C# Abstract Class vs Interface: Key Differences π
What's the difference: C# Abstract Class vs Interface?
Abstract class is a partially implemented base class, while interface declares a contract for methods without implementation.
Abstract classes suit types sharing implementation, whereas interfaces suit independent implementations. Abstract classes allow fields, constructors, and concrete methods, while interfaces don't. Classes can inherit one abstract class but multiple interfaces.
This table summarizes the differences between an abstract class and an interface:
Feature | Abstract Class | Interface |
---|---|---|
Inheritance | Single inheritance | Multiple inheritance |
Implementation | Partial implementation allowed | No implementation allowed |
Fields | Can have fields | Cannot have fields |
Constructors | Can have constructors | Cannot have constructors |
Methods | Can have concrete methods | Only method declarations |
Access Modifiers | Allowed for methods | No access modifiers |
Use case | Common implementation sharing | Independent implementation |
Type of abstraction | Achieves partial abstraction | Achieves complete abstraction |
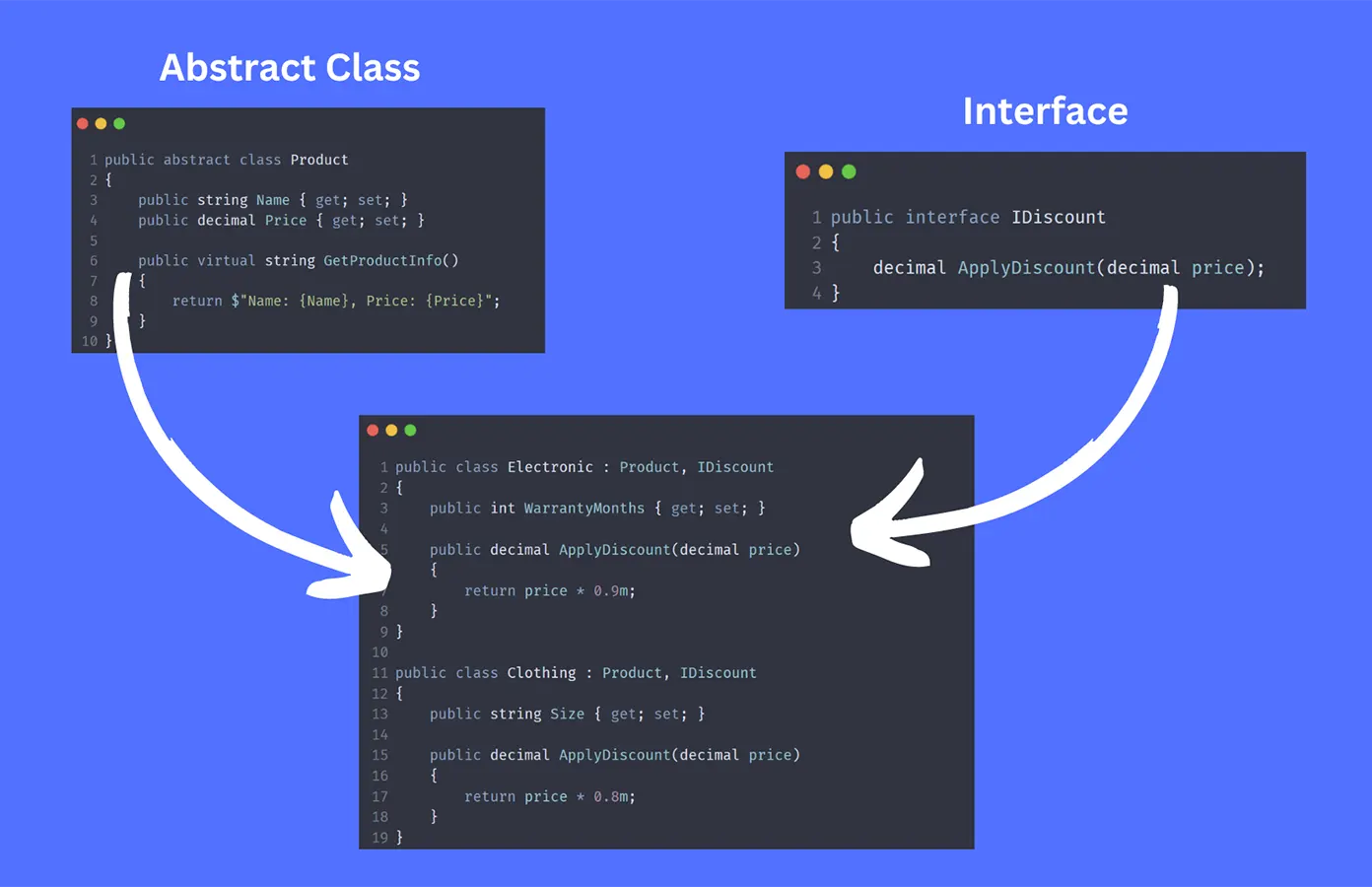
What is an abstract class?
An abstract class in C# is a superclass that serves as a base for other classes. It can have abstract members, which must be implemented by derived concrete subclasses.
Abstract classes can also have virtual members with a default implementation, allowing subclasses to override them if needed.
If a subclass doesn't implement all abstract members or is also declared abstract, it cannot be instantiated.
Abstract class:
- Base for other classes
- Contains abstract members
- Subclasses must implement abstract members
- Can have virtual members
- Subclasses can override virtual members
- Cannot be instantiated
- Subclass either implements all members or is also abstract
Abstract Class Example
public abstract class PaymentMethod
{
public abstract void ProcessPayment(decimal amount);
protected string FormatTransaction(decimal amount)
{
return $"Processed ${amount} payment.";
}
}
public class CreditCardPayment : PaymentMethod
{
public override void ProcessPayment(decimal amount)
{
// Process credit card payment
Console.WriteLine(FormatTransaction(amount));
}
}
public class PayPalPayment : PaymentMethod
{
public override void ProcessPayment(decimal amount)
{
// Process PayPal payment
Console.WriteLine(FormatTransaction(amount));
}
}
In this example, PaymentMethod
is an abstract class with an abstract method ProcessPayment
and a protected method FormatTransaction
. The derived classes CreditCardPayment
and PayPalPayment
both inherit from PaymentMethod
and provide their own implementation.
The FormatTransaction
method can be called by the derived classes, allowing them to share the functionality of formatting the transaction details. This promotes code reusability as the formatting logic is written only once in the abstract class and can be used by any derived class inheriting from it.
What is an interface?
An interface is a contract that defines method signatures. It doesn't contain implementation, instead, it acts as a blueprint that classes must implement.
We use interfaces to enforce a specific structure or behavior on classes that implement the interface.
We use interfaces for abstraction, multiple inheritance, and loose coupling.
Interface Example
public interface IPaymentProcessor
{
bool ValidatePaymentDetails(PaymentDetails paymentDetails);
void ProcessPayment(PaymentDetails paymentDetails);
}
public class CreditCardProcessor : IPaymentProcessor
{
public bool ValidatePaymentDetails(PaymentDetails paymentDetails)
{
// Validate credit card details
}
public void ProcessPayment(PaymentDetails paymentDetails)
{
// Process credit card payment
}
}
public class PayPalProcessor : IPaymentProcessor
{
public bool ValidatePaymentDetails(PaymentDetails paymentDetails)
{
// Validate PayPal details
}
public void ProcessPayment(PaymentDetails paymentDetails)
{
// Process PayPal payment
}
}
public class PaymentDetails
{
// Payment details properties
}
// Usage:
IPaymentProcessor paymentProcessor = new CreditCardProcessor();
paymentProcessor.ProcessPayment(paymentDetails);
The IPaymentProcessor
interface defines methods for payment processing. Classes CreditCardProcessor
and PayPalProcessor
implement this interface, providing their payment processing logic. By using the interface, we ensure consistency and flexibility, allowing easy switching or adding of payment methods.
When to use an abstract class?
Use abstract class when you want to provide a base implementation for methods while preserving polymorphism.
If you don't care about polymorphism, it doesn't make sense to use abstract classes. That's because the purpose of abstract classes is to be a superclass. Without having subclasses, that purpose is not fulfilled.
What is Polymorphism?
Polymorphism is a concept in object-oriented programming, where objects of different classes can be treated as objects of a common superclass. It enables a single function or method to handle various data types, providing flexibility and extensibility of code.
When to use an interface?
Use an interface when you want to multiple classes to implement the same contract.
Interfaces are beneficial in several scenarios:
- Promote reusability
- Encourage modular design: Interfaces help to create modular and loosely coupled code. Support multiple inheritances: Unlike abstract classes, a class can implement multiple interfaces.
- Enforce consistent behavior
- Facilitate testing: Using interfaces, you can create mock objects for testing purposes.
- Design patterns: Interfaces play a crucial role in design patterns, such as Factory, Decorator, Observer, and Dependency Injection.
When to use an abstract class instead of an interface?
Use an abstract class over an interface when you need to provide default implementations for methods.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts β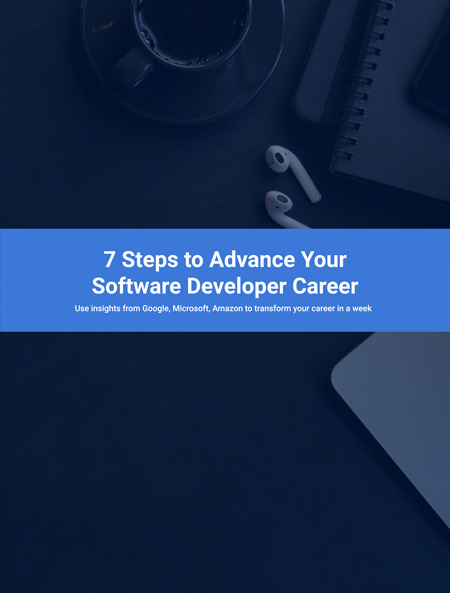
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.