C# TryParse: What is it? How to use it?
Exceptions are every developer's nightmare.
Wouldn't it be great if there was a way to avoid them altogether?
C# TryParse is a method that allows you to check the validity of a string before attempting to convert it into a specific type. It can save you from a lot of headaches down the road.
In this blog post, we'll take a look at what TryParse is and how to use it. It's an essential tool in any coder's toolkit, so it's worth taking the time to learn how to use it properly.
It definitely saved me a few times when I was starting out.
What is TryParse?
TryParse is .NET C# method that allows you to try and parse a string into a specified type.
It returns a boolean value indicating whether the conversion was successful or not.
If conversion succeeded, the method will return true and the converted value will be assigned to the output parameter.
If conversion failed, the return value will be false and the output parameter will not change value (default value).
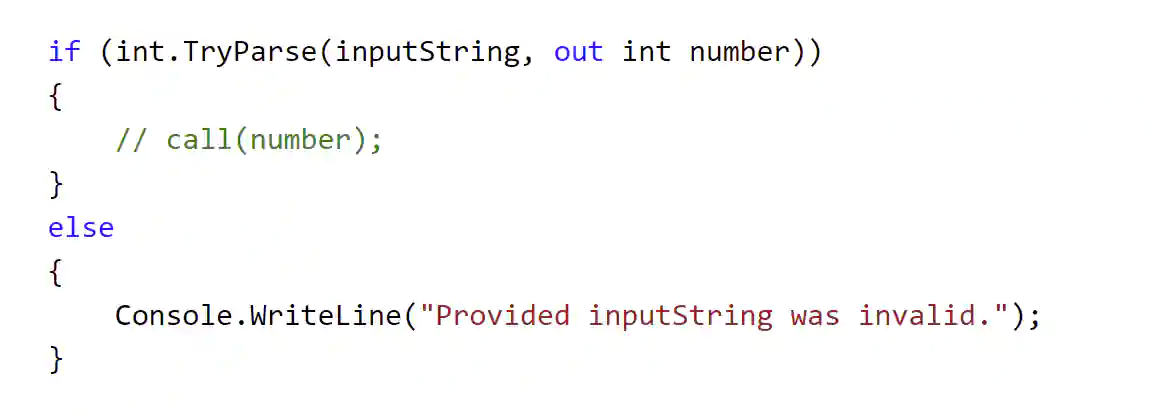
Example useage of TryParse in C#.
Out parameter
The out parameter is how the TryParse method passes the parsed value back to you.
It's a parameter of type T, where T is the type that you are trying to parse the string into.
Static method
TryParse is a static method, so it can be called without needing an instance of the class.
TryParse is used for many types:
If we look at the underlying .NET code, we can see that TryParse uses complex methods to figure out if value can be parsed to a given type.
This example shows code used to check if string value is a number:
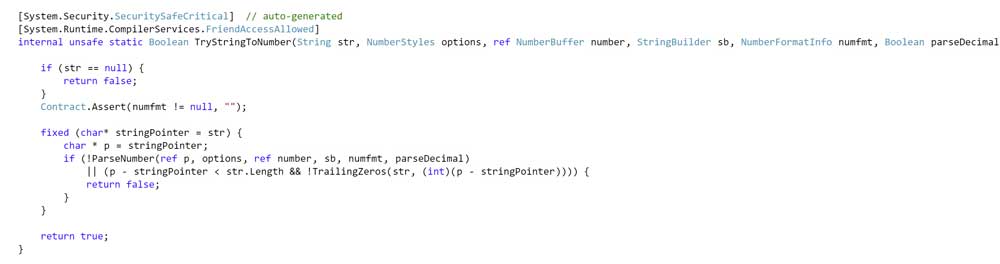
.NET source code for TryParse.
What's the difference between Parse and TryParse?
The main difference between Parse and TryParse is that Parse throws an exception if the string doesn't contain a valid number. The TryParse method returns true if the string contains a valid number without the exception.
System.FormatException: ‘Input string was not in a correct format.'
How to use TryParse?
You can use TryParse with different types, but the syntax is the same for all of them.
How to parse string to DateTime in C#?
To parse a string to DateTime in C#, you use the TryParse method and pass the string to be parsed and the type of DateTime you want it converted to.
If the conversion is successful, then a DateTime object will be assigned to the out parameter.
Here's an example:
string input = "1/1/2022";
DateTime.TryParse(str, out DateTiem date);
How to parse numeric string in C#?
To parse numeric string to a number in C#, use the following code:
string str = "5";
if (Int32.TryParse(str, out int num))
{
// .. rest of your code
}
The if-check is necessary because TryParse can return false even if the string contains a valid number. In that case, the num
above would default to 0, which could lead to some unexpected results.
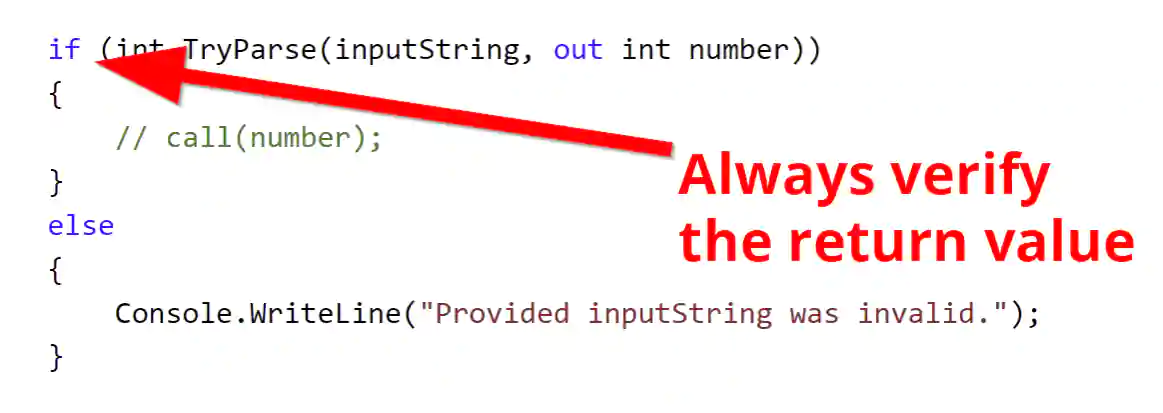
Use-cases
TryParse is often used in cases where you need to validate input from a user, or read data from a file.
For example, if you are reading data from a text file, you can use TryParse to check that the string represents a valid number.
I find myself mostly using int.TryParse. It's a go-to way to convert a string representation of number to an integer.
Should I use TryParse?
Yes, use TryParse in your code whenever you need to parse a string into a different type. It's a quick and easy way to validate input, without having to throw an exception if the conversion fails.
Just remember to always check if conversion failed by checking the return value of TryParse.
When was TryParse introduced to C#?
The TryParse method was introduced in the first version of .NET Framework. It has been a part of the language since then.
Format providers
If we look at the underlying .NET code, we'll see that TryParse has overloading method that takes in a format:
public static bool TryParse(String s, NumberStyles style, IFormatProvider provider, out SByte result) {
NumberFormatInfo.ValidateParseStyleInteger(style);
return TryParse(s, style, NumberFormatInfo.GetInstance(provider), out result);
}
The format providers are used to give more control over how the string is parsed based on different culture settings.
For example, you can use a specific format provider to parse a string into a DateTime using the Japanese culture:
DateTime.TryParse("2016/12/24", CultureInfo.CreateSpecificCulture("ja-JP"), out DateTime date);
The format provider is rarely used, but it's a good option to have if you need more control over how the string is parsed. Besides dates, it comes useful when parsing numbers in other languages, with different number separators and so on.
Try-Parse Pattern
Try-Parse Pattern is a design pattern for exception handling. Try-Parse method is expected not to throw an exception if the action is unsuccessful. Instead, it is supposed to return false.
The pattern is not restricted to converting strings to other types. It can be used for any action that may or may not succeed.
Conclusion
TryParse is a useful .NET method that allows you to try and parse a string into a specified type. It's very useful when you need to parse a string representation of a number into an integer.
When converted successfully, the method's outputs the numeric value as an out parameter, and the method returns true if the conversion was successful.
If the string can't be parsed, then an exception is thrown and the method returns false.
You should use TryParse in your code whenever you need to parse a string into a different type. It's a quick and easy way to validate input, without having to throw an exception if the conversion fails. Your code will be more robust and it will be easier to maintain.
It also has overloading methods that take in a format, which gives you more control over how the string is parsed.
If you end up creating your own parsing implementation, it's a good practice to use the Try-Parse design pattern. This will help you avoid throwing exceptions in your code.
Examples
using System;
public class Program
{
public static void Main()
{
string s = "10.0";
int.TryParse(s, out int value);
Console.WriteLine(value); // 0
decimal.TryParse(s, out decimal decimalValue);
Console.WriteLine(decimalValue); // 10.0
}
}
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →- What is TryParse?
- Out parameter
- Static method
- What's the difference between Parse and TryParse?
- How to use TryParse?
- How to parse string to DateTime in C#?
- How to parse numeric string in C#?
- Use-cases
- Should I use TryParse?
- When was TryParse introduced to C#?
- Format providers
- Try-Parse Pattern
- Conclusion
- Examples
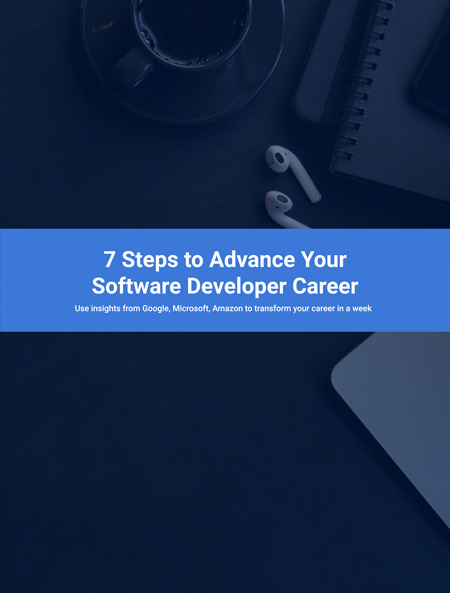
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.