C#: Generate a random number
How to generate a random number in C#?
To generate a random number in C#:
- Instantiate the
Random
class. - Call
Next(n)
to generate a random integer between 0 and n-1. - Capture the returned random integer.
Here's the full code to generate a random number in C#:
var random = new Random();
int myRandomNumber = random.Next(5);
Console.WriteLine(myRandomNumber); // 3
How to generate a random number in range?
To generate a random number in range from X to Y:
- Instantiate the
Random
class. - Call
Next(X, Y)
to generate a random integer between X and Y. - Capture the returned random integer.
Here's the full code:
var random = new Random();
int myRandomNumber = random.Next(5, 10);
Console.WriteLine(myRandomNumber); // 6
How to generate cryptographically strong random values?
The Random class doesn't create strong random values.
To create strong random values, use the static GetInt32
method from the System.Security.Cryptography.RandomNumberGenerator class:
int randomNumber = System.Security.Cryptography.RandomNumberGenerator.GetInt32(5, 15);
Console.WriteLine(randomNumber); // 8
The GetInt32 method has two overloads:
Overload | Use |
---|---|
GetInt32(Int32) | Generates a random integer between 0 (inclusive) and a specified exclusive upper bound sing a cryptographically strong random number generator. |
GetInt32(Int32, Int32) | Generates a random integer between a specified inclusive lower bound and a specified exclusive upper bound using a cryptographically strong random number generator. |
Best practices for generating random numbers
Single instance of the Random class
Always try to reuse the single instance of the Random class because:
- Initializing a Random class is expensive for performance,
- Two Random instances created withing couple of milliseconds yield the same sequence of values. This behavior is often not desired.
Thread safety
The System.Random class is not thread safe, so use the locking mechanism if you plan to generate random numbers in multithreaded environment.
FAQ
How are random numbers generated?
By default, the .NET framework generates random numbers based on the current system time.
Numbers generated using the Random class are not considered reliable for high-stake scenarios such as cryptography because the system clock has limited granularity. For example, two Random instances created withing couple of milliseconds yield the same sequence of values.
Watch this video if you want to learn more on how random numbers are generated in C#:
What is the Seed value?
The Random class has a constructor that accepts the seed value. Seed is a a starting value for the pseudo-random number generation algorithm.
If we use the same seed for different Random objects, they will generate the same series of random numbers. This functionality is useful when we want reproducibility.
var random = new Random(3);
int myRandomNumber = random.Next(3);
var random2 = new Random(3);
int myRandomNumber2 = random2.Next(3);
Console.WriteLine(myRandomNumber); // 0
Console.WriteLine(myRandomNumber2); // 0
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →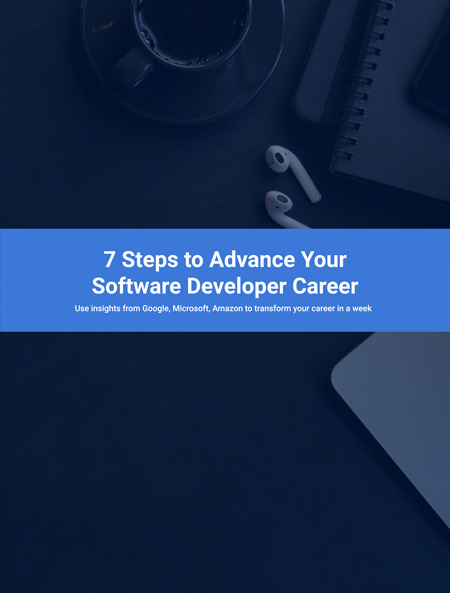
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.