C#: Checked vs Unchecked | Differences between Checked and Unchecked keyword in C#
C#: Checked vs Unchecked
The main difference between checked and unchecked is that the checked keyword enables overflow checking, while the unchecked keyword disables it. In checked context, an exception is thrown if the result is out of range, while unchecked truncates the result to fit the range.
Here's a code example to illustrate the difference:
int x = int.MaxValue;
int y = int.MaxValue;
int z1 = x + y; // throws OverflowException in checked context
int z2 = unchecked(x + y); // does not throw an exception
In the example above, the addition of x and y results in a value that is outside the range of the int type. In the checked context, this throws an OverflowException
exception. In the unchecked context, the result is truncated to fit within the range of int and no exception is thrown.
Here is a table that summarizes the difference between checked
and unchecked
keywords:
Keyword | Description |
---|---|
checked | Enables overflow checking. An exception is thrown at runtime if an arithmetic operation or conversion results in a value that is outside the range of the destination type. |
unchecked | Disables overflow checking. The result of an arithmetic operation or conversion is truncated to fit within the range of the destination type, and no exception is thrown. |
C#: Compile-time vs Runtime overflow
For constants, the compiler checks for value overflow during the compile time.
For example:
const int max = int.MaxValue * 2;
The code above will give you the error:
"The operation overflows at compile time in checked mode" - CS0220
Which operators are affected by the checked operator?
The checked operator affects expressions with the ++, −−, +, − (binary and unary), , *, /, and explicit conversion operators between integral types.
When should I use checked?
The checked context is used by default and it should be used for most scenarios.
By using the checked operator, your code will throw an error if an integral variable tries to exceed the maximum value, allowing you to catch the issue and prevent it from happening.
We rarely need to use the unchecked
operator.
When should I use unchecked?
You should use unchecked
when you are confident that the arithmetic will not result in an overflow or underflow, and you want to improve performance by avoiding the overhead of the checked context.
Is the default overflow checking context `checked` or `unchecked`?
The default overflow checking context is checked. By default, an exception is thrown at runtime if an arithmetic operation or conversion results in a value that is outside the range of the destination type.
Does the checked overflow context apply to string?
The checked overflow context doesn't apply to string length. Checked context is only supported for integral types.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
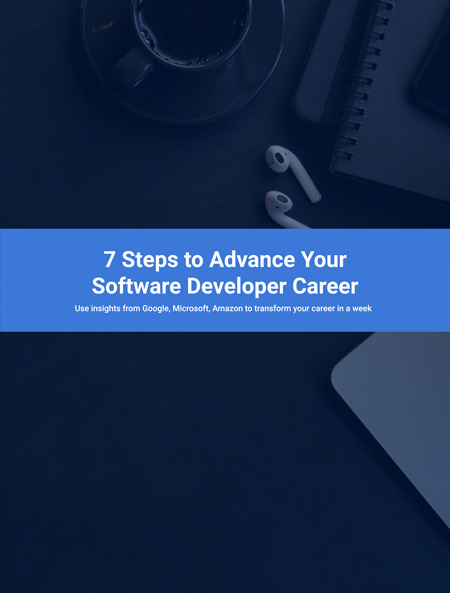
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.