C#: Why is string a reference type?
C#: String reference type
In C#, a string is a reference type because it is an object that is stored on the heap rather than the stack. This means that when you create a string variable and assign it a value, you are creating a reference to an object on the heap that contains the string value.
The main reason that strings are reference types in C# is that they are immutable, which means that once a string object is created, its value cannot be changed. If strings were value types, every time you wanted to modify the value of a string, a new string object would have to be created and the old one would have to be discarded, which would be inefficient and waste a lot of memory. By using a reference type, C# can avoid this overhead and allow strings to be modified more efficiently.
Another reason that strings are reference types in C# is that they can be very large, and it would be inefficient to store them directly on the stack. By using a reference type, C# can store a string on the heap and only use a small reference to it on the stack, which is more efficient in terms of memory usage.
Overall, the choice to make string a reference type in C# was made to balance the need for efficiency with the need to allow strings to be modified and to support large string values.
String reference type example
Here's an example of how strings work as reference types in C#:
string str1 = "Hello";
string str2 = str1;
// At this point, str1 and str2 both reference the same string object on the heap
str2 = "World";
// Now, str2 references a new string object on the heap with the value "World"
// str1 still references the original string object with the value "Hello"
Console.WriteLine(str1); // Outputs "Hello"
console.WriteLine(str2); // Outputs "World"
In this example, we create a string str1 and assign it the value "Hello". Then we create another string str2 and assign it the value of str1. At this point, str1 and str2 both reference the same string object on the heap with the value "Hello".
Then we modify the value of str2 by assigning it a new string "World". This creates a new string object on the heap with the value "World" and assigns str2 to reference this new object. However, str1 still references the original string object with the value "Hello", so when we print the values of str1 and str2, we get "Hello" and "World" respectively.
This demonstrates how strings behave as reference types in C# - when you assign one string variable to another, you are creating a reference to the same object rather than creating a new copy of the object.
Main characteristics of reference types in C#
The key characteristics of reference types in C# include:
- Reference types are stored on the heap, rather than on the stack.
- Reference types are passed by reference, rather than by value. This means that when you pass a reference type to a method or assign it to a new variable, you are passing or assigning a reference to the memory location of the object, rather than creating a new copy of the object.
- Reference types are initialized to null by default, unless you explicitly assign them a value. Reference types support inheritance, which means that you can create a new class that derives from an existing class and inherits its properties and methods.
- Reference types are garbage collected, which means that the .NET runtime automatically frees up memory that is no longer being used by reference type objects. This helps to prevent memory leaks and ensure that the program's memory usage stays within a certain limit.
- You can use the null keyword to represent a null reference, which is a reference that does not point to any object in memory. Attempting to call a method or access a property of a null reference will result in a NullReferenceException.
- Reference types can implement interfaces, which are contracts that define a set of methods that a class must implement. This allows you to create objects that have a specific set of behaviors, regardless of their specific implementation.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →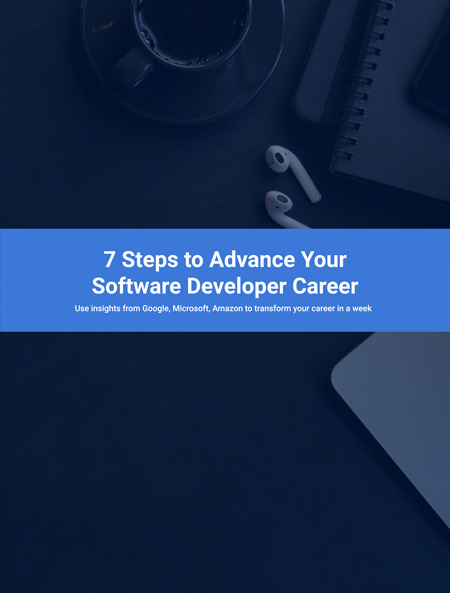
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.