C#, use the .Length property.
For example:
string c = "Hello World!";
int stringLength = c.Length; // 12 characters in string Hello World!
Examples
using System;
public class Program
{
public static void Main()
{
Console.WriteLine("Hello World".Length); // 11
string unicode = "\u1F642"; // represents 🙂
Console.WriteLine(unicode.Length); // 2
string str = "";
Console.WriteLine(str.Length); // 0
Console.WriteLine(string.Empty.Length); // 0
}
}
What is String Length in C#?
String Length in C# is a property on the String object that returns the number of characters in a string. The returned length value is of type Int32.
The Length property of a string is the number of Char objects it contains, not the number of Unicode characters.
The length property returns the number of Char objects instead of the number of Unicode characters because a Unicode character might be represented by more than one character.
What is the max string length in C#?
The maximum string length in C# is 2^31 characters. That's because String.Length is a 32-bit integer.
How to change string length in C#?
You can't change the string length in C#. The String.Length property is read-only.
To change the string length, you need to create a new string.
If you try to change the Length property, you will get an error:
error CS0200: Property or indexer 'string.Length' cannot be assigned to -- it is read only
How to access each Unicode character?
To access each Unicode character in a C# string, you use the System.Globalization.StringInfo class to work with each Unicode character instead of each character.
Exception
One of the most frequent null-reference exceptions is with String.Length property.
If you try to access the Length property on a null string, you will get a NullReferenceException.
Many developers forget to check for null before accessing the Length property.
Index vs Length
In C#, string length returns the number of characters in a string.
The index property returns the zero-based position of the character that is located at a specified location within an instance of String.
The length property doesn't start at 0. It starts at the first character in the string. But the index property starts at 0.
string test = "12345";
Console.WriteLine(test.IndexOf("1")); // 0
Under the hood
Internally, C# stores strings as a read-only collection of Char
objects. There's also a null-terminating character at the end of a C# string.
C# doesn't count the null-terminating character in the length of a string. The null-character is just a way C# marks the end of a string under the hood.
Termination
Programming languages have different approaches to determine the string end:
- Null-terminated - Strings in C are null-terminated sequences of characters, with a special character following the last character—written "\0" — to show the end of the string.
- Length-prefixed -Pascal stores the length in the first bytes of the string.
- Length-and-Character Array - String as a structure with an array of characters, and stores the length in a separate allocation.
Changes in length
Programming languages have different approaches to handle changes in string length:
- Static string length is fixed and cannot be changed at runtime.
- Limited Dynamic string length can be increased or decreased, but the number of characters that can be stored in it is limited.
- Dynamic string length can grow or shrink as needed, up to the maximum size allowed by the underlying system.
In C#, strings have dynamic string length. It means that the string can grow or shrink as needed.
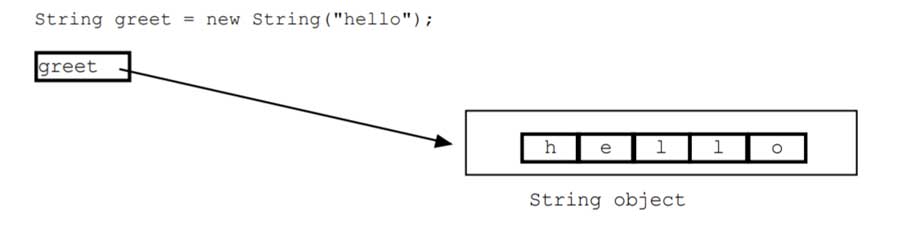
String class
The String class holds the implementation details and the string manipulation functionality. Looking at the source code, we can see that the string length is crucial for the string class.
Functionality such as String.Substring, String.IndexOf, and String.Remove require the length of a string in order to work correctly.
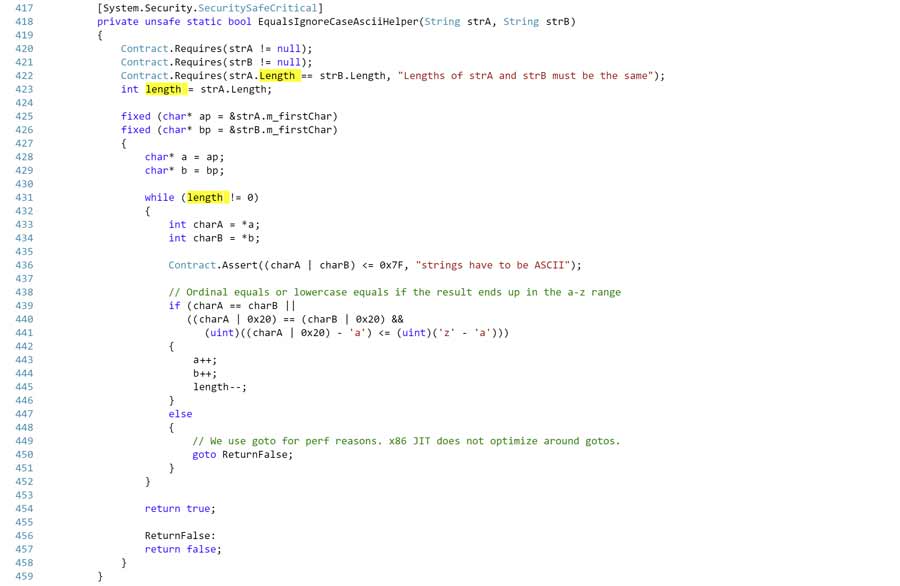
System.String class.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →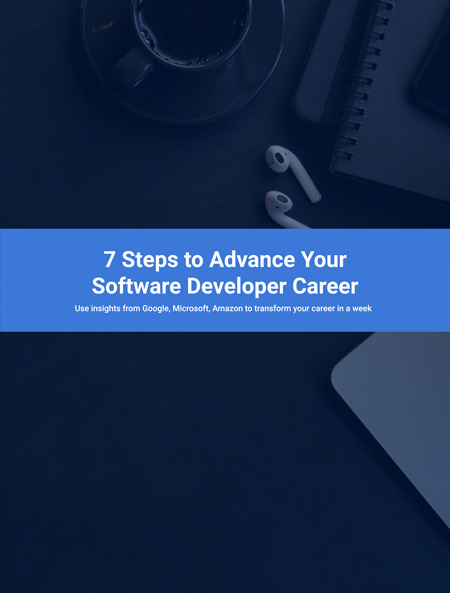
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.