PATCH vs PUT in REST API | Differences between PATCH and PUT
RESTful APIs are everywhere. But if you're a web developer faced with designing a REST API, you know it's hard to decide which HTTP request method is best for various use cases.
Updating a resource is a very common task for REST APIs and there are two HTTP request methods that developers commonly use: PUT and PATCH.
Difference between PUT and PATCH
The main difference between PUT and PATCH in REST API is that PUT handles updates by replacing the entire entity, while PATCH only updates the fields that you give it.
If you use the PUT method, the entire entity will get updated. In most REST APIs, this means it will overwrite any missing fields to null. On the other hand, the PATCH method updates individual fields without overwriting existing fields.
PUT uses more bandwidth because it handles full resources, so PATCH was introduced to reduce the bandwidth usage.
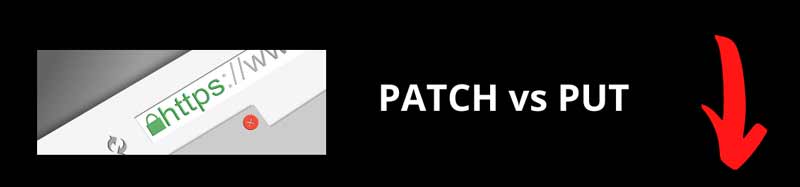
PUT | PATCH | |
---|---|---|
Partial Updates | ❌ | ✔️ |
Bandwidth | ⬆️ | ⬇️ |
Creates a resource | ✔️ | ❌ |
Idempotent | ✔️ | ❌ |
Safe | ❌ | ❌ |
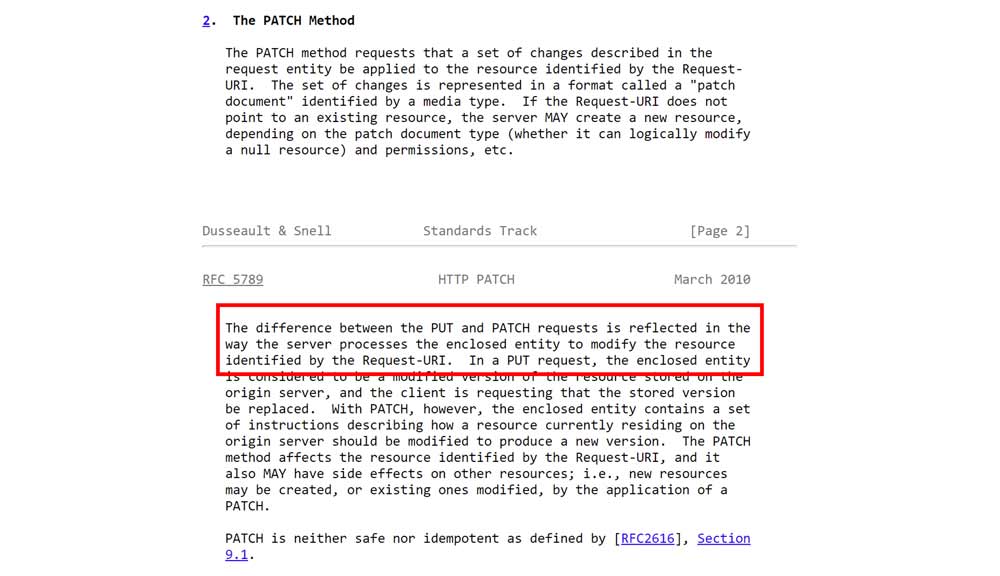
PUT
The HTTP PUT request method is used to create a new resource or replace an existing one.
The IETF has defined the HTTP PUT method as "only allowing a full replacement of a document."
The client must send a complete entity in a request body with all the new values for the resource.
- client provides the resource ID
- if the resource exists, replace it with the inbound data
- if it doesn't exist, create a new one
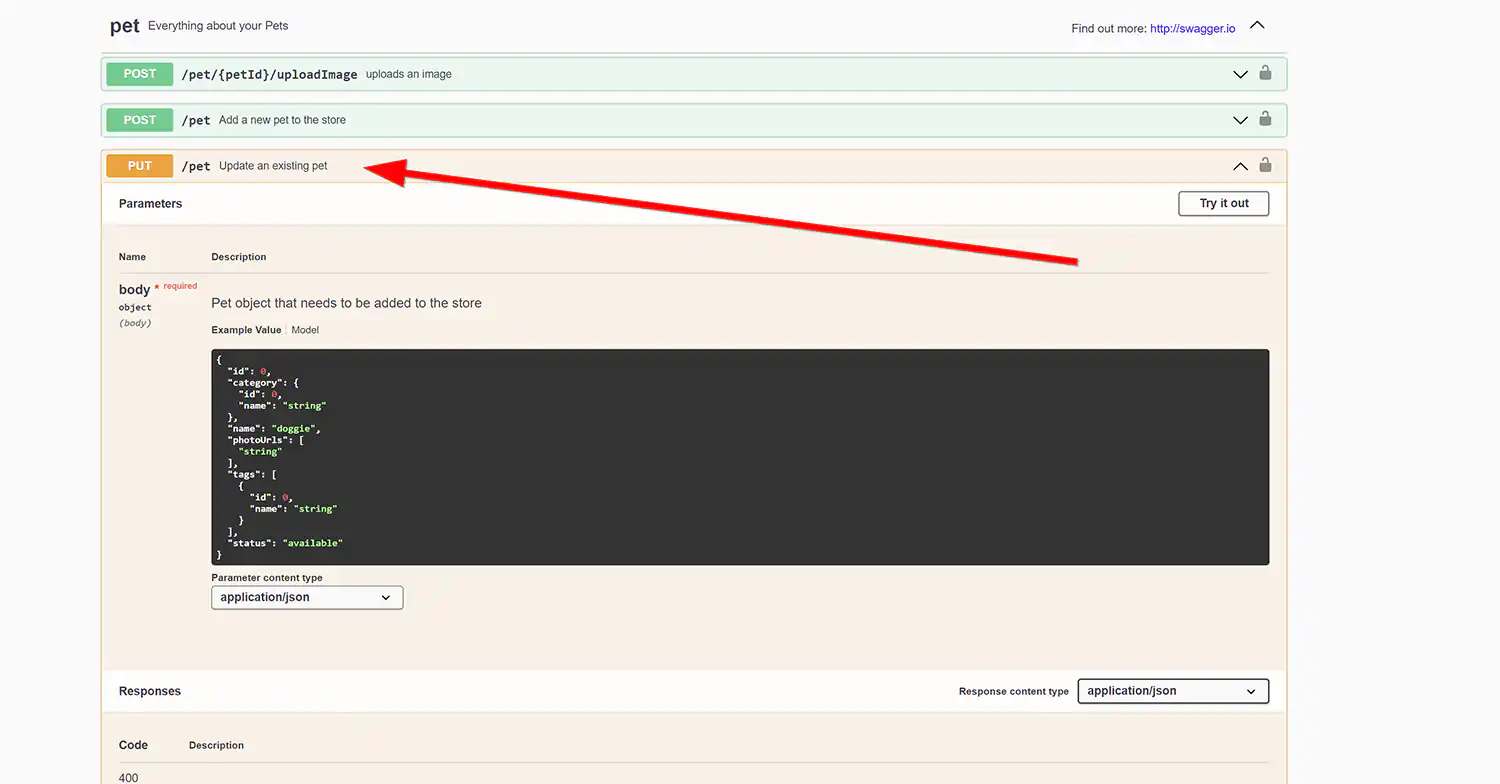
Advantage of using PUT
- We don't have to re-fetch the entity after successfully updating it. Since PUT sends the entire entity, it'll replace whatever is there with what we send.
- We can always overwrite the complete entity if we send down all fields and do not include any delta modification to existing fields.
- PUT creates a resource if one doesn't exist.
PATCH
PATCH is used to change or add data to existing resources.
PATCH only updates the fields that we pass, while the HTTP PUT method updates the entire resource at once.
HTTP PATCH method was added to the HTTP protocol in 2010 to overcome the limitations of HTTP PUT requests, which provide no support for partial updates.
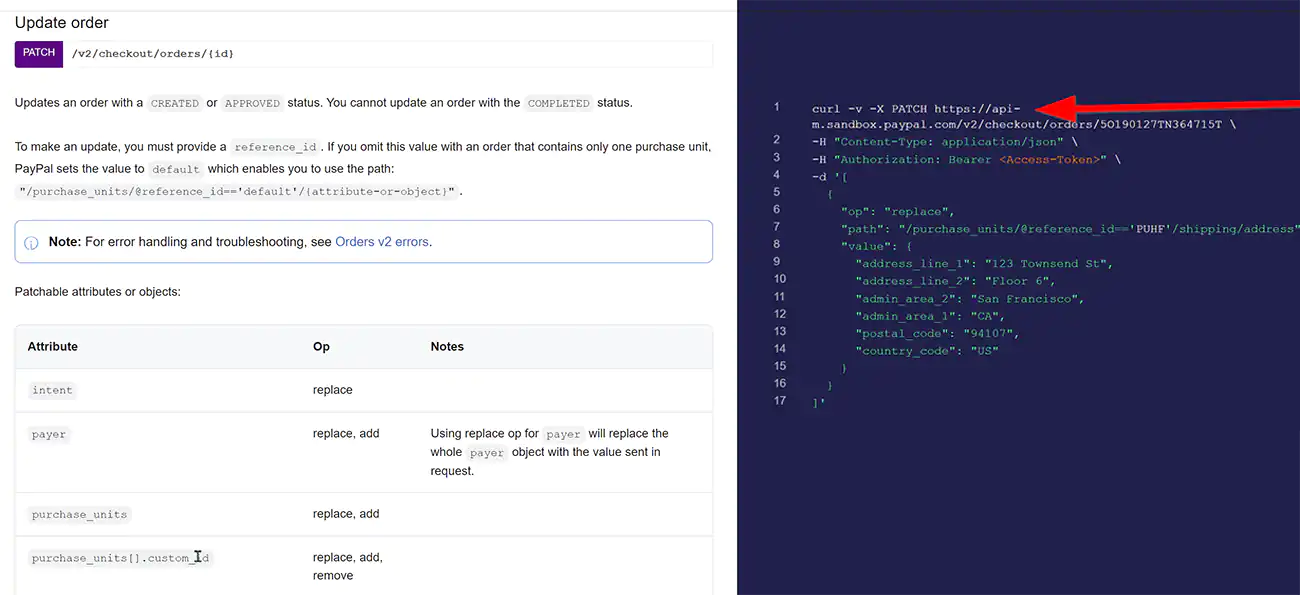
Advantage of using PATCH
- The client doesn't need to know the complete state of the resource before sending an update request.
- PATCH makes it easier to keep a track of update intent to see what changed with each request.
- PATCH saves bandwidth by allowing partial updates.
Overall, PATCH is more efficient than PUT for updating large objects. Moreover, PATCH can be useful in situations where we need to review logs to debug. Reproducing how resource change is easier with PATCH rather than PUT because the request body has a very specific intent.
Generic Updates vs Intent
It's important to consider both client and server implementation when choosing between PATCH and PUT.
From the client's perspective, PATCH seems great because it only updates the data that you need to. But when you look at it from a server's perspective, things get complicated.
For example:
PATCH /orders/533
{
"total": 8.34
}
In this request, we only want to update the total
, meaning that server implementation should not touch other fields. However, server developers now need to know all fields that clients might update.
We call this the "intent" of the update and it's not explicit in a PATCH request.
To overcome this, we can use a feature called JSON Patch, where we define the intent of the update.
An intent represents a change you want to make of an entity. With property-based patching, you can use HTTP headers to express the intent of an update.
PATCH /orders/533
[
{ "op": "replace", "path": "/total", "value": "8.34" }
]
According to RFC6902, the supported intents are "add", "remove", "replace", "move", "copy", or "test".
JSON Patch makes updating a resource a lot easier. The client defines the intent, and the server updates the object based on the context of that definition.
Idempotent Principle
RESTful architecture is all about idempotent operations. An idempotent operation has the same result, no matter when it is done. Non-idempotent operations can have different results.
An idempotent method's definition applies to the resource rather than the result. If you call the same idempotent method twice, the method should produce the same result both times.
An idempotent method should not have any side effects. A side-effect is any state change outside the method itself.
PUT is idempotent but PATCH is not because of its ability to modify the resource's entire content rather than specific fields.
PATCH is not idempotent because the modified data might result in a different state than if it had never been patched.
Concurrent updates
When applying PATCH changes, it's a good idea to include a condition in the request to prevent it from succeeding if someone else updated the resource in the meantime.
The ETag HTTP response header is an ID. It is the identifier for a specific version of a resource.
When the If-Match header and ETags are used in combination, they help to make sure that when a client is trying to update a resource, they can't. Instead, the old resource is protected.
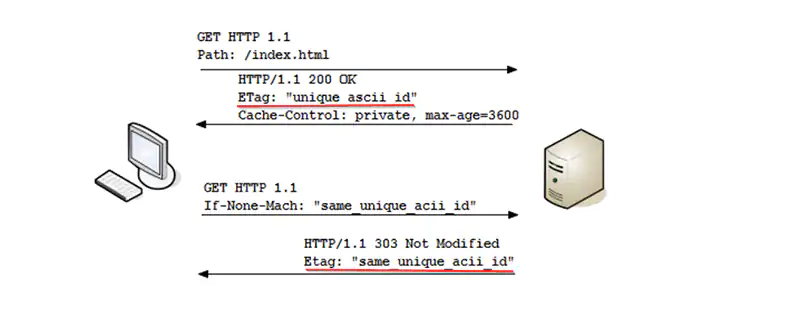
Summary - PATCH vs PUT
The Internet Engineering Task Force (IETF) is a group of people who work on how the World Wide Web should work. They have rules, but it is up to developers to follow them by adding them to their systems.
If you have to choose between PATCH and PUT, consider these main differences:
- With a PUT request, the server modifies the resource identified by the Request-URI in place. In contrast, with a PATCH request, the server replaces or updates only certain parts of a resource without changing other fields.
- PUT can create a resource, but PATCH cannot.
- PUT is idempotent, but PATCH is not.
- PUT uses more bandwidth than PATCH because it sends full request bodies.
If you want to learn more about REST APIs, take a look at my guide on Best REST API books.
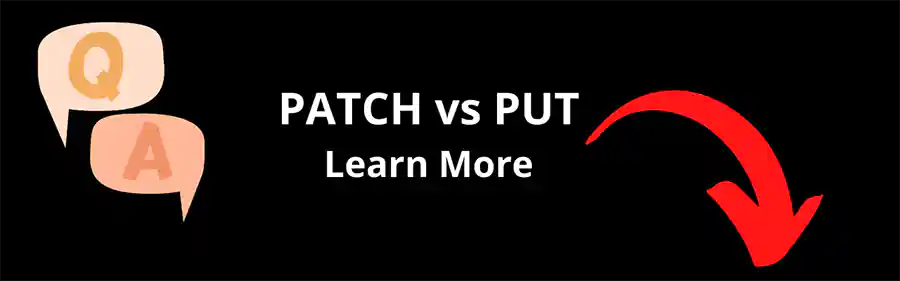
FAQ
What is the difference between PUT and PATCH?
The difference between PUT and PATCH in REST API is that PUT handles updates by replacing the entire entity, while PATCH only updates the fields that you give it.
Should I use PUT or PATCH?
If you have a simple application with small entities, using PUT is most likely easier because you can model your "create" and "update" functionality off of the same code. But if you are doing a lot of updates, for example in a dashboard feature, having a granular control with PATCH is better.
Do PATCH requests have a body?
Yes. PATCH requests have a body that comprises the instructions to modify or add data to an existing resource.
Which HTTP status code should PATCH return?
Successful PATCH requests should return a status code from the 2xx range. The two most common are 204 (No Content) if there is no response data and 200 (OK) if there is some response data.
Which HTTP status code should PUT return?
Successful PUT requests should return a status code from the 2xx range. If the intended resource does not yet have a current representation and the PUT request creates one, the origin server must send a 201 (Created) response to the client.
Can you use both PUT and PATCH?
Yes, you can design your APIs to support both PUT and PATCH since they have different purposes and use cases.
Is PATCH or PUT easier for the client?
It depends on the use case, but PATCH is usually easier for the client because it allows to make changes without reloading the whole resource.
Is PATCH allowed on read-only resources?
Yes, you can use a patch if the only thing you want to do is test something using the "test" intent. You don't need to change anything in the patch.
Why PUT is idempotent and PATCH is not?
PUT is idempotent because calling it once or several times produces the same effect. PATCH is not idempotent because the update intent might yield different results every time.
Can PATCH be idempotent?
Yes, it can.
For example, if you use PATCH to update an order's total with "set" : \ "price" : 50 \
, then successive patches with this field set to the same value will
produce the same result, thus the operation will be idempotent.
Does an idempotent method have side-effects?
No, an idempotent method should not have any side effects.
Is PUT safe?
PUT is not safe. An HTTP method is safe if it doesn't change the server's state. A method is safe if it leads to a read-only operation.
Can PATCH create a resource?
It may, according to RFC 5789, but in practice, it doesn't. That's different between PATCH and PUT because PUT creates a resource if it doesn't exist.
Read more: REST API Best Practices
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
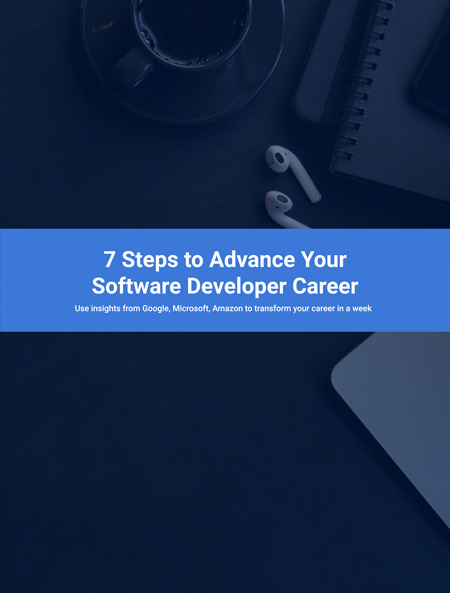
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.