C#: Convert String to Int
How to convert string to int in C#?
The top 3 ways to convert string to int in C# are:
1. TryParse
The best way to convert string to int in C# is to use the TryParse
method.
To convert string to int in C#:
- Pass the string as the first parameter to int.TryParse
- Pass the int reference as the second parameter using the out parameter modifier.
- Capture the boolean returned from int.TryParse.
- If int.TryParse returned
true
, the string to int conversion worked. - If int.TryParse returned
false
, the string to int conversion failed and the int value will be 0 (default).
Here's the full code showing successful string to int conversion:
string number = "505";
if (int.TryParse(number, out int result))
{
Console.WriteLine($"Conversion worked {result}"); // Conversion worked 505
}
else
{
Console.WriteLine($"Conversion failed {result}");
}
Here's the full code showing failed string to int conversion:
string number = "invalid";
if (int.TryParse(number, out int result))
{
Console.WriteLine($"Conversion worked {result}");
}
else
{
Console.WriteLine($"Conversion failed {result}"); // Conversion failed 0
}
2. Parse
The main difference between int.TryParse and int.Parse is that int.TryParse won't throw an exception if the passed string is in invalid numeric format. On the other hand, int.Parse throws exceptions that we need to catch.
If you want to use int.Parse, make sure your string is in valid format or add code to catch exceptions.
Here's a code example of successful use of int.Parse:
string number = "505";
int result = int.Parse(number);
Console.WriteLine($"Conversion worked {result}"); // Conversion worked 505
If the input string is not a valid string representation of a number, string to int conversion fails:
string number = "invalid";
int result = int.Parse(number);
Error: System.FormatException: Input string was not in a correct format. at System.Number.ThrowOverflowOrFormatException(ParsingStatus status, TypeCode type) at System.Int32.Parse(String s)
3. Convert
Another way to convert string to int is to use the Convert class:
string number = "505";
int result = Convert.ToInt32(number);
Console.WriteLine($"Conversion worked {result}"); // Conversion worked 505
The static method Convert.ToInt32
uses int.Parse
to convert string to int:
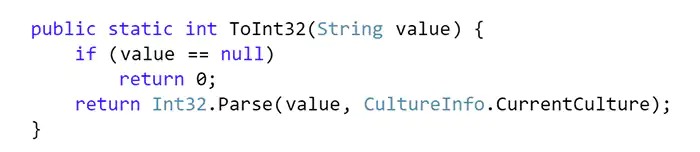
Underlying implementation of C# Convert.ToInt32.
The main benefit of using Convert class instead of calling int.Parse directly is that Convert has overloads for different data types. For example, Convert.ToInt32()
accepts both string and DateTime:
public static int ToInt32(String value) ;
public static int ToInt32(DateTime value);
So with Convert
we can keep our syntax consistent.
Supported types
Convert is a static class that exposes methods for converting a string to varios numeric data types:
Type | Convert Method |
---|---|
decimal | ToDecimal(String) |
float | ToSingle(String) |
double | ToDouble(String) |
short | ToInt16(String) |
int | ToInt32(String) |
long | ToInt64(String) |
ushort | ToUInt16(String) |
uint | ToUInt32(String) |
ulong | ToUInt64(String) |
ToInt32 can throw FormatException or OverflowException.
FAQ
What's the signature of int.TryParse method?
The signature of the int.TryParse is:
public static bool TryParse (string? s, out int result);
What is the Try-Parse Pattern?
Try-Parse Pattern is a design pattern for exception handling. Try-Parse method is expected not to throw an exception if the action is unsuccessful. Instead, it is supposed to return false.
The pattern is not restricted to converting strings to other types. It can be used for any action that may or may not succeed.
How does String to Int conversion work?
.NET uses the internal Number
class from the System
namespace to define logic that recognizes numbers in a string.
The most important method for converting String to Int is the ParseNumber
method:
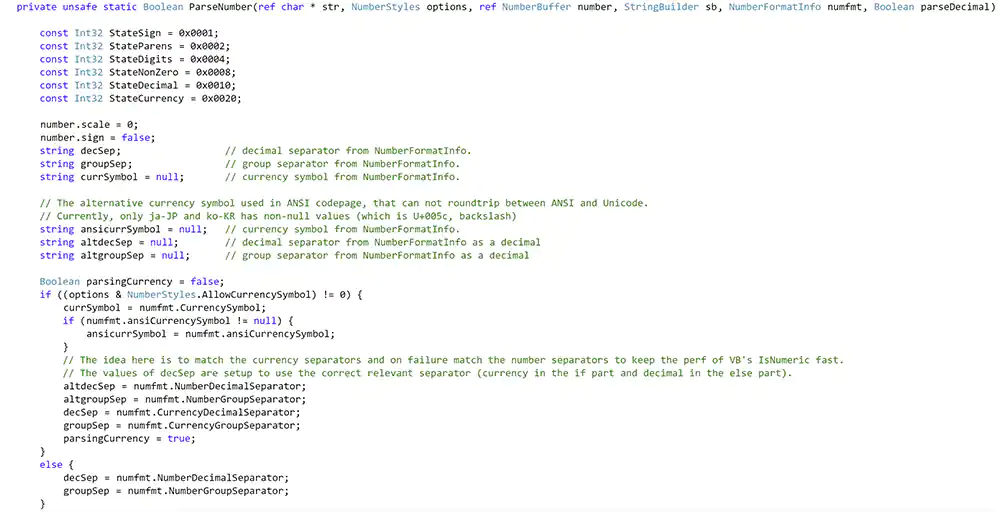
Underlying implementation of C# ParseNumber.
There are many gotchas when parsing an int. For example, leading spaces, trailing spaces, parentheses, currency symbols, and more.
ParseNumber
supports complex scenarios using NumberStyles. Depending on the style you select, the parser validates and converts the string.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →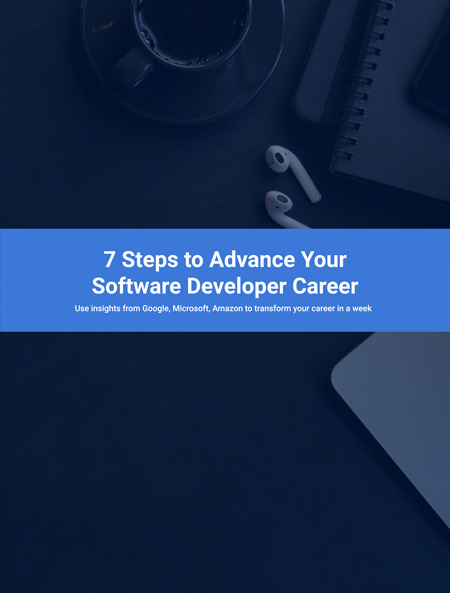
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.