How to add an item to the list if it doesn't exist in C#?
To add an item to the list, if it doesn't exist:
- Use the
Contains
method to check if the item exists in the list. It returns true if the item exists in the list. - If
Contains
is true, use theAdd
method to add the item to the list. - Create a generic extension method to make it easy to add an item to the list if it doesn't exist.
List<string> list = new List<string>() { "exists" };
list.AddIfNotExists("exists");
list.AddIfNotExists("doesn't exist");
Console.WriteLine(string.Join(", ", list)); // exists, doesn't exist
public static bool AddIfNotExists<T>(this List<T> list, T value)
{
if (!list.Contains(value))
{
list.Add(value);
return true;
}
return false;
}
Performance
The Contains
method does a linear, O(n) search. If you have a lot of items in the list, this can be slow.
To get a faster collection search, consider to:
- Use a hashset or a dictionary,
- BinarySearch method on the List
- Sorted List
In most cases, you won't run into performance problems. If you have a list with over 10,000 items, do some performance testing to find which method performs the best.
IEnumerable
To implement the "add if not exists" method using IEnumerable:
- Use
Contains
on the IEnumerable to check if the item exists in the list. - If
Contains
is false, use Append on the IEnumerable to add the item. - Use a new variable to store the returned value because
Append
creates a new collection.
public static IEnumerable<T> AddIfNotExists<T>(this IEnumerable<T> list, T value)
{
if (!list.Contains(value))
{
return list.Append(value);
}
return list;
}
We need to create a new variable because in C#, IEnumerable is an immutable collection. It means that we cannot add, modify, or remove items.
The Append
method does not change the elements in the collection, it just makes a copy of the collection with the extra element added.
The benefit of using the IEnumerable interface is that it's more general. The code will work with any type that implements IEnumerable, not just List.
For example, we could use the same code to add an item to a string list:
string[] words = { "apple", "banana", "carrot" };
IEnumerable<string> newWords = words.AddIfNotExists("celery")
.AddIfNotExists("apple")
.AddIfNotExists("banana");
Console.WriteLine(string.Join(", ", newWords)); // apple, banana, carrot, celery
HashSet
Consider using a HashSet to prevent duplicate values in a collection.
In C#, HashSet cannot have duplicate elements. The implementation takes care of it for you.
So, if you keep calling the Add
method with an existing item, nothing will happen:
HashSet<string> items = new ();
items.Add("1");
items.Add("1");
items.Add("1");
items.Add("2");
Console.WriteLine(string.Join(", ", items)); // 1, 2
However, unlike List, HashSet doesn't guarantee order of items.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →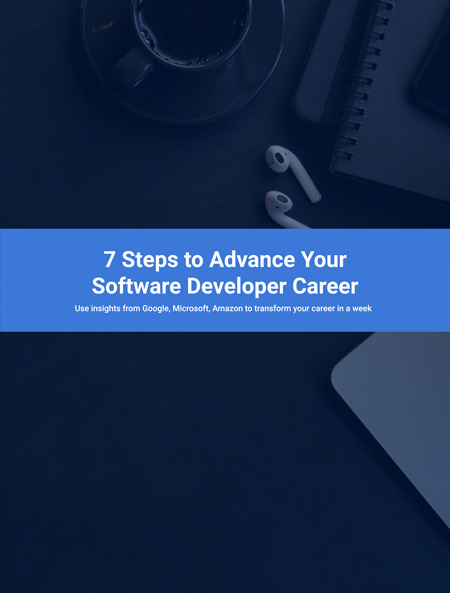
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.