C# Contains Ignore Case: Quick guide
The C# contains method allows developers to check if a string exists within another string.
But what if two strings are in different cases?
What if you want a method that works with both uppercase and lowercase letters?
So many times I've made a mistake of not making sure that both strings are in the same case when I've used contains before.
These mistakes are hard to catch because they don't cause exceptions or errors.
In this post, I will share my favorite way of ignoring case when checking if a string contains a substring.
Case insensitive contains
To perform case insensitive contains in C#, use the String.IndexOf method.
The String.IndexOf()
finds the first occurrence of a particular string inside another string. The comparison type is determined by the StringComparison parameter, which we pass as the second parameter.
- String.IndexOf() returns the position of the first occurrence of a substring inside a string.
- The first parameter is the instance of the String class you want to search for.
- The StringComparison.OrdinalIgnoreCase parameter tells the compiler to ignore the case when it is looking for an index.
Since String.IndexOf()
returns the zero-based index of the first occurrence, we can compare it with -1 to identify whether the string was found or not.
Extension method
If you do a lot of string comparisons, you can use this extension method to make the process a lot easier:
public static class StringExtensions
{
public static bool ContainsCaseInsensitive(this string source, string substring)
{
return source?.IndexOf(substring, StringComparison.OrdinalIgnoreCase) > -1;
}
}
For instance, here's how you could use this method:
string sentence = "Hello world";
Console.WriteLine(sentence.ContainsCaseInsensitive("hello")); //prints True
Console.WriteLine(sentence.ContainsCaseInsensitive("world")); //prints True
The extension method also makes sure that the source string is not null.
Using the extension method is my favorite way of checking strings for equality. It's simple and easy to read.
It also works with both uppercase and lowercase letters, so there is no need for multiple versions of the same code.
Don't use .Upper or .Lower
If you want to perform a case insensitive search, do not use the String.ToUpper or String.ToLower methods.
IndexOf
is much better approach because Upper
and Lower
return a new instance of the string class, which leads to unnecessary allocation of memory.
String.IndexOf()
returns the zero-based index of the first occurrence of substring
. It doesn't make a new copy.
If it succeeds, it returns an integer; if it fails to find the substring it returns -1 (this behavior can be
Alternative using Regex
Another option for case insensitive contains is to use regular expressions.
If you are comfortable with Regex, this approach will be faster than the IndexOf approach.
We can use the Regex.IsMatch
method, which returns a Boolean value.
To make sure that the matches are case insensitive, we can pass a RegexOptions.IgnoreCase as the second parameter to Regex.IsMatch
.
Regex.IsMatch("United States", Regex.Escape("states"), RegexOptions.IgnoreCase);
Note: Make sure you use Regex.Escape, otherwise the string will be considered a regular expression. This would lead to all kinds of unexpected results.
The only downside is that regular expressions might be new to some developers, so IndexOf is the simplest solution.
Normalize strings to uppercase
In case you need to normalize a string, always normalize to uppercase.
Converting strings to uppercase is important because it makes sure that all the characters are the same case. This is necessary for making sure that C# can accurately convert between different locales.
If you use lowercase letters, a small group of characters cannot be converted back.
Use String.ToUpper(CultureInfo.InvariantCulture)
to make sure that you normalize to uppercase.
Examples
using System;
using System.Text.RegularExpressions;
public class Program
{
public static void Main()
{
string numbers = "one, two, FIVE";
Console.WriteLine(numbers.Contains("five")); // False
Console.WriteLine(numbers.IndexOf("five")); // -1
Console.WriteLine(numbers.IndexOf("five", StringComparison.OrdinalIgnoreCase)); // 10
Console.WriteLine(numbers.IndexOf("five", StringComparison.OrdinalIgnoreCase)); // 10
Console.WriteLine(Regex.IsMatch(numbers, Regex.Escape("five"), RegexOptions.IgnoreCase)); // True
}
}
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →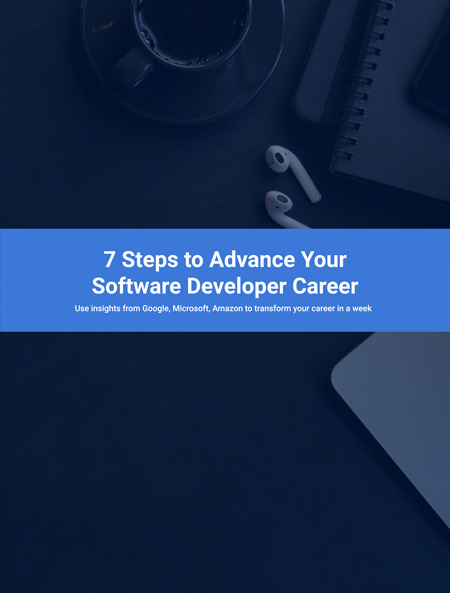
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.