C# Custom Exceptions: Complete Guide [2023]
- Create a custom exception in C#
- Custom Exceptions Best Practices
- What to avoid when creating custom exceptions in C#?
- Custom Exception Performance
- Custom Exception examples
- Custom exception class with custom properties
- Most important terms
- FAQ: Custom Exceptions
- When should I create a custom exception class?
- How do I throw a custom exception?
- How do I catch a custom exception?
- Can I add additional properties or methods to my custom exception class?
- What are the `base()` calls in custom exceptions?
Create a custom exception in C#
In C#, you can create custom exceptions by creating a new class that derives from the Exception
class. The custom exception class can also have additional properties or methods that you need.
Here is an example of how you could create a custom exception class in C#:
public class InvalidInputException : Exception
{
public InvalidInputException() : base() { }
public InvalidInputException(string message) : base(message) { }
public InvalidInputException(string message, Exception innerException) : base(message, innerException) { }
}
This custom exception class above represents a condition where the user has provided invalid input. The class derives from the built-in Exception
class and adds a few additional constructors to make it easier to create the exception.
Here is an example of how to throw and catch this custom exception:
try
{
// code that might throw an exception
if (inputIsInvalid)
{
throw new InvalidInputException("The input is invalid!");
}
}
catch (InvalidInputException ex)
{
// handle the exception
Console.WriteLine(ex.Message);
}
In this example, the try block contains code that might throw an InvalidInputException
if the input is invalid. If the exception is thrown, it is caught in the catch block and the error message is printed to the console.
Custom Exceptions Best Practices
Here are a few things to keep in mind when creating custom exceptions in C#:
-
Create a separate exception class for each error condition that you want to handle. This makes it easier to identify and handle specific exceptions in your code.
-
Override the
Message
property to provide a meaningful error message that describes the error condition. This message will be displayed when the exception is thrown. -
Override the
ToString()
method to return a string representation of the exception, which can be useful for logging and debugging purposes. -
Add additional properties or methods that you need to your custom exception class to provide more information about the error condition.
What to avoid when creating custom exceptions in C#?
Here are a few things to avoid when working with custom exceptions in C#:
-
Avoid creating too many custom exception classes. It is a good idea to create a separate exception class for each error condition that you want to handle, but you should try to keep the number of exception classes to a minimum. Having too many exception classes can make your code more difficult to understand and maintain.
-
Avoid throwing exceptions for expected error conditions. Exceptions are intended to handle unexpected error conditions, not to control the normal flow of your code. If you have an error condition that you expect to occur under certain circumstances, it is better to handle it using normal control flow statements like
if
andelse
rather than throwing an exception. -
Avoid catching and ignoring exceptions. If you catch an exception, you should take some action to handle the error condition. Ignoring exceptions can cause problems in your code and make it difficult to track down and fix bugs.
-
Avoid catching the base Exception class. The Exception class is the base class for all exceptions in C#, and catching this class will catch all exceptions, including those that you might not be prepared to handle. Instead, you should catch specific exception types that you are prepared to handle.
Custom Exception Performance
Using custom exceptions in C# should not have a significant impact on the performance of your code. Throwing and catching exceptions can be somewhat slower than normal control flow statements like if and else, but the difference is usually small and should not be a concern unless you are writing code that needs to be extremely high performance.
In general, the benefits of using custom exceptions far outweigh any potential performance issues. Custom exceptions can help you write more organized and maintainable code by providing a clear and concise way to represent and handle error conditions.
Custom Exception examples
Custom exception class with custom properties
Here is an example of a custom exception class that includes custom properties:
public class InvalidInputException : Exception
{
public string InvalidInput { get; }
public InvalidInputException(string message, string invalidInput) : base(message)
{
InvalidInput = invalidInput;
}
}
This custom exception class represents an error condition where the user has provided invalid input. The class includes a custom property called InvalidInput
that stores the invalid input that caused the exception.
Here is an example of how you could throw and catch this custom exception:
try
{
// code that might throw an exception
if (inputIsInvalid)
{
throw new InvalidInputException("The input is invalid!", invalidInput);
}
}
catch (InvalidInputException ex)
{
// handle the exception
Console.WriteLine(ex.Message);
Console.WriteLine("Invalid input: " + ex.InvalidInput);
}
In this example, the try
block contains code that might throw an InvalidInputException
if the input is invalid. If the exception is thrown, it is caught in the catch
block and the error message and invalid input are printed to the console.
Most important terms
Here is a table of some important terms related to custom exceptions in C#:
Term | Definition |
---|---|
Exception | The base class for all exceptions in C#. The |
Throw | The |
Catch | The |
Try-catch block | A |
Custom exception | A custom exception is a class that derives from the
|
Finally block | The |
FAQ: Custom Exceptions
Here are a few frequently asked questions about custom exceptions in C#:
When should I create a custom exception class?
It is generally a good idea to create a custom exception class when you want to represent a specific error condition that is not covered by the built-in exception classes in C#. For example, if you are working on a financial application, you might want to create a custom exception class to represent an error condition where the user has insufficient funds in their account.
How do I throw a custom exception?
To throw a custom exception, you can create an instance of your custom exception class and use the throw
keyword to throw it. For example:
throw new InvalidInputException("The input is invalid!");
How do I catch a custom exception?
To catch a custom exception, you can use a catch block with a type parameter that matches the type of your custom exception class. For example:
try
{
// code that might throw an exception
}
catch (InvalidInputException ex)
{
// handle the exception
}
Can I add additional properties or methods to my custom exception class?
Yes, you can add any additional properties or methods that you need to your custom exception class. This can be useful if you want to provide more information about the error condition. For example, you might want to add a property to store the invalid input that caused the exception, or a method to log the exception to a file.
What are the `base()` calls in custom exceptions?
In C#, the base
keyword is used to access the base class of a derived class. When creating a custom exception class that derives from the built-in Exception
class, you can use the base
keyword to call the constructors of the base class.
For example, consider the following custom exception class:
public class InvalidInputException : Exception
{
public InvalidInputException() : base() { }
public InvalidInputException(string message) : base(message) { }
public InvalidInputException(string message, Exception innerException) : base(message, innerException) { }
}
This custom exception class has three constructors, each of which calls the corresponding constructor of the Exception
class using the base
keyword.
The first constructor calls the default constructor of the Exception
class, the second constructor calls the Exception
constructor that takes a message parameter, and the third constructor calls the Exception
constructor that takes a message and inner exception parameter.
The base()
calls in the custom exception class allow the class to inherit the behavior and functionality of the Exception
class, while also adding any additional properties or methods that are specific to the custom exception class.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →- Create a custom exception in C#
- Custom Exceptions Best Practices
- What to avoid when creating custom exceptions in C#?
- Custom Exception Performance
- Custom Exception examples
- Custom exception class with custom properties
- Most important terms
- FAQ: Custom Exceptions
- When should I create a custom exception class?
- How do I throw a custom exception?
- How do I catch a custom exception?
- Can I add additional properties or methods to my custom exception class?
- What are the `base()` calls in custom exceptions?
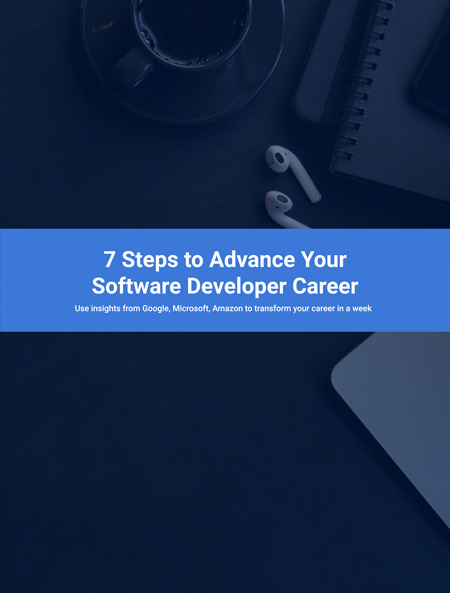
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.