C# Optional Parameters: Everything You Need to Know
Named and optional parameters are one of my favorite features of C#. I use them all the time.
In this blog post, we will discuss what optional arguments are and how to use them. We will also provide some examples to help you understand how they work.
What are C# optional parameters?
C# optional parameters are a way to specify the parameters that are required and those that are optional.
If we do not pass the optional argument value at calling time, it uses the default value.
Inside the method definition, we define a default value for the optional argument.
The compiler won't complain if we don't pass arguments for the optional parameters. The compiler knows to use the default value for any omitted parameters.
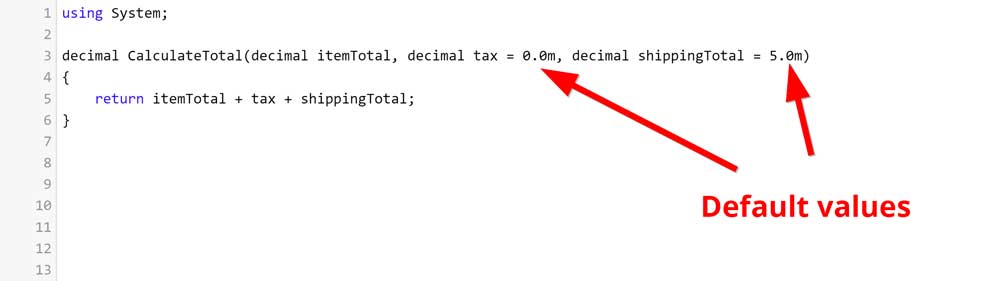
Named parameters and optional parameters
Named parameters and optional parameters go hand in hand.
Named arguments let you leave out optional arguments in a method call if you pass the remaining arguments by name.
You can put named arguments in any order, but only after you've listed all the required arguments. That's why required arguments are also called fixed arguments. They come at the end of the parameter list.
For example, when we have two optional parameters, we can change their order:
using System;
decimal test = 5.0m;
decimal CalculateTotal(decimal itemTotal, decimal tax = test, decimal shippingTotal = default) // optional arguments
{
return itemTotal + tax + shippingTotal;
}
Console.WriteLine(CalculateTotal(10.50m)); // 10.50 - first parameter is not optional
Console.WriteLine(CalculateTotal(10.50m, tax: 5.0m)); // 15.50
Console.WriteLine(CalculateTotal(itemTotal: 10.50m, tax: 5.0m)); // 15.50
Console.WriteLine(CalculateTotal(itemTotal: 10.50m, tax: 5.0m, shippingTotal: 1.0m)); // 16.50
Console.WriteLine(CalculateTotal(itemTotal: 10.50m, shippingTotal: 12.0m, tax: 5.0m)); // 27.50
The default values assigned in the following snippet to shippingTotal
and tax
are 0.
How to spot optional parameters?
IntelliSense uses brackets to show optional parameters. The method definition lists all the parameters, but you can pass arguments in any order if you used named arguments approach.

IntelliSense shows that optional parameters were omitted.
Default values restrictions
A default value of an argument is restricted to:
- a constant expression;
- a
new
expression of a value type, such as an string enum or a struct; - a
default
expression of a value type;
If you try to assign a variable to the optional argument, you will get a compile time error:
Default parameter value for must be a compile-time constant.
Advantages
The biggest advantage of using optional arguments with named parameters is that it makes your code more readable.
It's self-documenting.
When you see a method call, you can immediately tell which arguments are required and which ones are not.
using System;
// static void main
decimal CalculateTotal(decimal itemTotal, bool addTax = false)
{
decimal tax = 10.0m; // Call the tax service.
return addTax ? itemTotal + tax : itemTotal;
}
Console.WriteLine(CalculateTotal(10.0m, false));
Console.WriteLine(CalculateTotal(10.0m, addTax: false)); // Using named parameter is much better.
In this code snippet, if we omit arguments, it is difficult to understand what false
means.
Disadvantages
A major disadvantage to using named arguments is that the method caller needs to know the implementation details of a method. If we change the parameter name in the called method, we also need to change the client method.
We run into a risk of violating the programming principle of Method Hiding, which states that the implementation of a method should be hidden from its clients.
Conclusion
Named and optional parameters are a great way to make your C# code more readable. They allow you to omit arguments when calling a method, and the compiler will substitute the default for any omitted arguments. You can provide a default for an optional parameter by specifying it in the method declaration.
However, be aware of the disadvantages to using named arguments before deciding whether or not to use them in your own code.
Optional argument value has to be a compile-time constant. It needs to be specified at the end of the parameter list.
Omitting an optional argument value on the calling side results in a call to the default parameter value.
When using overloads instead of optional parameters, make sure that you hide the implementation details from your clients.
Otherwise, they may not be able to call your overloaded methods correctly.
FAQ
We've answered some of the most common questions about optional parameters below. If you have a question, that's not answered here, feel free to ask us in the comments.
When were optional parameters introduced?
Optional Parameters were introduced in C# 4.0 release in 2010.
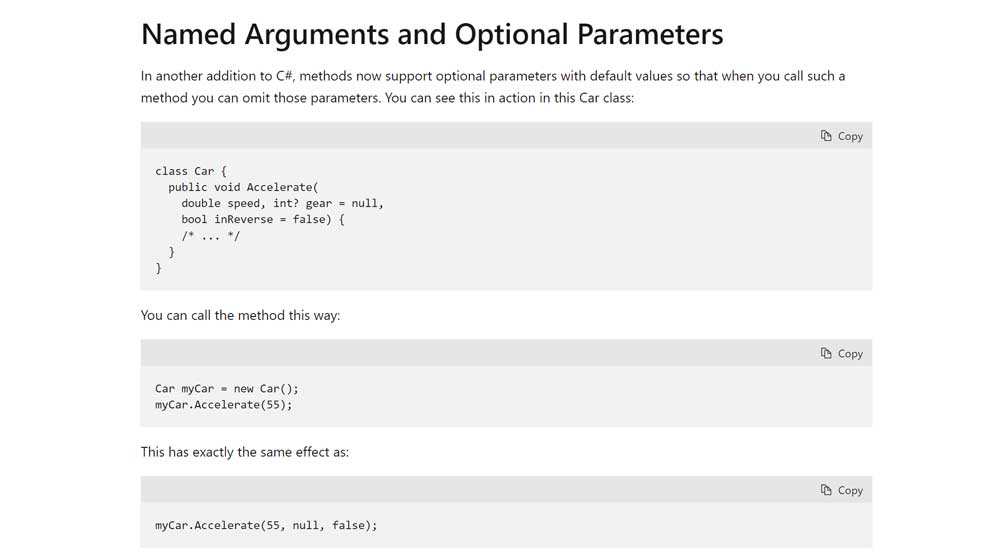
Screenshot from when Microsoft announced optional parameters in C# 4.0.
How does the compiler know which parameter is optional?
The compiler substitutes the default value of an optional parameter on the calling side.
A parameter is optional if it specifies a default value in its declaration:
void Foo (int x = 23) { Console.WriteLine (x); }
If we just call:
Foo(); // 23
The compiler infuses the value 23 into the executable code at the calling side, by defaulting the argument to x. The following call to Foo is syntactically identical to:
Foo (23);
Should I use optional arguments or method overloading?
The main difference between optional arguments and overloads is that optional parameters use the same method body, but overloads use different method bodies.
The advantage of optional arguments is that they are more concise. However, overloads are more explicit about what the function does.
- Use optional parameters If your method body is small and concise.
- Use method overloading if your method body is large and varies based on different parameters.
What is the Optional attribute?
Optional attribute from the System.Runtime.InteropServices namespace is used to mark the parameter as optional.
public void Foo([Optional, DefaultParameterValue(null)]string myOptionalParameter1, [Optional, DefaultParameterValue(null)]string myOptionalParameter2)
{
}
Using the attribute was the way to go before C# 4.0, but now it's not used as much.
What's the difference between required parameters and optional parameters?
The main difference between required parameters and optional parameters is that mandatory parameters must be provided when the function is called, while optional parameters automatically supplies the value if it's not provided.
Required parameters also include:
- Reference parameters
- Output parameters
- Parameter arrays
C# includes only one type of optional parameter:
- Default value parameters
Code examples
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
- What are C# optional parameters?
- Named parameters and optional parameters
- How to spot optional parameters?
- Default values restrictions
- Advantages
- Disadvantages
- Conclusion
- FAQ
- When were optional parameters introduced?
- How does the compiler know which parameter is optional?
- Should I use optional arguments or method overloading?
- What is the Optional attribute?
- What's the difference between required parameters and optional parameters?
- Code examples
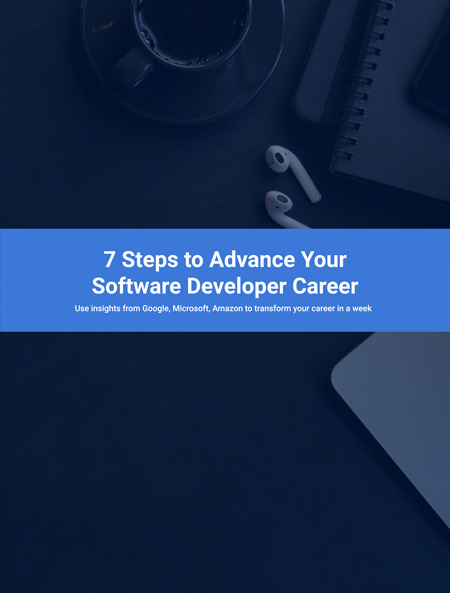
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.