C#: Difference between IsNullOrEmpty and IsNullOrWhiteSpace
IsNullOrEmpty vs IsNullOrWhiteSpace
The main difference between IsNullOrEmpty and IsNullOrWhiteSpace in C# is that IsNullOrEmpty checks if there's at least one character, while IsNullOrWhiteSpace checks every character until it finds a non-white-space character.
Moreover, IsNullOrEmpty was introduced in the .NET Framework version 2.0, while IsNullOrWhiteSpace was introduced in .Net Framework 4.0.
White space is any character that is not visible on screen. For example, space, line break, or an empty string.
IsNullOrEmpty vs IsNullOrWhiteSpace examples
The following examples show difference between IsNullOrEmpty and IsNullOrWhiteSpace:
string name = ".NET";
Console.WriteLine(string.IsNullOrEmpty(name)); // False
Console.WriteLine(string.IsNullOrWhiteSpace(name)); // False
Console.WriteLine(string.IsNullOrEmpty(string.Empty)); // True
Console.WriteLine(string.IsNullOrWhiteSpace(string.Empty)); // True
Console.WriteLine(string.IsNullOrEmpty(null)); // True
Console.WriteLine(string.IsNullOrWhiteSpace(null)); // True
Console.WriteLine(string.IsNullOrEmpty(" ")); // False
Console.WriteLine(string.IsNullOrWhiteSpace(" ")); // True
Console.WriteLine(string.IsNullOrEmpty("\n")); // False
Console.WriteLine(string.IsNullOrWhiteSpace("\n")); // True
IsNullOrEmpty vs IsNullOrWhiteSpace similarities
IsNullOrEmpty and IsNullOrWhiteSpace have the following simialrities:
- They return a boolean.
- Both are static methods.
- Both are pure methods. Pure methods don't not make any changes to state.
Why do we need need to do a null check?
In .NET, strings are a nullable value type.
C# Nullable value types is a type representation that allows a variable to contain either a value or null.
If we try to access a property on a string that's null
, we'll get a null-reference exception and our code will break.
Tony Hoare introduced Null references in ALGOL W back in 1965. Since then it crashed so many applications. He even calls null-reference to be a billion dollar mistake.
In C#, we use IsNullOrEmpty and IsNullOrWhiteSpace to protect our code against null-reference exceptions.
IsNullOrEmpty implementation
The implementation of IsNullOrEmpty in .NET:
public static bool IsNullOrEmpty(String value)
{
return (value == null || value.Length == 0);
}
IsNullOrEmpty returns true
if either value is null
or if the lenght of the string is zero.
IsNullOrWhiteSpace implementation
The implementation of IsNullOrWhiteSpace in .NET:
public static bool IsNullOrWhiteSpace(String value) {
if (value == null) return true;
for(int i = 0; i < value.Length; i++)
{
if(!Char.IsWhiteSpace(value[i])) return false;
}
return true;
}
IsNullOrEmpty returns true
if either value is null
or if all characters of the string are white space.
The method uses a for
loop through each character and performs the Char.IsWhiteSpace
check.
Which characters are considered white spaces?
In .NET, these characters are consider white spaces:
- U+0009 = Horizontal tab
- U+000a = Line feed
- U+000b = Vertical tab
- U+000c = Form feed
- U+000d = Carriage return
- U+0085 = Next line
- U+00a0 = No-break space
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →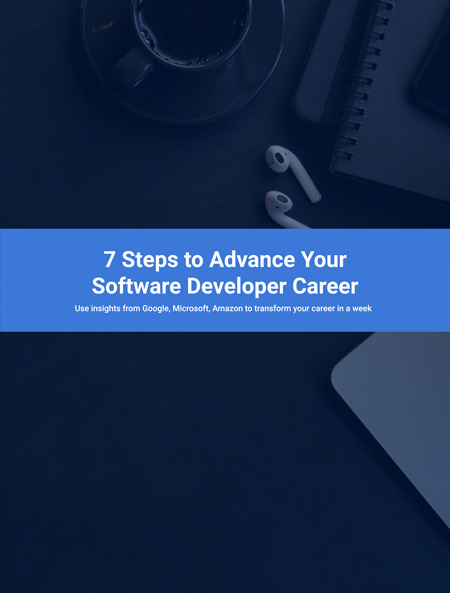
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.