How to print a PDF file in C#? | 4 Easy Ways (FREE and Paid)
Printing a file in C#/.NET is more complicated than it should be. Unfortunately, .NET doesn't have built-in features for talking to a printer.
So let's take a look at some free and paid options for printing a PDF file.
How to print a PDF in C#?
Here are the most common ways to print a PDF file in C#:
Method 1: Copy the file to the network printer
The easiest way to print a PDF file in C# is to just copy it to a network printer:
string filePath = @"C:\Path\To\Your\File.pdf";
string printerSharePath = @"\\ServerName\PrinterShareName";
string fileName = Path.GetFileName(filePath);
string destPath = Path.Combine(printerSharePath, fileName);
// Copy the PDF file to the printer's network share location
File.Copy(filePath, destPath, true);
Keep in mind that some older printers might not support automatic PDF printing.
Method 2: Print using SumatraPDF
public static void PrintUsingSumatraPDF(string fullFilePathForPrintProcess, string printerName, CancellationToken cancellationToken)
{
PrintQueue print_queue = LocalPrintServer.GetDefaultPrintQueue();
Process print = new Process();
print.StartInfo.FileName = "sumatrapdf.exe";
print.StartInfo.UseShellExecute = true;
print.StartInfo.CreateNoWindow = true;
print.StartInfo.WindowStyle = ProcessWindowStyle.Hidden;
print.StartInfo.Arguments = "-print-to \"" + printerName + "\" -exit-when-done \"" + fullFilePathForPrintProcess + "\"";
print.Start();
PrintSystemJobInfo target_job = null;
while (!print.HasExited && target_job == null && !cancellationToken.IsCancellationRequested)
{
print_queue.Refresh();
PrintJobInfoCollection info = print_queue.GetPrintJobInfoCollection();
foreach (PrintSystemJobInfo job in info)
{
if (job.Name.Substring(job.Name.LastIndexOf("\\") + 1) == fullFilePathForPrintProcess)
{
target_job = job;
}
}
Thread.Sleep(50);
}
while(target_job != null && !target_job.IsCompleted && !cancellationToken.IsCancellationRequested)
{
Thread.Sleep(50);
}
}
The above C# method uses SumatraPDF executable to print a PDF file to a specified printer.
It starts a process, waits for the print job to start, and then waits for it to complete printing.
It uses a PrintQueue
object to monitor the print job status and a CancellationToken
to allow the cancellation of the operation.
SumatraPDF is a free and open-source PDF reader and viewer software for Windows. It is lightweight and designed to be fast and easy to use.
Method 3: Print using Adobe Acrobat
If your machine has Adobe Acrobat installed, you can use it to print a PDF.
The process is similar to the one we use to print using SumatraPDF. However, I prefer using SumatraPDF because it's an open-source solution, Adobe Acrobat is not.
Here's how to print using Adobe Acrobat:
public static void PrintUsingAdobeAcrobat(string fullFilePathForPrintProcess, string printerName)
{
string printApplicationPath = Microsoft.Win32.Registry.LocalMachine
.OpenSubKey("Software")
.OpenSubKey("Microsoft")
.OpenSubKey("Windows")
.OpenSubKey("CurrentVersion")
.OpenSubKey("App Paths")
.OpenSubKey("Acrobat.exe")
.GetValue(String.Empty).ToString();
const string flagNoSplashScreen = "/s";
const string flagOpenMinimized = "/h";
var flagPrintFileToPrinter = string.Format("/t \"{0}\" \"{1}\"", fullFilePathForPrintProcess, printerName);
var args = string.Format("{0} {1} {2}", flagNoSplashScreen, flagOpenMinimized, flagPrintFileToPrinter);
var startInfo = new ProcessStartInfo
{
FileName = printApplicationPath,
Arguments = args,
CreateNoWindow = true,
ErrorDialog = false,
UseShellExecute = false,
WindowStyle = ProcessWindowStyle.Hidden
};
var process = Process.Start(startInfo);
process.EnableRaisingEvents = true;
if (process != null)
{
if (!process.HasExited)
{
process.WaitForExit();
process.WaitForInputIdle();
process.CloseMainWindow();
}
process.Kill();
process.Dispose();
}
}
Get the path of the Acrobat executable by accessing the registry keys.
Construct command line arguments to print the PDF file to a specified printer using Acrobat.
Create a
ProcessStartInfo
object with the path to the Acrobat executable and the constructed command line arguments.Start a new process using the
Process.Start()
method with theProcessStartInfo
object.- Enable raising events for the process.
Wait for the process to finish printing the file by calling the
WaitForExit()
method.- Close the process window and dispose of the process.
If the process is still running after a set amount of time, kill and dispose of the process.
Keep in mind, printing using Adobe Acrobat is not always going to be the smoothest. The application is not designed for command-line printing and there may be issues with printer window staying open.
Method 4: Using a commercial package (Paid)
Commercial solutions for printing a PDF file in .NET work the best.
If you want to do it right, you'll have to invest $1,000+ per year in one of the solutions.
Some commercial solutions for printing PDF files in .NET include:
IronPDF
IronPDF is a commercial library for .NET that provides tools for creating, editing, and extracting PDF content in .NET projects.
IronPDF offers features such as generating PDFs from HTML, URLs, JavaScript, CSS, and various image formats, adding headers/footers, signatures, attachments, and passwords and security, performance optimization, and more.
PDFPrinting.NET
PDFPrinting.NET is a simple-to-use API for printing, displaying, and converting PDF documents in .NET applications. It features a built-in font rendering engine, advanced rasterization engine for image printing, and works with any type of printer. The library is a standalone .NET assembly and does not depend on third-party libraries, making it easy to integrate and use in any project.
Spire.PDF
Spire.PDF for .NET is a standalone .NET API used for creating, editing, handling, and reading PDF files without any external dependencies, including Adobe Acrobat. The API offers many rich features, including digital signature, PDF merge/split, metadata update, and converting HTML, XPS, Text, and Image to PDF. Additionally, Spire.PDF for .NET supports security features, data extraction, and document settings features. The Pro Edition costs $999, while the Free Edition is limited to 10 pages of PDF.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →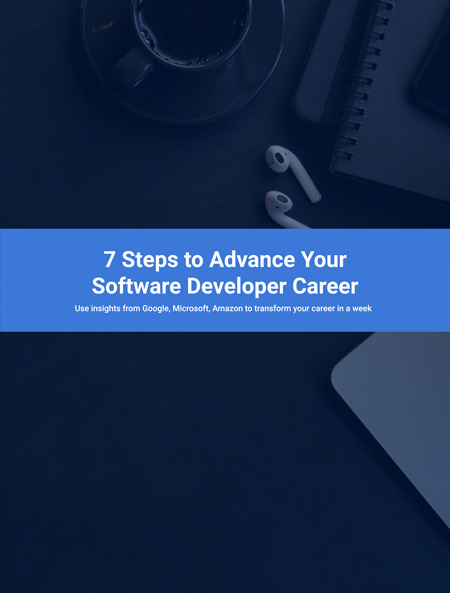
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.