C#: Difference between Dynamic and Object
Dynamic vs Object
The main difference between Object type and dynamic type in C# is that Object type is statically typed, while dynamic variables are dynamically typed. Moreover, for Object, the C# compiler checks types during compile-time, but for dynamic, it checks types during runtime.
Object class is the root of all classes, so it's used everywhere. On the other hand, we use the dynamic type rarely. We mostly use it to make interaction with other dynamic languages easier.
Since Object type is strongly-typed, Visual Studio IntelliSense provides suggestions as we type, but for dynamic type, the IntelliSense doesn't exist.
Dynamic type is prone to errors because type-checking happens at runtime.
Because of that, I never use it. I suggest you avoid it, as well.
Dynamic | Object |
---|---|
Dynamically typed. | Statically typed. |
Type checked on runtime. | Type checked at compile-time. |
Reassigned value can be of any type, regardless of initially assigned value. | Reassigned value must be of the same time as initially assigned value. |
Cannot use lambda expressions. | Support lambda expressions. |
Prone to runtime errors due to runtime type-checking. | Avoid runtime errors due to compile-time type-checking. |
No IntelliSense. | IntelliSense is available. |
What is dynamic type?
Dynamic is a type that Microsoft introduced in C# 4.0 in 2010.
When we use the dynamic
keyword, we can reassign any value to our variable. That value doesn't have to be of the same type. It can be different every time:
dynamic bag = "Cool";
Console.WriteLine($"{bag},{bag.GetType()}"); // Cool,System.String
bag = 10;
Console.WriteLine($"{bag},{bag.GetType()}"); // 10,System.Int32
bag = 0.5;
Console.WriteLine($"{bag},{bag.GetType()}"); // 0.5,System.Double
The assigned type doesn't even have to be a reference type, it can even be a value type (struct):
dynamic date = DateTime.Now;
date = 5;
date = "January";
Console.WriteLine(date); // January
C# is a statically typed language that uses a compiler to give us robust type-checking. But when we use the dynamic type, we briefly break away from C#'s strongly-typed nature.
What is an Object?
In C#, an Object is a class. It's the ultimate superclass that's a root of all other classes in the .NET Framework.
Since Object is on top of the hierarchy, it means we can cast any reference type as Object:
int myNumber = 5;
Object myObject = myNumber;
Console.WriteLine(myNumber); // 5
Console.WriteLine(myObject); // 5
When to use the Object type?
Use the Object type when you want to support more than one value type.
For example, when you a design method that takes in different value types.
In the example below, we want to get the number of engagements for a social media post.
Since we have Instagram and Facebook posts, our GetEngagementCount
method takes in a parameter of Object type. Using the is
keyword, we cast our Object class to a specific value-type:
public class InstagramPost
{
public int LikesCount { get; set; }
}
public class FacebookPost
{
public int ReactionsCount { get; set; }
}
int GetEngagementCount(Object entity)
{
if (entity is InstagramPost instagram)
return instagram.LikesCount;
else if (entity is FacebookPost facebook)
return facebook.ReactionsCount;
return default;
}
How does Object class impact performance?
Using Object class rather than a specific value-type impacts performance because it requires boxing and unboxing. Boxing is a process of converting a value type to an interface that this value type implements.
The performance impact of boxing and unboxing is low for most scenarios. But, if performance is your concern, it's always better to use specific value types.
When should I use dynamic in C#?
The dynamic type seems like a great thing because you can assign and reassign different types to the same variable. But that's not the case.
The dynamic keyword is usually a code smell because C# is a statically typed language that uses a compiler to give us robust type checking. But when we use the dynamic type, we break away from C#'s strongly-typed nature.
So, use cases for the dynamic keyword are very rare, they include:
- Dealing with unpredictable JSON bodies.
- When working with native code and assemblies.
- To simplify code that has a lot of reflections.
I never use it.
When should I not use dynamic in C#?
You shouldn't use dynamics when:
- You are too lazy to create a proper type.
- You suspect that runtime type-checking will cause errors.
- You can use generics instead.
What is Dynamic Language Runtime (DLR)?
The dynamic language runtime (DLR) is a runtime environment that adds support for dynamic languages to .NET.
The purpose of the dynamic runtime is to discover types at runtime, without compile-time checks.
This powerful feature lets us interact with other programming languages such as Python, Ruby, VB.NET.
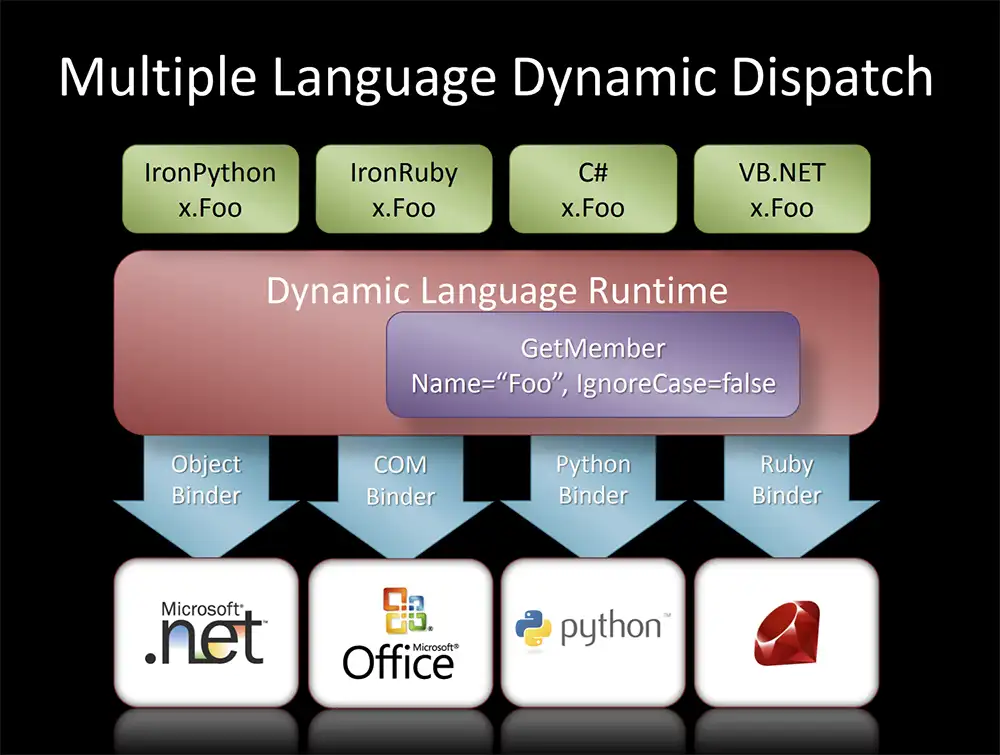
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
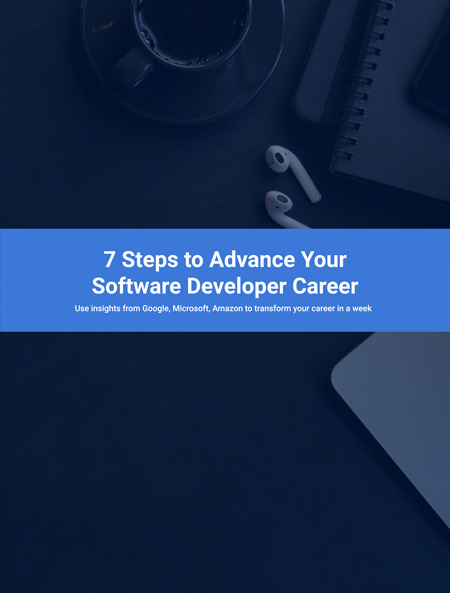
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.