C#: How to convert int to enum?
Cast int to enum
To convert into to enum in C#, use the cast operator.
The cast operator is pair of round brackets with the type that we want to convert to.
For example:
public enum Grade : int
{
A = 5,
B = 4,
C = 3,
D = 2,
F = 1,
}
Grade grade = (Grade)5;
Console.WriteLine(grade); // A
In the example above, we casted the integer 5 to Grade
enum A
.
Underlying types
We can cast (convert) int to enum because enum's underlying value is integer-based.
For example, if we try to use a decimal as an underlying type, we will get an error:
public enum Weight : decimal
{
Heavy = 1.0m,
}
Error: error CS1008: Type byte, sbyte, short, ushort, int, uint, long, or ulong expected
public enum Weight : byte
{
Heavy = 1,
}
To learn how to use string enums, read this article.
Type safety
We need to be careful when casting enums because enums don't have compile or runtime checks for supporting values. For example:
public enum Grade : int
{
A = 5,
B = 4,
C = 3,
D = 2,
F = 1,
}
Grade grade = (Grade)6;
Console.WriteLine(grade); // 6
In the code above, we don't get an error about the integer that we're casting being out of defined enum values.
To check if enum value is valid in C#, use on of the methods:
- Enum.IsDefined(Type enumType, object value)
Enum.IsDefined<TEnum>(TEnum value)
- Type.IsEnumDefined
Enum.IsDefined
is a static method. The method returns true if the integer value is in supported range:
public enum Grade : int
{
A = 5,
B = 4,
C = 3,
D = 2,
F = 1,
}
Console.WriteLine(Enum.IsDefined((Grade)6)); // False
Console.WriteLine(Enum.IsDefined((Grade)5)); // True
Console.WriteLine(Enum.IsDefined((Grade)5)); // True
Console.WriteLine(Enum.IsDefined((Grade)5)); // True
typeof(Grade).IsEnumDefined(5);
So, before we cast, we need to verify that enum is in supported range.
Extension method
Use this extension method to safely cast int to enums:
public static class ExtensionMethods
{
public static TEnum ToEnum<TEnum>(this int value)
{
if (typeof(TEnum).IsEnumDefined(value))
return (TEnum)(object)value;
return default;
}
}
Console.WriteLine(5.ToEnum<Grade>()); // A
Console.WriteLine(6.ToEnum<Grade>()); // 0
The extension method above safely converts int to enum. The method returns default value if enum doesn't have corresponding int value.
What is casting in C#?
Casting in C# is an operation that converts between data types. In C#, there are two types of casting: implicit and explicit. The main difference between implicit casting and explicit casting is that implicit casting is automatic, safe, and doesn't result in data loss. On the other hand, explicit casting is manual. For example, when we convert int to enum, we perform explicit casting and we tell the compiler that we accept the risks of two types not being compatible. To mitigate the risk, we can perform type checks, such as Enum.IsDefined
.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →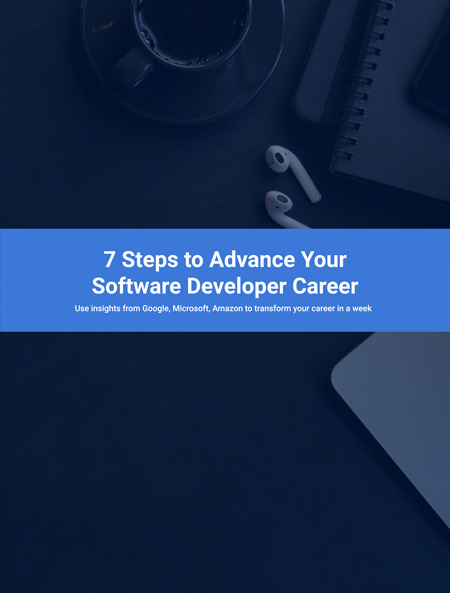
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.