How to add days to date in C#?
To add days to a date in C#:
- Get the current date using
DateTime.Now
- Call the
AddDays()
method to add the number of days you want to the date. - The returned value is a new DateTime object.
Here is the full example:
DateTime date = DateTime.Now;
date = date.AddDays(1); // Adds 1 days to the date
The AddDays method accepts a single parameter, which is the number of days to add to the date.
Remember to assign the date to the return value of AddDays because the date is immutable. That means that AddDays doesn't change the original date that we have, it just returns a new date that is 1 day later.
DateTime date = new DateTime(2022, month:2, day: 22);
date.AddDays(5);
Console.WriteLine(date); // 2/22/2022 12:00:00 AM
Month rollover
C# automatically rolls over dates when they go beyond the end of a month or year. For example, if you add 2 days to February 28th (the last day of the month), C# will automatically roll the date over to March 2nd.
DateTime date = new DateTime(2022, month:2, day: 28).AddDays(2);
Console.WriteLine(date); // 3/2/2022 12:00:00 AM
The same applies to years. If you add 365 days to January 1st (the first day of the year), C# will automatically roll the date over to January 1st of the next year:
DateTime date = new DateTime(2022, month:1, day: 1).AddDays(365);
Console.WriteLine(date); // 1/1/2023 12:00:00 AM
TimeSpan
Another way to add days to date is by using TimeSpan struct:
- Get the current date using
DateTime.Now
- Create a new TimeSpan object. The constructor takes in days, hours, minutes, seconds, and milliseconds.
- Use the
Add()
method to add the TimeSpan to the date.
DateTime date = new (2022, month:1, day: 1);
TimeSpan offset = new (days: 1, hours: 0, minutes: 0, seconds: 0, milliseconds: 0);
date = date.Add(offset);
Console.WriteLine(date); // 1/2/2022 12:00:00 AM
Using TimeSpan to add days is useful because it gives us more precision than just days. For example, if you want to add 1 day and 2 hours to a date, you can use the TimeSpan constructor that takes in hours:
DateTime date = new (2022, month:1, day: 1);
TimeSpan offset = new (days: 1, hours: 2, minutes: 0, seconds: 0, milliseconds: 0);
date = date.Add(offset);
Console.WriteLine(date); // 1/2/2022 2:00:00 AM
I prefer to use AddDays
because the syntax is cleaner and more concise, but both methods will achieve the same result.
FAQ
Is DateTime immutable in C#?
DateTime is immutable in C#. Immutable objects cannot be changed once they have been created. That means that the DateTime.Now property generates a new DateTime instance each time you call it.
Also, the AddDays method does not change the original date, it just returns a new date that results from adding days to the original date.
Because of immutability, we cannot simply do "day plus 1 day":
DateTime date = DateTime.Now;
date.Day += 1;
Error: (6,1): error CS0200: Property or indexer 'DateTime.Day' cannot be assigned to -- it is read only
Can I add negative numbers to a date?
If we add a negative number to the AddDays method, it will subtract that number of days from the date.
DateTime date = new (2022, month:1, day: 1);
date = date.AddDays(-5); // Subtracts 5 days from the date
Console.WriteLine(date); // 12/27/2021 12:00:00 AM
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →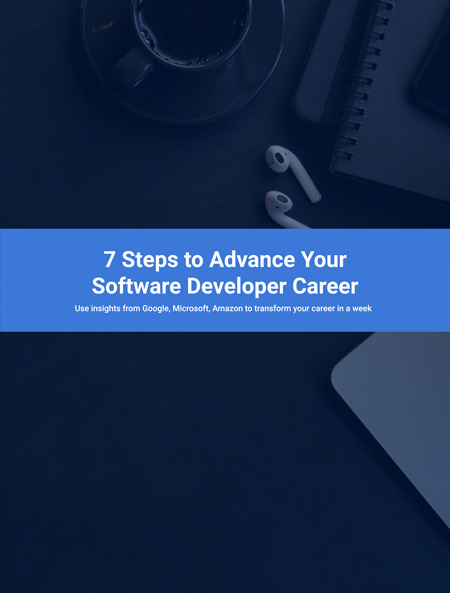
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.