Difference between IEnumerable and List
IEnumerable vs List
The main difference between IEnumerable and List in C# is that IEnumerable is an interface, while List is a concrete class. Moreover, IEnumerable is read-only and List is not. List represents the entire collection in memory, while IEnumerable provides an interface for getting the next item one-by-one (enumerating data).
Both IEnumerable and List are part of .NET's System.Collections namespace.
Deferred execution
Difference between IEnumerable and List is clear when working with LINQ.
IEnumerable is a deferred execution while List is an immediate execution.
IEnumerable will not execute the query until you enumerate over the data, whereas List will execute the query as soon as it's called. Deferred execution makes IEnumerable faster because it only gets the data when needed.
In the code example below, we use LINQ to query the list of numbers. Even though the LINQ Where
returns an IEnumerable, the execution is deferred until we call ToList()
. When you use ToList()
, you force the compiler to reify the results right away.
using System.Linq;
List<int> numbers = new() { 1, 2, 3, 4, 5};
IEnumerable<int> greaterThanTwo = numbers.Where(number => number > 2); // The query is not executed yet!
numbers.Add(6);
List<int> result = greaterThanTwo.ToList(); // Enumerates now.
Console.WriteLine(string.Join(',', result)); // 3,4,5,6
IEnumerable is more efficient and faster when you only need to enumerate the data once.
The List is more efficient when you need to enumerate the data multiple times because it already has all of it in memory.
Underlying interfaces and implementation
The IEnumerator interface defines how the compiler can access elements in a collection one at a time (enumerate). IEnumerable uses IEnumerator internally and is the recommended way to enumerate the contents of a collection.
On the other hand, List uses IList, a non-generic interface, internally. IList allows for more direct manipulation of the contents of a list and is the recommended way to manipulate a list.
The difference between IEnumerable and IList is that IEnumerable only provides a way to enumerate the contents of a list, while IList provides a way to manipulate the list itself.
IEnumerable
IEnumerable is an interface that allows us to write foreach semantics. All classes that implement this interface support foreach semantics.
List
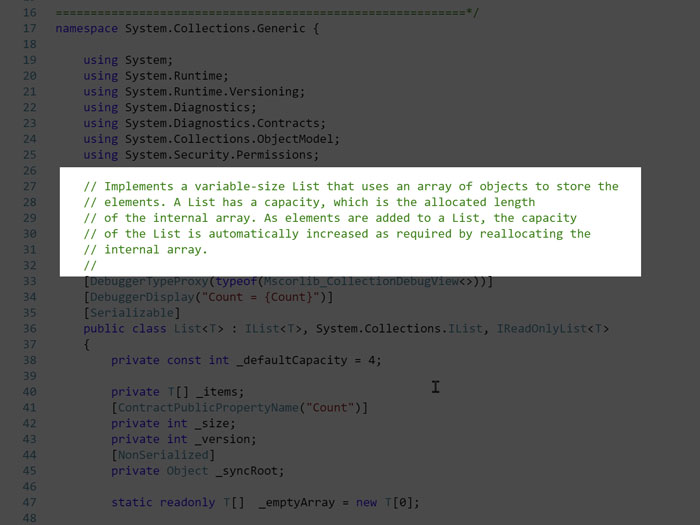
Lists store objects in an internal array. When the internal array gets full, the ArrayList will automatically create a new, larger, internal array and copy all the objects from the old array to the new one. Adding elements to a List is fast because there is usually a free slot at the end of the internal array.
The IList interface provides methods for manipulating lists. The most notable functionalities are:
- Add: Adds an object to the end of the list.
- Clear: Removes all objects from the list.
- Contains: Determines whether the list contains a specific object.
- IndexOf: Returns the index of the first occurrence of an object in the list.
- RemoveAt: Removes an object at a specific index from the list.
- Count: Read-only property describing how many elements are in the List.
FAQ
Should I use List or IEnumerable when designing methods?
Use IEnumerable if I only need basic functionalities over a collection like iterating. Use IList, ICollection if I need more specific operations over a collection.
When designing methods in C#, it's better to use interfaces rather than concrete implementations because it gives the most flexibility. For example, if our method takes in an IEnumerable as a parameter, then a caller can pass in a List, Array, Dictionary, or any collection that implements IEnumerable.
Is IEnumerable faster than List?
IEnumerable is conceptually faster than List because of the deferred execution. Deferred execution makes IEnumerable faster because it only gets the data when needed. Contrary to Lists having the data in-memory all the time.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →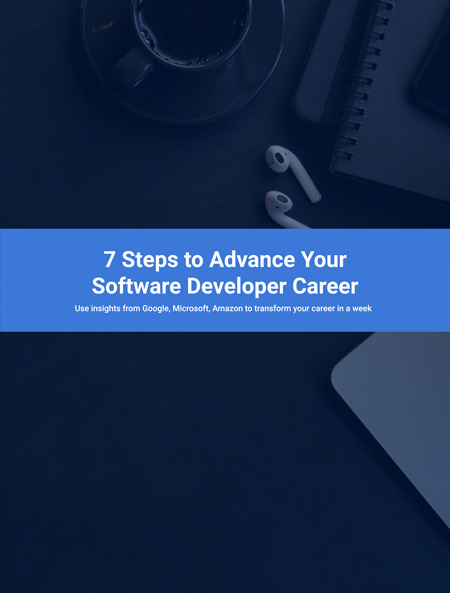
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.