C#: Controller vs ControllerBase
C#: Controller vs ControllerBase
The main difference between the Controller and ControllerBase classes in C# ASP.NET Core is that Controller is a higher-level class that inherits from ControllerBase. The Controller class includes additional functionality such as render views, JSON helpers, and action filters. On the other hand, the ControllerBase class provides the basic functionality for handling HTTP requests such as access to underlying HTTP context, request, response and URL.
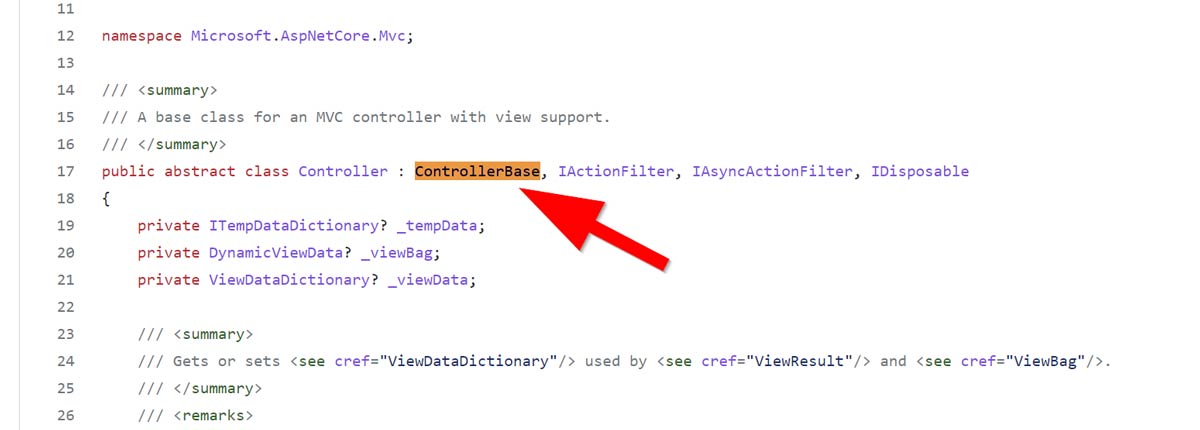
C# ASP.NET Core Controller class extends the functionality of the ControllerBase class.
ControllerBase
ControllerBase is a class in the C# ASP.NET Core framework that provides the basic functionality for handling HTTP requests such as access to the underlying HTTP context, request, response, routing information, and URL generation functionality. It does not include additional features such as View support and TempData, which are provided by the Controller class. It can be extended to include additional functionality if required, and it's a good choice when you want to create a custom controller class by inheriting from it.
Use ControllerBase when you are building Web APIs because they don't require view data.
Controller
The Controller class is a unified class created by ASP.NET Core, combining the Controller, ApiController, and AsyncController base classes from MVC5 and Web API 2.2.
The table below summarizes key features that Controller supports but ControllerBase doesn't.
Feature/Method | Description |
---|---|
ViewData | A dictionary-like object that allows you to pass data from the controller to the view. Data is stored in the ViewData property of the controller. |
View | Returns a ViewResult (derived from ActionResult) as the HTTP response. Defaults to a view of the same name as the action method, with the option of specifying a specific view. All options allow specifying a view model that is strongly typed and sent to the View. |
PartialView | Returns a PartialViewResult to the response pipeline. Partial views are reusable views that can be included in other views. |
Json | Returns a JsonResult containing an object serialized as JSON as the response. |
OnActionExecuting | Executes before an action method executes. This method can be overridden to perform additional tasks, such as logging, before the action method is executed. |
Controller vs ControllerBase Examples
Web API
Here is a code example of a simple web API controller using the ControllerBase class in C# ASP.NET Core:
[ApiController]
[Route("api/[controller]")]
public class MyApiController : ControllerBase
{
[HttpGet]
public IActionResult GetData(int id)
{
var data = new { id = id, name = "Your Name" };
return Ok(data);
}
[HttpPost]
public IActionResult AddData([FromBody] MyData model)
{
// Perform logic to add data
return CreatedAtAction(nameof(GetData), new { id = model.Id }, model);
}
}
Action Filters
Action filters are a great example of when to use Controller class over ControllerBase class.
In the example below, we use OnActionExecuting method to log the action name. OnActionExecuting is executed before the action method.
public class MyCustomController : Controller
{
public override void OnActionExecuting(ActionExecutingContext context)
{
var actionName = context.ActionDescriptor.DisplayName;
Console.WriteLine(actionName);
}
public IActionResult MyAction()
{
// Action logic here
return Ok();
}
}
When to use Controller versus ControllerBase?
Use a Controller when you need to handle web page requests and require additional functionality such as the ability to render views and generate HTML responses, pass data between actions, redirect the user to a different action and pass data from the controller to the view, and also access to action filters (OnActionExecuting, OnActionExecutionAsync, and OnActionExecuted methods).
On the other hand, use ControllerBase, when you are building Web APIs using core functionality such as HttpContext, Request, Response, RouteData, ModelState, and Url which can be used to access the underlying HTTP context, request, response, routing information, model binding and validation, and URL generation functionality respectively.
What is the inheritance relationship between Controller and ControllerBase classes?
The inheritance relationship between the Controller and ControllerBase classes in C# ASP.NET Core is that the Controller class inherits from the ControllerBase class. This means that the Controller class has access to all of the methods and properties of the ControllerBase class, but also includes additional features and functionality that are not present in the base class.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →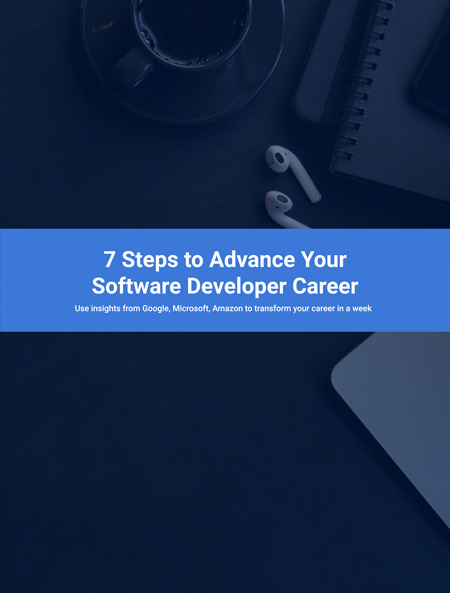
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.