React: Functional Components vs Class Components
React: Functional Components vs Class Components
Functional and class components in React differ in syntax and lifecycle management. Functional components use a function with a return statement to render, class components use render()
. Function components use hooks, class components use React lifecycle methods. Since 2019, function components are more popular, class components are outdated.
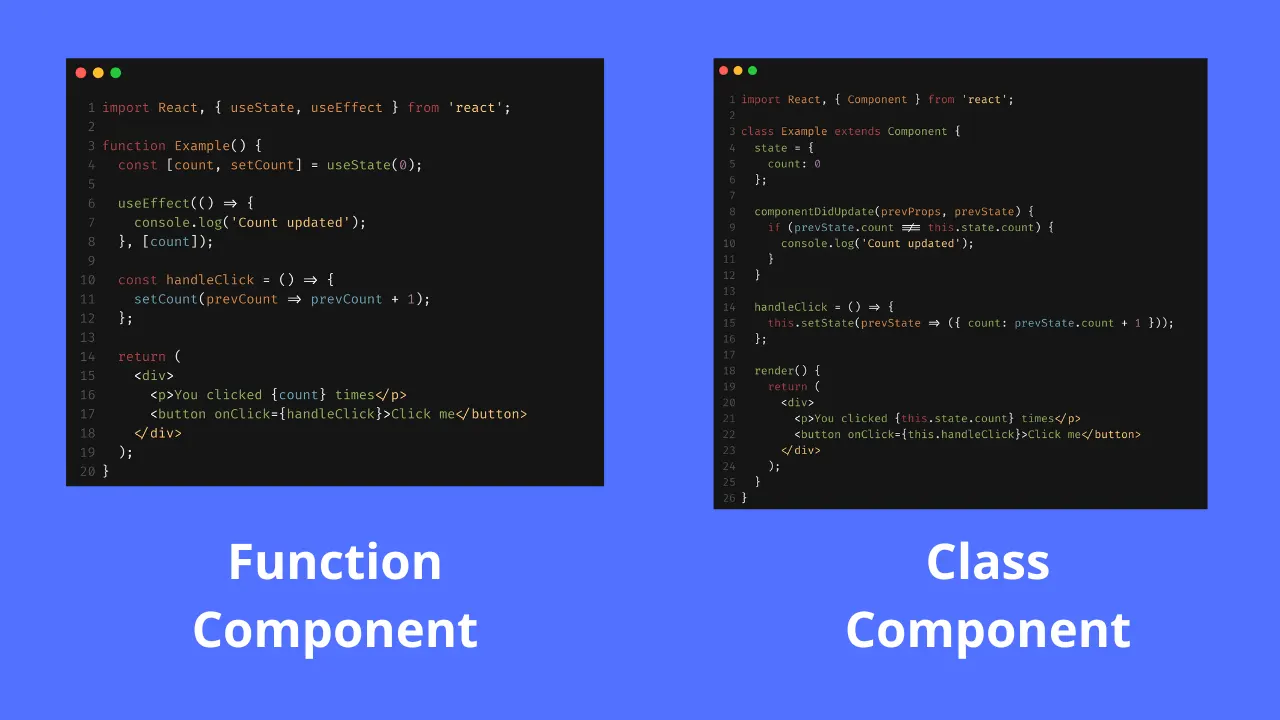
Function Components | Class Components | |
---|---|---|
Definition | JavaScript function that returns a JSX element | JavaScript class that extends React.Component and has a render() method that returns a JSX element |
State | Uses React Hooks to manage state | Has built-in state management using this.state |
Lifecycle Methods | Uses React Hooks to handle lifecycle events like | Uses class lifecycle methods like |
Props | Props are passed as a parameter to the function | Props are accessed through this.props |
Render | The component is defined within the function body and returned as a JSX element | The render() method is required and returns a JSX element |
Code Clarity | Simple and concise | More verbose and harder to read |
Performance | Sets you up to follow best practices | Easier to cause performance issues |
React Hooks
React 16.8 release in 2019 changed everything.
It introduced React hooks.
React hooks are functions that let functional components manage state, handle lifecycle events, and access other React features.
Before hooks, you could only do that using class components.
Are function components faster than class components?
On their own, function components are not faster than class components.
Your code inside components matters more than the style you pick.
However, using functional components will set you up to use best practices. In return, you'll see performance benefits.
React Team designed Hooks to be more efficient by avoiding overhead that classes require and reducing the need for deep component tree nesting.
Hooks also offer optimizations like useCallback
and useMemo
to address performance concerns related to inline functions.
Should I use function components or class components?
You should use function components over class components.
Function components allow you to use Hooks. That means you can use additional functionality and make it easier to manage state and side effects.
However, if you really need to use lifecycle methods, class components may still be necessary.
For example, I'm working on a project that doesn't support Redux Hooks. So if I want to use Redux, I need to create a class component.
Function vs Class: State
To manage the state in function components, we use the useState
hook. In class components, you can use state by defining a state object in the class's constructor and using this.setState to update it.
State with Function Components
import React, { useState } from 'react';
function Example() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(prevCount => prevCount + 1);
}
return (
<div>
<p>You clicked {count} times</p>
<button onClick={handleClick}>
Click me
</button>
</div>
);
}
State with Class Components
import React, { Component } from 'react';
class Example extends Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState(prevState => ({ count: prevState.count + 1 }));
}
render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button onClick={this.handleClick}>
Click me
</button>
</div>
);
}
}
Function vs Class: Lifecycle methods
Here's a table showing the equivalent of class component lifecycle methods using React Hooks:
Class Component | Hook Equivalent |
---|---|
componentDidMount |
|
componentDidUpdate |
|
componentWillUnmount |
|
shouldComponentUpdate |
|
getDerivedStateFromProps |
|
getSnapshotBeforeUpdate | no direct hook equivalent, but can be achieved with refs |
Function vs Class: Monitor state changes
Here's an example of using componentDidUpdate
in a class component and the equivalent using the useEffect
hook in a functional component:
State with Function Components
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log('Count updated');
}, [count]);
const handleClick = () => {
setCount(prevCount => prevCount + 1);
};
return (
<div>
<p>You clicked {count} times</p>
<button onClick={handleClick}>Click me</button>
</div>
);
}
State with Class Components
import React, { Component } from 'react';
class Example extends Component {
state = {
count: 0
};
componentDidUpdate(prevProps, prevState) {
if (prevState.count !== this.state.count) {
console.log('Count updated');
}
}
handleClick = () => {
this.setState(prevState => ({ count: prevState.count + 1 }));
};
render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button onClick={this.handleClick}>Click me</button>
</div>
);
}
}
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →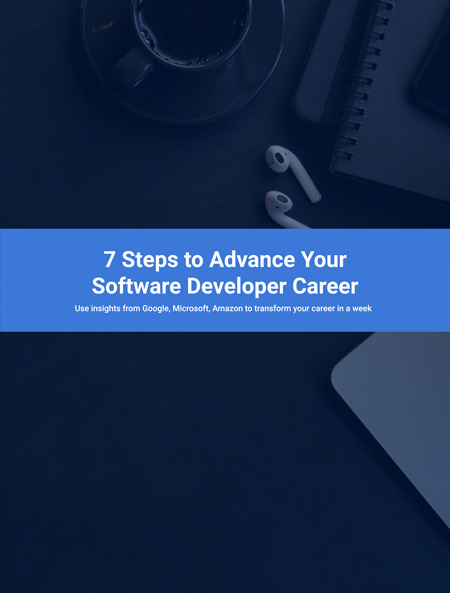
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.