C# protected: How to use it?
C# has several access modifiers that control the visibility of types and members.
The purpose of access modifiers is to prevent the misuse of members that are not ready for public use.
Access modifiers are important for developers because they make code easier to read and write.
One of the most important access modifiers is the protected
.
What is protected in C#?
Protected is a keyword that C# uses to make access restriction for class members.
When we mark members as protected, it becomes accessible only in the class where it's defined or inside the derived class.
The protected keyword is used to share functionality that derived classes might find useful.
In C#, we can use protected
access modifier in 3 combinations:
- Protected
- Protected internal
- Protected private

Protected
The protected access modifier lets you make variables and methods accessible only by code in the same class or struct, or in a derived class.
Protected makes members:
- accessible to all classes that extend the class regardless of the assembly
Protected internal
C# 7.2 added protected internal keyword.
Protected internal makes members:
- accessible to all classes that extend the class
- accessible to all other classes in same assembly
Private protected
Private protected access modifier is more restrictive than either protected or internal.
Private protected makes members:
- accessible ONLY to classes that extend the class AND are in same assembly
You have access to a private protected member only from code that’s in the same assembly and is within a subclass of the member declaration.
When to use protected in C#?
You can use the protected accessibility modifier to help children have access to their parents' properties. This is helpful when you have a base class that many subclasses derive from.
With protected, you can make a common property protected so that it is easy to maintain and reuse common logic.
One of the main benefits of protecting members is that it makes your code more robust and consistent. This helps to reduce the risk of bugs ad spaghetti code because it prevents other classes from modifying these behaviors.
Naming convention
Private, protected, internal, and protected internal fields and properties are named using _camelCase.
That naming convention clarifies that protected members are different root classes, and should not be mistaken for fields or properties.
Accessibility and inheritance
C# has four levels of accessibility and inheritance:
- public - available to all
- private - accessible only from within the type that declared them
- internal - members are available to code defined in the same component
- protected - members are available to code within the type that declared them, and subclasses of that type
C# Protected Vs Private
The main difference between protected and private is that private hides the member from further derivation, while protected allows access in subclasses of its own type.
Example
In this example, derived class SubOrder
cannot access the private member tax
:
public class Order
{
private decimal tax = 0;
public void Calculate()
{
}
}
public class SubOrder : Order
{
public SubOrder()
{
tax = 5;
}
}
If we ran this, we would get Compilation error:
'Program.Order.tax' is inaccessible due to its protection level
However, if we change the tax
variable to protected
, the above code will compile:
public class Order
{
protected decimal tax = 0;
public void Calculate()
{
}
}
public class SubOrder : Order
{
public SubOrder()
{
tax = 5;
Console.WriteLine(tax); // 5
}
}
C# Protected Internal vs Private Protected
The main difference between protected internal and private protected is that code can access the protected internal member in the same assembly.
Protected private members are accessible only to classes that extend the class and are in the same assembly.
Example
public class Example
{
protected internal decimal CalculateTax()
{
return 0;
}
}
public class Order
{
public void Calculate()
{
var taxTotal = new Example();
taxTotal.CalculateTax();
}
}
Even though the Order
class doesn't derive from the Example
class, it can still call the CalculateTax
method because this member is protected internal.
What's an assembly?
Assembly is a collection of types and resources that can be combined to form a logical unit of functionality.
C# packs assemblies as versioned files that we can reuse in other projects. For example, we can take advantage of third-party libraries like Entity Framework or ASP.NET MVC.
When we use a reference to a shared assembly, it becomes a dependency that we need to have available on our build machine.
What is inheritance?
Inheritance is a way of deriving new classes from existing classes. The new class is said to be derived from the old one, which is called the base class.
The most common example is when we are deriving a subclass from a parent class. We can do this by adding colon after the name of the base class, followed by an identifier for the derived class.
For example:
public abstract class Animal
{
public void Eat()
{
Console.WriteLine("I'm hungry");
}
}
public abstract class Mammal : Animal
{
public override void Eat()
{
Console.WriteLine("Nom Nom");
}
}
The Mammal
class is derived from Animal
and it inherits all the members of its base class.
Conclusion
C# protected is used to allow derived classes access to base class properties and methods with the same name as long as those properties and methods are not private.
As with other access modifiers, protected can be used with fields, properties and methods.
Securing the inheritance helps follow object-oriented principals and the concept of polymorphism.
This new modifier can decrease coupling within the base class and help define abstractions between derived classes by allowing access only when needed.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →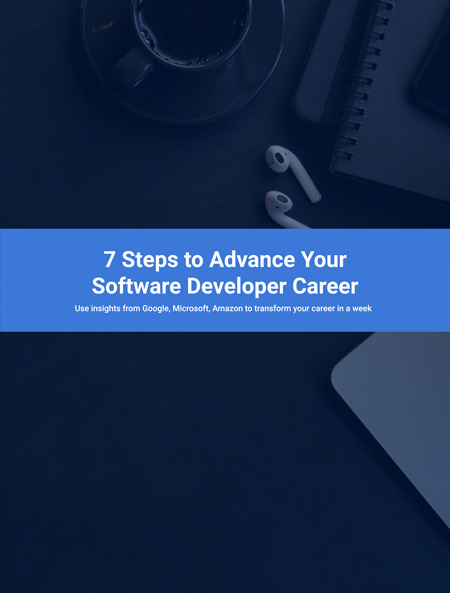
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.