How to add an item to a dictionary in C#?
Add() method
To add an item to a dictionary in C#:
- Check if item already exists in a dictionary using the
ContainsKey
method. - If the item doesn't exist, add it using the
Add
method. TheAdd
method takes in two arguments - key and value.
Dictionary<int, string> numbersToWords = new ();
if (!numbersToWords.ContainsKey(1))
numbersToWords.Add(1, "one");
Console.WriteLine(string.Join(',', numbersToWords)); // [1, one]
It's important to check if key already exists in a dictionary because C# cannot have duplicate keys.
If we try to add a key that already exists, we will get an exception:
Dictionary<int, string> numbersToWords = new ();
numbersToWords.Add(1, "one");
numbersToWords.Add(1, "one");
Console.WriteLine(string.Join(',', numbersToWords)); // [1, one]
Error: System.ArgumentException: An item with the same key has already been added. Key: 1 at System.Collections.Generic.Dictionary
2.TryInsert(TKey key, TValue value, InsertionBehavior behavior) at System.Collections.Generic.Dictionary
2.Add(TKey key, TValue value)
Indexer approach
To use an indexer to add an item to a dictionary:
- Use square brackets on the dictionary. The indexer value represents the key that we want to assign.
Dictionary<int, string> numbersToWords = new ();
numbersToWords[1] = "one";
numbersToWords[1] = "one";
Console.WriteLine(string.Join(',', numbersToWords)); // [1, one]
The indexer approach doesn't throw an exception if the key exists, instead it updates the mapped value:
Dictionary<string, int> wordOccupance = new ();
wordOccupance["water"] = 5;
Console.WriteLine(string.Join(',', wordOccupance)); // [water, 5]
wordOccupance["water"] = 6;
Console.WriteLine(string.Join(',', wordOccupance)); // [water, 6]
The indexer approach also lets you use arithmetic operators to change values:
wordOccupance["water"]++;
Console.WriteLine(string.Join(',', wordOccupance)); // [water, 7]
FAQ
What are indexers in C#?
Indexers are a way to access the elements in a class or struct that store a list or dictionary of values. Indexers work like properties, but you access them using an argument that is an index number instead of a property name.
For example, the indexer for Dictionary class has the following getter and setter:
object IDictionary.this[object key] {
get {
if( IsCompatibleKey(key)) {
int i = FindEntry((TKey)key);
if (i >= 0) {
return entries[i].value;
}
}
return null;
}
set {
if (key == null)
{
ThrowHelper.ThrowArgumentNullException(ExceptionArgument.key);
}
ThrowHelper.IfNullAndNullsAreIllegalThenThrow<TValue>(value, ExceptionArgument.value);
try {
TKey tempKey = (TKey)key;
try {
this[tempKey] = (TValue)value;
}
catch (InvalidCastException) {
ThrowHelper.ThrowWrongValueTypeArgumentException(value, typeof(TValue));
}
}
catch (InvalidCastException) {
ThrowHelper.ThrowWrongKeyTypeArgumentException(key, typeof(TKey));
}
}
}
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →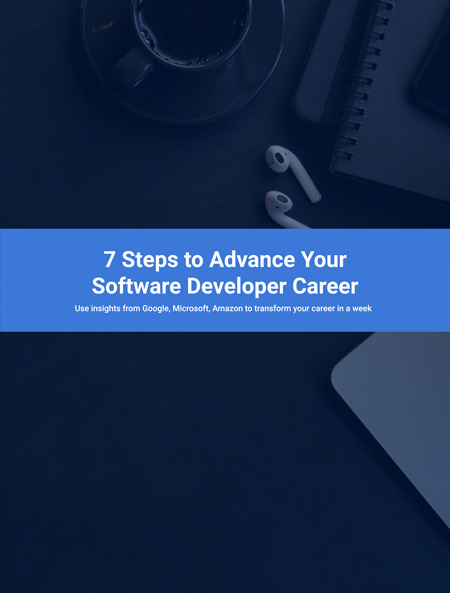
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.