47 Terraform Interview Questions [2023]
This is a list of top Terraform interview questions that employers ask candidates during interviews in 2023.
Beginners Terraform interview questions
What is Terraform?
Terraform is a tool that helps you build, change and version your infrastructure safely and efficiently. It can manage existing providers like popular services such as Azure and AWS, as well as custom in-house solutions.
The key features of Terraform are:
- Infrastructure as Code: Infrastructure is described using a high-level configuration syntax. This allows a blueprint of your data center to be versioned and treated like any other code. Infrastructure can be shared and re-used.
- Execution Plans: Terraform has a "planning" step where it generates an execution plan. The execution plan shows what Terraform will do when you call apply. This lets you avoid any surprises when Terraform manipulates infrastructure.
- Terraform creates a graph of all your resources, and it parallelizes the creation and modification of any non-dependent resources. This makes Terraform build infrastructure as efficiently as possible and gives operators insights into dependencies in their infrastructure.
- Complex changesets can be applied to your infrastructure with little or no human interaction. With the previously mentioned execution plan and resource graph, you know exactly what Terraform will change and in what order, avoiding many possible human errors.
What does the terraform plan
command do?
The Terraform plan command is used to create an execution plan. Terraform performs a refresh, unless explicitly disabled, and then determines what actions are necessary to achieve the desired state specified in your configuration files.
The terraform plan
command is a convenient way to check whether the execution plan for a set of changes matches your expectations, making no changes to real resources or the state.
What is infrastructure as code (IAC)?
Infrastructure as Code (IaC) is the managing and provisioning of infrastructure through code instead of through manual processes.
With IaC, you create files with your infrastructure specifications. This makes it easier to edit and distribute configurations. It also ensures that you provision the same environment every time.
The main benefits of infrastructure as code over manual processes are:
- Scalability: You can quickly and easily provision new infrastructure as your needs change.
- Repeatability: You can consistently reproduce your environment, making it easier to troubleshoot issues.
- Version Control: By storing your configurations in code, you can use version control tools to track changes. This way, you can roll back to previous versions if necessary.
- Automation: Automating the provisioning of infrastructure reduces the chances of human error.
What is the difference between terraform plan
and terraform apply
?
The main difference between terraform plan
and terraform apply
is that terraform apply
actually applies the changes to your infrastructure, while terraform plan
only creates an execution plan.
Another difference is that terraform apply
accepts a set of input variables, while terraform plan
does not.
What is a state file in Terraform?
A state file is a json file that Terraform creates after it successfully creates infrastructure. This file contains information about the resources Terraform created, and is used by Terraform to know what resources it handles.
How to write comments in Terraform?
To write comments in Terraform, you can use the "#" character. Anything that comes after a "#" character will be ignored by Terraform.
For example:
# This is a comment
resource "aws_instance" "example" {
# The next line specifies the instance type
instance_type = "t2.micro"
}
What happens if Terraform state file is deleted?
If the Terraform state file is deleted, Terraform cannot manage the resources it created.
To fix this, you would have to create a new state and import your resources into it.
What is a Terraform module?
A Terraform module is a collection of Terraform configuration files that are used to create a specific infrastructure resource. For example, there is a module for creating a virtual network might include configuration files for creating subnets, route tables, and security groups.
We use Terraform modules to create reusable components for complex infrastructure. This way, you don't have to create all the configuration files from scratch each time you want to provision a new resource.
How to import resources in Terraform?
The Terraform import command is used to import existing resources into your Terraform state. This is useful if you have resources that were created outside of Terraform, or by another tool.
To import a resource, you need to know the type of the resource and the name or ID of the resource. For example, to import an Azure Key Vault Secret, run the Terraform import command as:
terraform import azurerm_key_vault_secret.example "https://example-keyvault.vault.azure.net/secrets/example/fdf067c93bbb4b22bff4d8b7a9a56217"
Advanced Terraform interview questions
Where can you store Terraform state?
You can store Terraform state in a local file or in a remote storage backend.
The difference between a local and remote Terraform state is that with a remote state, other members of your team can access the state file. With a local Terraform state, only the person who created it has access.
What is Terraform state locking?
Terraform state lock is a locking mechanism that is used to prevent two users from modifying the state file at the same time.
When a user runs Terraform apply, Terraform will lock the state file. If another user tries to run Terraform apply while the state file is locked, they will receive an error.
How to remove a resource from Terraform state?
To remove a resource from your Terraform state, you can use the terraform state rm
command.
For example, to remove an Azure Key Vault Secret, run the Terraform state rm command as:
terraform state rm azurerm_key_vault_secret.example
When you remove a resource from your Terraform state, it doesn't destroy the resource. The command only removes the resource from your Terraform state.
How to pass variables to a module?
Input variables allow you to customize aspects of Terraform modules without changing the source code for those modules. This way, you can share modules between different Terraform configurations, making your module composable and reusable.
You can set the values of your variables using CLI options and environment variables. If you declare them in child modules, the calling module should pass values to the child module block.
How to use environment variables?
To use environment variables in Terraform, set the TF_VAR_name variable where name is the name of the variable you want to set.
How does Terraform import command work?
The Terraform import command can only add resources to the state. It doesn't create any configuration files. A future version of Terraform will create configuration files as well.
Before running Terraform import, you need to write a resource configuration block for the resource you want to import.
Is Terraform cloud agnostic?
Terraform is cloud-agnostic in that it can manage various cloud infrastructures. Terraform provides a cloud-specific provider for each major cloud provider, such as AWS, Azure, GCP, and so on. But you still need to write different Terraform code for each cloud. So Terraform is not completely cloud agnostic.
How does Terraform init work?
The Terraform init command initializes a working directory for Terraform. This is the first command you should run after creating a new Terraform configuration or cloning an existing one from version control. You can run this command multiple times without any issues.
When to use depends_on in Terraform modules?
Terraform's depends_on argument can be used to explicitly declare a dependency on another resource. This is useful where Terraform cannot determine the correct order in which to create resources, or when you want to override Terraform's default behavior.
For example, if you have a module that creates an Azure VM, and you want to ensure that the VM is created after the network interface.
What are Terraform workspaces?
Terraform workspaces are used to isolate environment state. This is useful when you want to maintain separate states for different environments, such as development, staging, and production.
To create a Terraform workspace, use the Terraform workspace new command.
What are some best practices for workspaces?
When using Terraform workspaces, it is important to keep the following points in mind:
- Always use a separate workspace for each environment
- Do not commit sensitive information to your version control system
- Use state locking to prevent concurrent modifications
What are tfvars?
tfvars are Terraform variables that are stored in a file with a .tfvars extension. These files are used to store sensitive information, such as passwords and API keys.
Tfvars files should not be checked into version control systems, as they can contain sensitive information.
What are some ways to organize Terraform configuration?
There are a few different ways to organize your Terraform configuration:
- Modules: Modules are used to create reusable components for complex infrastructure. This way, you don't have to create all the configuration files from scratch each time you want to provision a new resource.
- Workspaces: Workspaces are used to isolate environment state. This is useful when you want to maintain separate states for different environments, such as development, staging, and production.
- Tfvars files: Tfvars fitles are used to store sensitive information, such as passwords and API keys. These files should not be checked into version control systems.
How do you debug Terraform?
There are a few different ways to debug Terraform:
- Use the -debug flag: This flag will print out additional debugging information.
- Set the TF_LOG environment variable: This variable can set the logging level for Terraform. The potential values are TRACE, DEBUG, INFO, WARN, and ERROR.
- Use the Terraform console command: This command will open an interactive console that you can use to evaluate expressions. This is useful for debugging variables and interpolations.
What are some disadvantages of Terraform?
Main disadvantages of Terraform are:
-
It does not support all cloud platforms
-
It can be complex to use for infrastructure that is not entirely cloud-based
-
There can be a learning curve associated with its use
What providers does Terraform support?
Terraform supports a wide variety of providers, including AWS, Azure, Google Cloud Platform, Oracle Cloud Infrastructure, and many more.
How does composition work in Terraform?
One of the key features of Terraform is its ability to compose complex infrastructure from simple components. This enables you to reuse configuration blocks and create modular infrastructure.
What is Terraform cloud?
Terraform cloud is a cloud-based service that provides an easy way to provision, manage, and collaborate on Terraform configurations. It includes a web-based UI, CLI, and API.
What is Terraform enterprise?
Terraform Enterprise is an on-premises version of Terraform Cloud. It includes all of the same features, but is designed for use in corporate environments.
What is Terraform module registry?
Terraform module registry is a collection of ready-to-use modules for common infrastructure needs. Modules can create reusable components for complex infrastructure.
What is a backend in Terraform?
In Terraform, backend defines how the state is loaded and how an operation such as apply is executed. There are many backend types available in Terraform like AWS S3, Azure storage, GCP storage, Local files etc.
What's the first command you need to run in the Terraform configuration directory?
The first command you need to run in the Terraform configuration directory is "terraform init". This command initializes the Terraform working directory, which contains all the files to manage your infrastructure.
This command also downloads any required plugins for the providers you're using in your configuration. For example, if you're using the AWS provider, Terraform will download the AWS provider plugin.
What is Terragrunt?
Terragrunt is a thin wrapper for Terraform that provides extra functionality for working with multiple Terraform modules.
Some common ways to use Terragrunt include:
- Referencing Terraform modules in other modules
- Passing variables to Terraform modules
- Applying Terragrunt configurations to multiple environments
- Using Terragrunt with a remote state backend
Terragrunt is open source and available on GitHub.
How to use Terraform for automated delivery?
The key to using Terraform for automated delivery is to understand the workflow. The basic idea is that you write your infrastructure code in Terraform, then push it to a remote repository such as GitHub. When you're ready to deploy your changes, you run a script that calls the "terraform apply" command.
For example, we can use Azure DevOps to automate validating, testing and deploying infrastructure is codified and automated via pipelines.
Pipelines give developers feedback quickly when the changes they make to Git do not work as expected or are not validated.
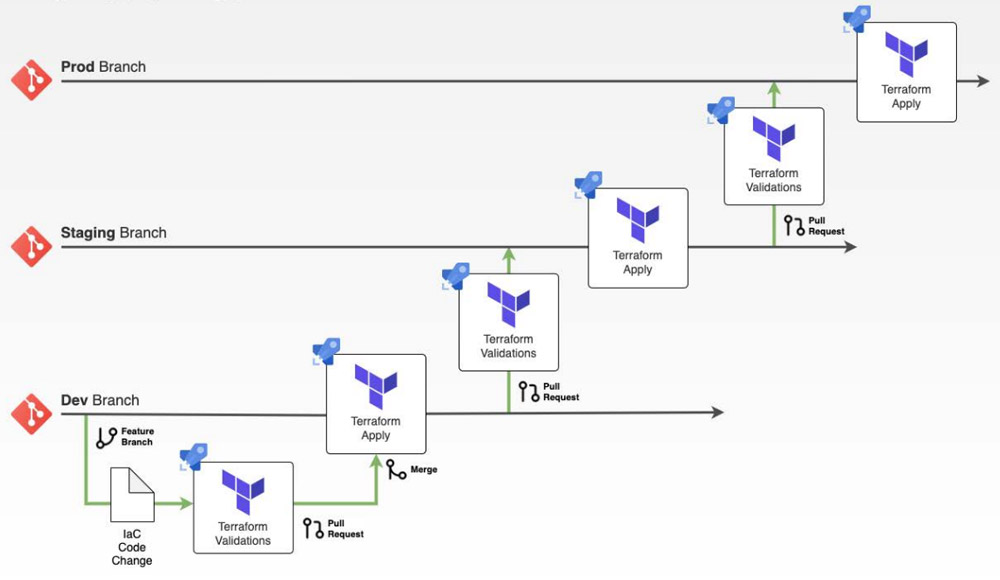
What is Terraform configuration file?
Terraform configuration files are used to describe the desired state of your infrastructure. The files are written in the HashiCorp Configuration Language (HCL), and they usually have a ".tf" extension. The Terraform code in these files is used to provision and manage resources in your infrastructure.
The configuration files can be used to create, update and delete resources. They can also be used to manage dependencies between resources, and to define input and output variables for modules.
What to remove Terraform resource?
To remove a Terraform resource, you can use the "terraform destroy" command. This command will delete all the resources that were created by Terraform.
Before running this command, make sure that you have a backup of your Terraform state file. The state file contains all the information about the resources that Terraform is managing.
How to lock Terraform module versions?
The "version" attribute can be used in a module in a Terraform configuration file if the Terraform Module Registry is being used as a source. If GitHub is being used as a source, then the branch and versions can be specified in the query string by using "?'ref'".
What is Terraform Core?
Terraform Core is the main component of Terraform. It is a Go binary that is used to execute Terraform plans. Terraform Core includes the following:
- A CLI that can be used to apply plans, destroy resources, and manage modules
- A built-in state management system
- A plugin SDK for developing providers
Terraform Core is open source and available on GitHub.
What are some Terraform commands?
Terraform has many commands, but some of the most common are:
- Terraform init: This command is used to initialize a Terraform working directory. It downloads any required plugins for the providers being used in the configuration.
- Terraform plan: This command is used to create an execution plan. The execution plan is a description of the actions that Terraform will take to reach the desired state.
- Terraform apply: This command is used to apply the changes described in the execution plan. This will create or update resources in your infrastructure.
- Terraform destroy: This command is used to destroy resources that have been created by Terraform.
- Terraform refresh: This command is used to update the state file with the real-world resources. This is useful when you make changes to your infrastructure outside of Terraform.
- Terraform output: This command is used to print the values of output variables. This is useful for debugging or for creating scripts that use Terraform data.
- Terraform show: This command is used to print the contents of the state file. This is useful for debugging or for understanding how Terraform represents your infrastructure.
Senior Terraform interview questions
What are some ways to apply the DRY principle in Terraform?
One of the ways to apply the DRY principle (don't repeat yourself) in Terraform is to use modules. Modules allow you to create reusable components for your infrastructure. This way, you don't have to write the same configuration files repeatedly.
Another way to apply the DRY principle is to use variables. Variables allow you to parameterize your configurations, so that you can reuse the same configuration files with different values.
Finally, you can use Terraform's built-in functions to create reusable snippets of code. For example, the "list" function can create a list of resources, which can then be iterated over using the "for" loop.
If the list of environments or regions that you're deploying to grows large, consider using a CSV file that you can import into Terraform using the "csvdecode" function, as suggested in this presentation.
Conclusion
Terraform interview questions and answers are important for anyone who is looking to get into DevOps or Infrastructure as a Code. These questions can help you understand the tool better and also prepare you for any interview questions that may come your way.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
- Beginners Terraform interview questions
- What is Terraform?
- What does the terraform plan command do?
- What is infrastructure as code (IAC)?
- What is the difference between terraform plan and terraform apply?
- What is a state file in Terraform?
- How to write comments in Terraform?
- What happens if Terraform state file is deleted?
- What is a Terraform module?
- How to import resources in Terraform?
- Advanced Terraform interview questions
- Where can you store Terraform state?
- What is Terraform state locking?
- How to remove a resource from Terraform state?
- How to pass variables to a module?
- How to use environment variables?
- How does Terraform import command work?
- Is Terraform cloud agnostic?
- How does Terraform init work?
- When to use depends_on in Terraform modules?
- What are Terraform workspaces?
- What are some best practices for workspaces?
- What are tfvars?
- What are some ways to organize Terraform configuration?
- How do you debug Terraform?
- What are some disadvantages of Terraform?
- What providers does Terraform support?
- How does composition work in Terraform?
- What is Terraform cloud?
- What is Terraform enterprise?
- What is Terraform module registry?
- What is a backend in Terraform?
- What's the first command you need to run in the Terraform configuration directory?
- What is Terragrunt?
- How to use Terraform for automated delivery?
- What is Terraform configuration file?
- What to remove Terraform resource?
- How to lock Terraform module versions?
- What is Terraform Core?
- What are some Terraform commands?
- Senior Terraform interview questions
- What are some ways to apply the DRY principle in Terraform?
- Conclusion
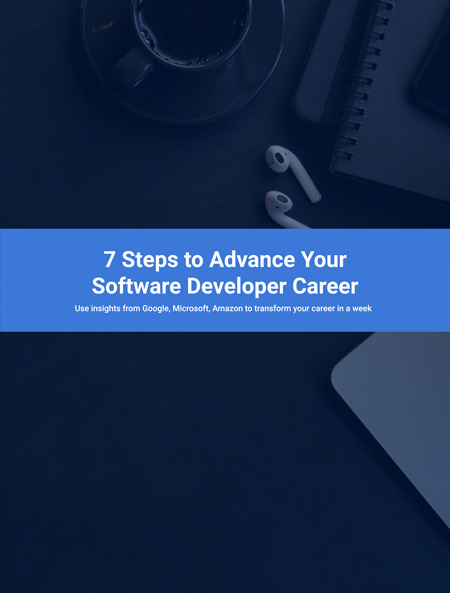
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.