REST API Authentication: 9 Proven Methods
Authentication is one of the key components of REST API best practices.
No one wants their APIs to be hacked.
But how do you strike a balance between security, simplicity, and developer user experience?
In this article, find out which method is the best for your REST API authentication.
There are 9 main approaches to authentication in REST APIs:
1. Basic Authentication
HTTP Basic Authentication is a simple method for authentication using a standard HTTP header.
It involves sending the username and password, encoded using Base64, in the "Authorization" header.
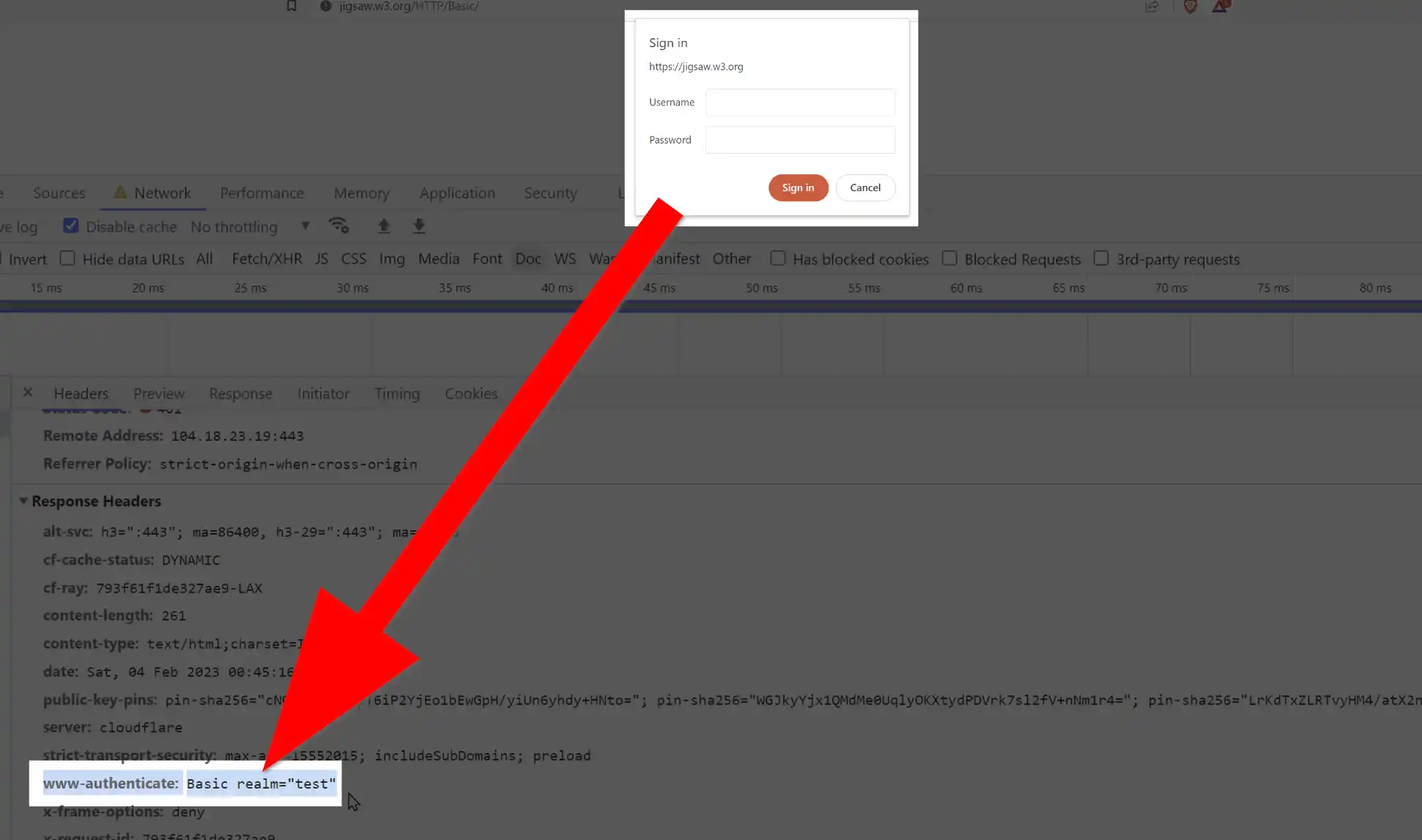
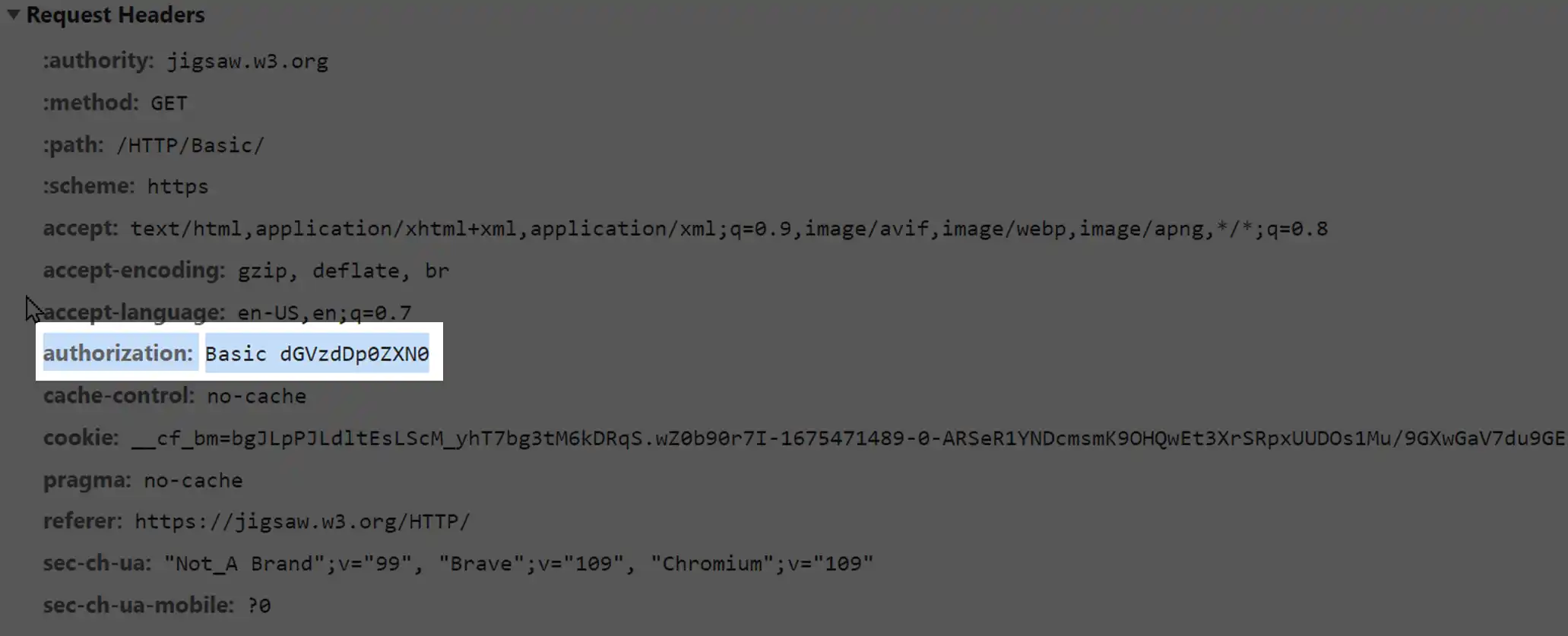
Basic authentication credentials should only be sent over an encrypted connection. If used over unencrypted networks, anyone can easily decode a base64 string. Hence, using Secure Sockets Layer (SSL) and Transport Layer Security (TLS) channels is a must when using Basic Authentication.
IETF clearly defined Basic Auth in RFC 7617.
2. Bearer Token
The Bearer token is a standard way to pass tokens to an API for authentication defined by RFC 6750.
It is widely adopted for token-based authentication and is used by including the token in the Authorization header without additional encoding.
If you have a bearer token, you don't need any further proof of authentication.
Are Bearer tokens just a way of sending tokens?
Yes, bearer tokens are more of a transportation method rather than an authentication method.
How you get the token is a different story. They were made popular by OAuth, but since then they've been used for different use cases. For example, Private Access Tokens (PATs).
Here's an example from Atlassian's API:
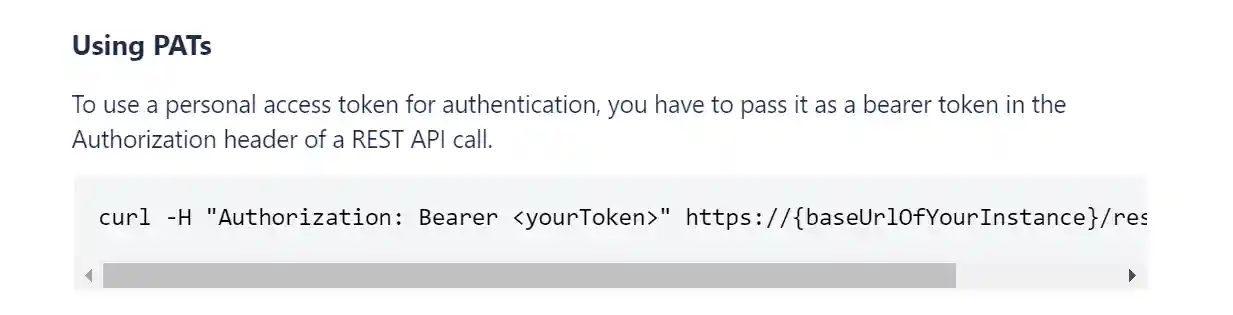
Bearer Token format example:
Authorization: Bearer QDAmQ9TStkDCpVK5A9kFowtYn2k
3. API Keys
API Key authentication approach uses a key-value combination.
API keys can be sent as part of the request:
- payload,
- HTTP headers, or
- query string.

The server can invoke credentials and tokens as needed. For example, if a user's permission level changes or if the client compromises the key.
API keys are susceptible to the same risks as Basic authentication because they don't expire. If someone steals them, the only thing you can do is revoke them.
4. Cookies
Session cookies are a common implementation of token-based authentication.
- The login endpoint returns a "Set-Cookie" header on the response which instructs the API client to store the session token.
- Subsequent requests include the token as a "Cookie" header.
- The server receives the client cookie and looks up the cookie token to identify the user.
A big advantage of cookies is the "Secure" security attribute. Cookies are associated with a domain and can be marked as "Secure" to prevent accidental sending over a non-HTTPS connection.
Are Cookies RESTful?
The use of cookies in RESTful API may violate the principle of statelessness, but when used solely for API authentication, they are fine.
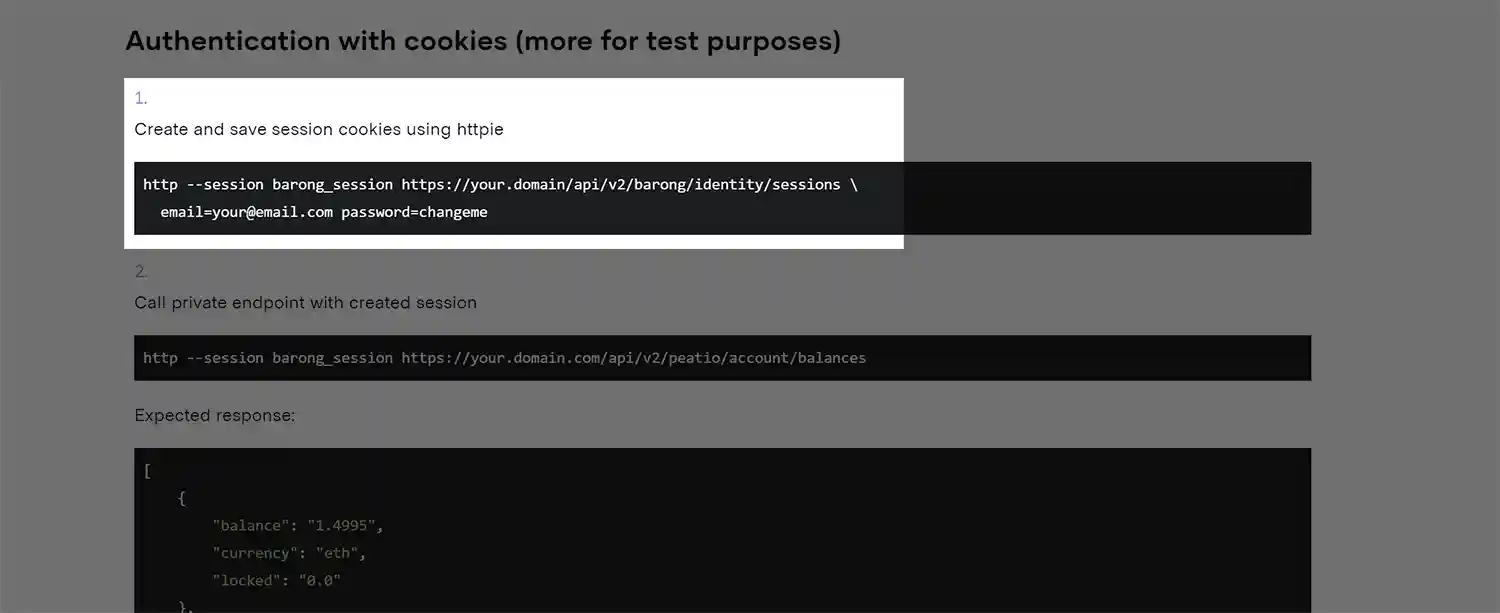
5. OAuth 2.0
OAuth 2.0 is considered a gold standard when it comes to REST API authentication, especially in enterprise scenarios involving sophisticated web and mobile applications.
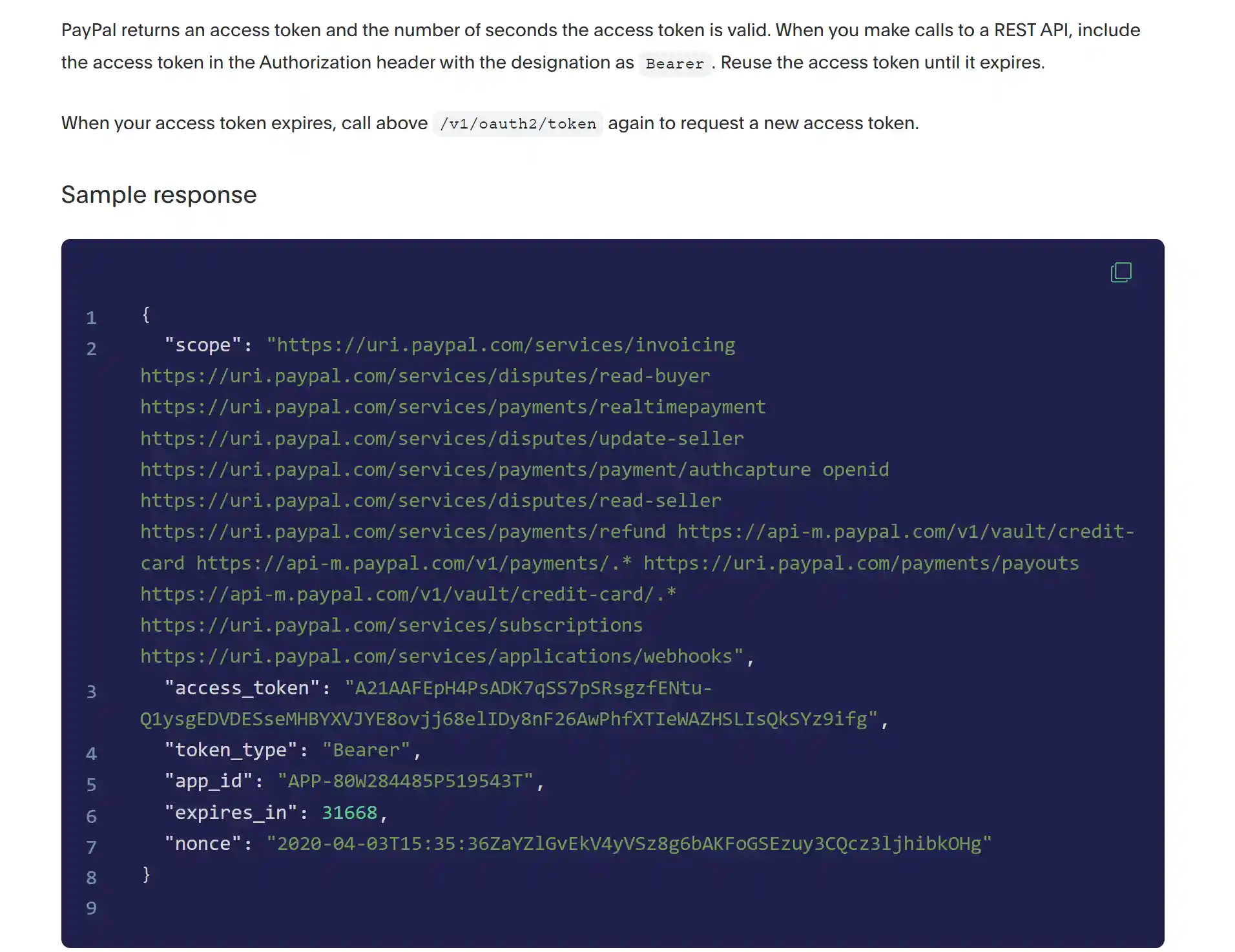
Benefits of expiration
Unlike regular API keys, OAuth tokens have expiration date. That's a major security benefit.So even if someone steals your token, they'll have limited time to use it.
The downside is that you have to periodically renew the token which makes the integration more complex.
More than Authentication
OAuth is not only about authentication, but also authorization.
OAuth supports the concept of scopes, a method of limiting an application's access to a user's account and associated credentials.
OAuth 2.0 can support dynamic collections of users, permission levels, scope parameters and data types.
JWT
OAuth 2.0 does that using JWTs.
JSON Web Tokens (JWT) are a method for representing claims securely between two parties.
JWT tokens securely store encrypted, user-specific data, and then transmit that data between applications as needed.Because these web tokens can be signed, it's possible to imbue the token with new user roles and permissions once the user has been authenticated.
This mitigates the risk of creating a single point of failure in the validation process. However, managing the distribution of symmetric keys required for payload integrity verification is a daunting security challenge in dynamic, highly scalable application environments.
OAuth History
OAuth was first developed in 2006 by Blaine Cook, Chris Messina, and Larry Halff. They were looking for a way to allow OpenID users to authorize to access their service.
As a result of their efforts to standardize the process, they formed a discussion group in 2007. The OAuth Core 1.0 final draft was released in December of that year. Soon after, Google's DeWitt Clinton joined the effort and they polished an RFC in April 2010.
Since then, all third-party Twitter applications have been required to use OAuth. The protocol has been updated over the years with OAuth 2.0 being published as a standards track RFC in October 2012.
6. OpenID Connect
OpenID Connect is an identity layer built on top of the OAuth 2.0 protocol.
It provides a standard for authentication and allows users to authenticate to a web application using an existing account from a different site such as Google, Facebook, or GitHub.
If you're REST API requires secure user data transfer across multiple third-party web applications, OpenID Connect is the easiest way to do it.
7. Mutual TLS (mTLS)
Mutual TLS authentication is a two-way authentication process between a client and server. Both the client and server must present X.509 certificates to verify their identity.
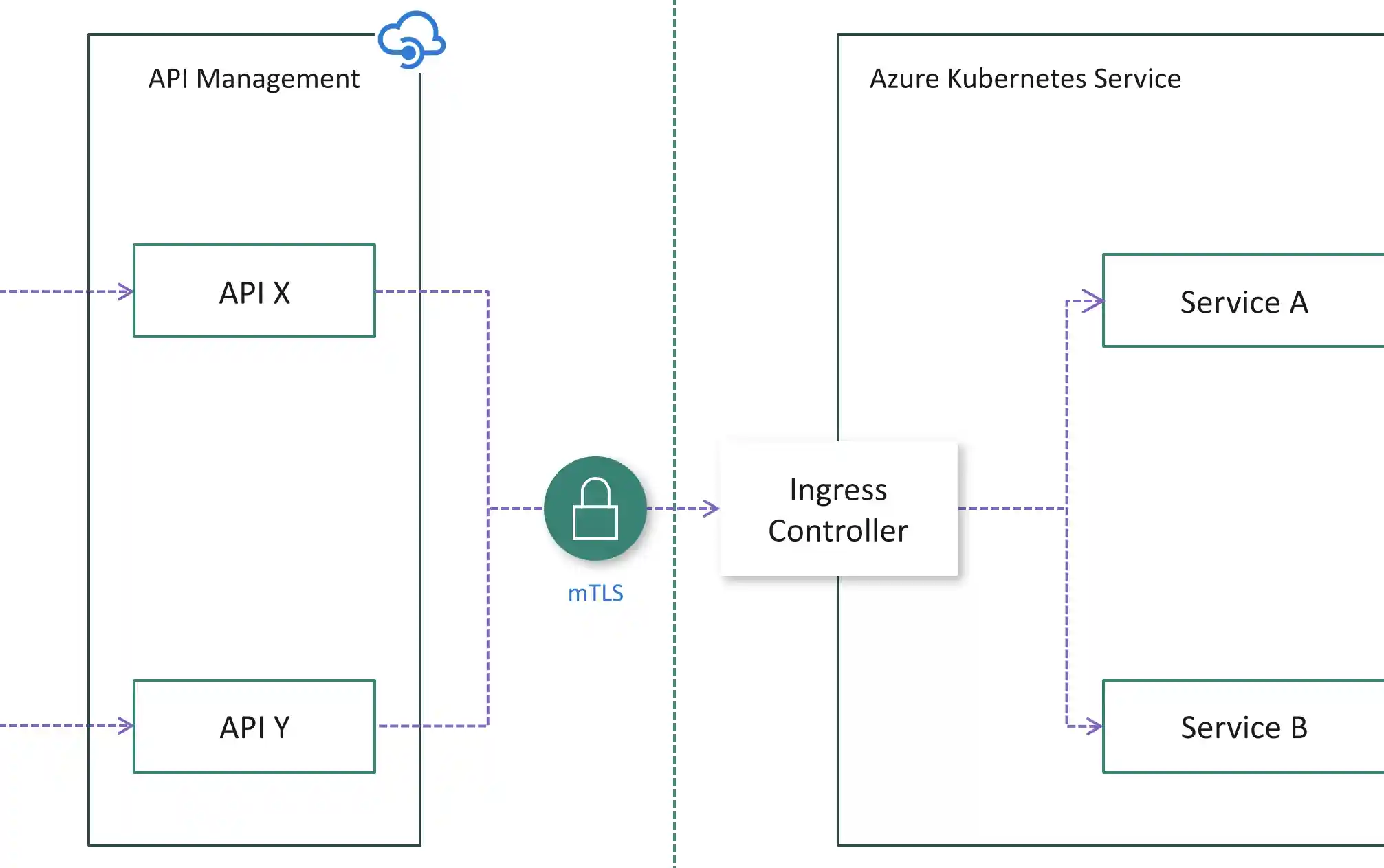
Mutual TLS is commonly used for Internet of Things (IoT) and business-to-business applications.
Mutual authentication requires both the client and server to authenticate themselves to each other using an SSL/TLS handshake with certificate exchange and validation. Authorization is handled separately from the authentication process.
8. IP Access Restriction
IP Access Restriction is a method of REST API authentication. It restricts access to an API based on the IP address of the client making the request.
You maintain a list of allowed IP addresses, and the API only accepts requests from these IP addresses. If a request is made from an IP address that is not in the allowed list, the API can return a 401 Unauthorized response or any other relevant HTTP error code.
IP Access Restriction is a simple and effective way to secure APIs that don't have too many consumers. For example, B2B APIs or internal systems.
Keep in mind that IP Access Restriction can become complex if the client making the request is behind a proxy or a load balancer.
9. HTTP Digest
HTTP Digest is an authentication scheme supported by HTTP, which sends a salted hash of the password instead of the raw value.
A big downside of this method is the hashing algorithm. HTTP Digest uses MD5 which is now considered insecure.
Do yourself a favor and avoid using HTTP Digest authentication in new applications.
HTTP Digest access authentication has been introduced in 1997 as part of RFC2069.
FAQ: REST API Authentication
What is token-based authentication?
Token-based authentication is a method that consists of several steps:
- the client makes a request to a login endpoint with the user's credentials
- the client receives a time-limited token
- the client then sends this token as part of requests to other API endpoints
- every API endpoint validates the token by checking it against a token database
What is JWT?
JWTs are a type of security token that provides a way to securely store and transfer information.
They are made up of two parts:
- a JSON object that contains claims about a user,
- a header that describes the format of the token.
JWTs are digitally signed and encrypted, making them secure against tampering.
The token is created by serializing the session fields into a JSON object, then encoding the object into a string that acts as the token ID. When presented back to the API, the token is decoded and parsed to recover the session attributes.
What is HMAC Encryption?
HMAC (hash-based message authentication code) is a secure system for protecting tokens.
It is based on a cryptographic hash function and incorporates a secret key known only to the API server. When used to authenticate tokens, HMAC produces a short authentication tag that is appended to the token.
If even a single bit of the token is altered, the tag will change, preventing the attacker from tampering with or faking new tokens. Furthermore, HMAC-SHA256 can immediately reject requests if the authentication tag is invalid or missing, without any database lookups, preventing any timing attacks.
Is a 401 response required for unauthenticated requests to APIs with Basic Authentication?
Basic Authentication needs to respond with 401 for unauthenticated requests as defined by RFC2617.
However, that could cause an information security leak.
For example, the GitHub API uses a version of Basic Authentication that is slightly different from the standard defined in RFC2617. According to the standard, unauthenticated requests should receive a 401 Unauthorized response, but this could disclose the existence of user data. To avoid this, the GitHub API returns a 404 Not Found response instead.
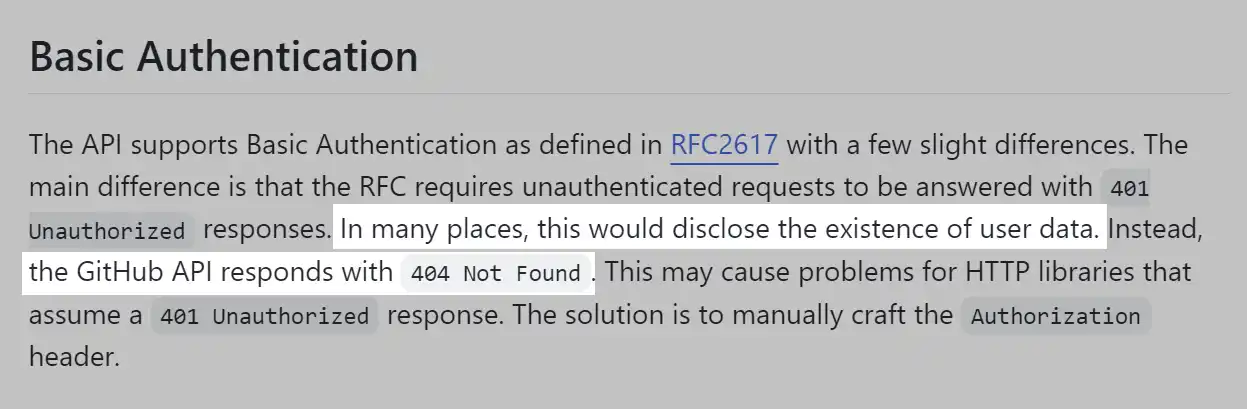
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →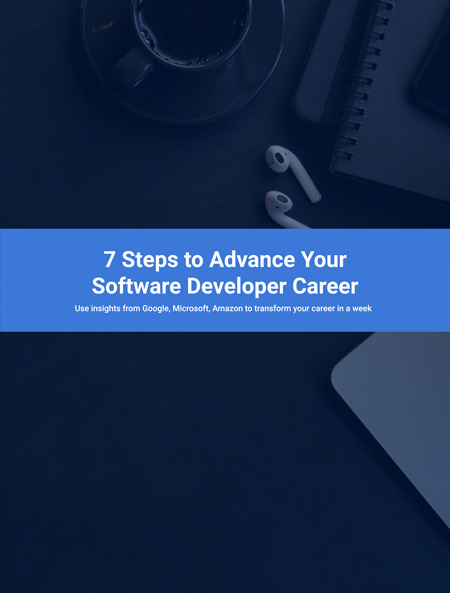
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.