C#: How to enumerate an enum?
Enumerate an enum in C#
To enumerate an enum in C#:
- Use the Enum.GetValues method.
- Provide the enum type in the angle brackets.
- Enum.GetValue returns an array of the values of the specified enumeration type.
- Loop through the array to get enum's keys and values.
For example:
public enum Country
{
Unknown,
Canada,
UnitedStates
}
foreach(var country in Enum.GetValues<Country>())
{
Console.WriteLine(country.ToString() + ' ' + (int)country);
}
// Output:
// Unknown 0
// Canada 1
// UnitedStates 2
We cast the enum to int to get the underlying integer value.
GetValues<TEnum>()
is only available in .NET version 5 and newer.
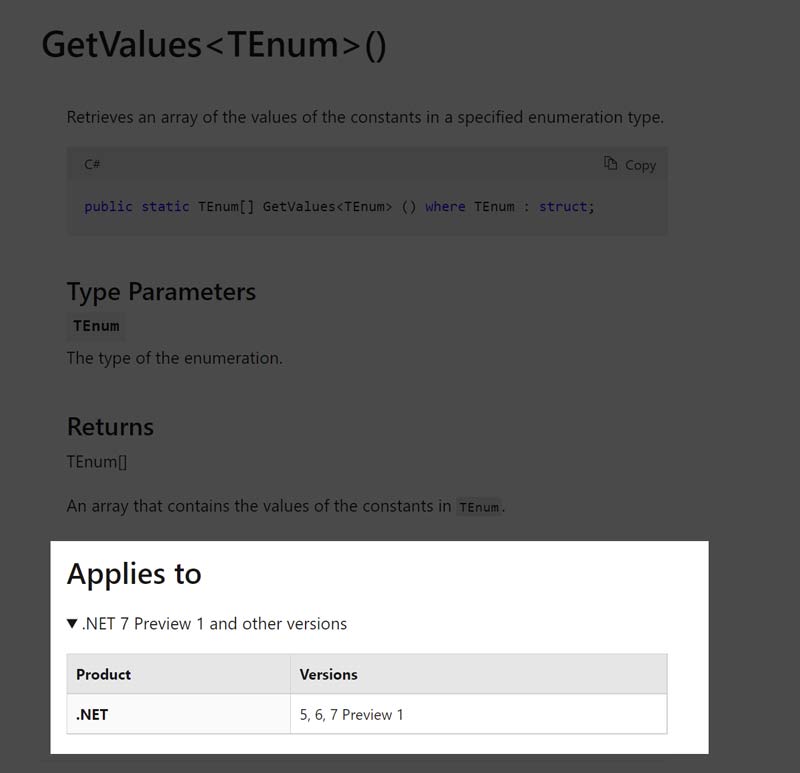
Enumerate an enum prior .NET 5
To enumerate an enum in .NET versions before version 5:
- Use the Enum.GetValues(Type) static method. Pass the enum type as the first parameter.
- Enum.GetValues(Type) returns an array of values of constants in the enum.
- Loop through the array to get underlying values.
- Cast the underlying object to appropriate integral value.
This example shows how to enumerate an enum using Enum.GetValues(Type):
foreach(var country in Enum.GetValues(typeof(Country)))
{
Console.WriteLine(country.ToString() + ' ' + (int)country);
}
A disadvantage of this approach is that Enum.GetValues can throw ArgumentException if the provided type is not an enum.
Loop through names
To loop through enum names, we can use Enum.GetNames method:
foreach(Country country in Enum.GetNames<Country>())
{
Console.WriteLine(country);
}
As mentioned in the C# book C# 9.0 in a Nutshell, CLR implements GetValues and GetNames by reflecting over the fields in the enum’s type.
Enum.GetNames returns an array of names for the given enum type.
Composite enum members
Enumerating using Enum.GetValues also works for composite members. Composite enum members consist of multiple enum members combined using a logic operator.
For example:
public enum Location
{
Unknown,
Canada,
UnitedStates,
NorthAmerica = Canada | UnitedStates,
}
foreach(Location location in Enum.GetValues<Location>())
{
Console.WriteLine(location.ToString());
}
// Output:
// Unknown
// Canada
// UnitedStates
// NorthAmerica
LINQ
Another method to enumerate an enum is to use LINQ:
var locations = (Location[]) Enum.GetValues(typeof(Location));
var withValues = locations.Select(location => new { Value = (int)location, Name = location.ToString() });
Console.WriteLine(withValues.ElementAt(1)); // { Value = 1, Name = Canada }
Performance
To improve the performance of looping through enum values, explicitly cast the type. When we cast the enum explicitly, we avoid the use of IEnumerator
to iterate over the returned Array.
Benchmarks show 1.6x better performance when we cast the returning type when looping over an enum.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →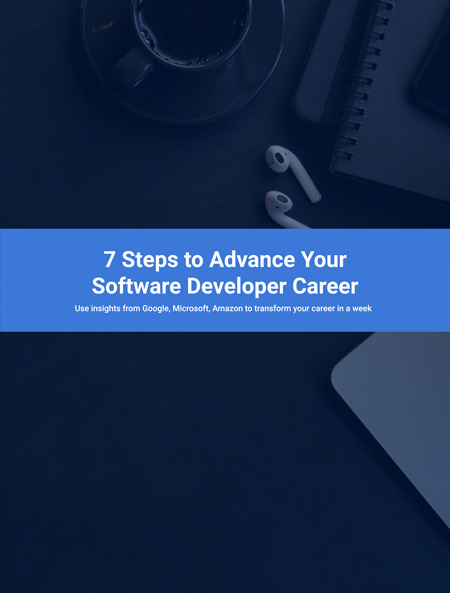
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.