How to use get and set accessors in C#?
Key Points
- C# Get and Set accessors are shorthand for defining a property with both a getter and a setter method.
- Property initializers let us define the default value for automatic properties.
- To make an auto property read-only, omit the set modifier from the accessor definition.
How to use get and set accessors in C#?
To use get and set accessors in C#:
- Define the property accessor type and name. For example,
public int Age
. - Add the
get
accessor body. The program executes theget
body when we ready the property. - (optional) Add the
set
accessor body. When we change the property, it executes the set body.
The third step is optional because we can have a read-only property.
public class Person
{
private int _age;
public int Age
{
get
{
return _age;
}
set
{
_age = value;
}
}
}
Person person = new ();
Console.WriteLine(person.Age); // 0
person.Age = 32;
Console.WriteLine(person.Age); // 32
What does { get; set; }
syntax mean in C#?
In C# classes it's very common to see { get; set; }
code.
The { get; set; }
syntax in C# is a shorthand for the automatic property.
The { get; set; }
syntax in C# is a shorthand way of defining a property with both a getter and a setter method. It allows you to define a property without explicitly declaring the getter and setter methods.
This syntax doesn't explicitly define bodies for get and set accessors:
public class Person
{
public int Age {get; set;}
}
Instead, it provides a default implementation. This code is logically equivalent to:
public class Person
{
private int _age;
public int Age
{
get
{
return _age;
}
set
{
_age = value;
}
}
}
The only difference between using the "get set syntax" and explicit private variable implementation is that with automatic properties we cannot access the private variable.
With the "get set syntax", the compiler takes care of the implementation:
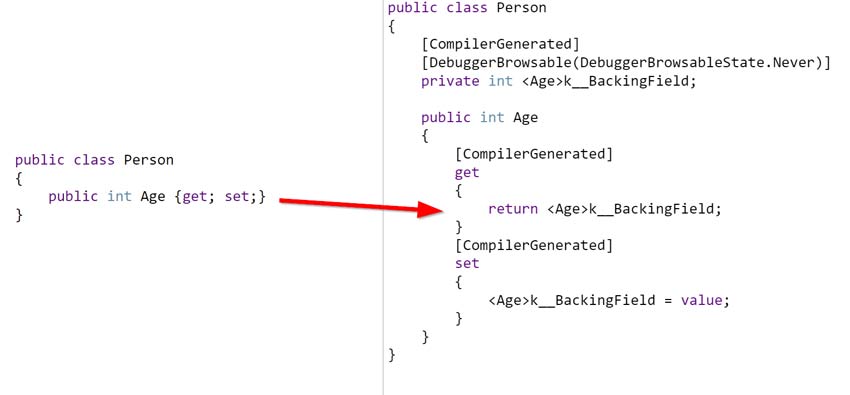
Property initializers
C# 6.0 introduced property initializers. Property initializers let us define the default value for the automatic properties.
To use property initializers in C#:
- Define the property accessor type and name.
- Use the
{ get; set; }
syntax. - Pass the default value using the equal operator
=
;
public int Age { get; set; } = 10;
The default value of the property above is 10.
How to make the automatic property Read-only?
To make the automatic property read-only, we omit the set
modifier:
public int Age { get; } = 10;
In the code above, the Age property is now read-only.
We can only access it in the constructor:
public class Person
{
public int Age { get; } = 10;
public Person(int age)
{
Age = age; // this works!
}
public void SetAge(int age)
{
Age = age; // won't work! Property or indexer 'Person.Age' cannot be assigned to -- it is read only
}
}
Why do we use properties in C#?
1. Data validation
The main use of properties is data validation. For example, we can add logic that prevents setting the age property to a negative value:
public int Age
{
get
{
return _age;
}
set
{
if (value < 0)
throw new ArgumentException("Age cannot be a negative number.")
_age = value;
}
}
2. Encapsulation
We use the private variable to hold the value locally. It provides encapsulation and lets us build the internal implementation without affecting outside code.
3. Raising events
Another use of get and set accessors in C# is raising events. We often want to perform action based on change. For example, "if order status changes to shipped, send an email". Set accessors are a great place to raise those events because they are the only point where object can change. So you don't have to duplicate the code by having it just before the setter call.
FAQ
What is encapsulation in C#?
In C#, encapsulation is a design principal that separates the external behavior from the internal implementation. We use encapsulation to group related logic in a meaningful way. For example, we can group customer information in a Customer
object. That object can store information that we don't want other areas of code to access, for example, password. So, we use a combination of accessor properties and modifiers to define the level of encapsulation. It defines how accessible a piece of information should be.
Do private variables need to have underscore in C#?
Private variables in C# don't have to be named with underscore. Adding an underscore to the name of a private variable is a naming convention, but not a requirement. Your code will run without errors even if you don't use the underscore prefix.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
- How to use get and set accessors in C#?
- What does { get; set; } syntax mean in C#?
- Property initializers
- How to make the automatic property Read-only?
- Why do we use properties in C#?
- 1. Data validation
- 2. Encapsulation
- 3. Raising events
- FAQ
- What is encapsulation in C#?
- Do private variables need to have underscore in C#?
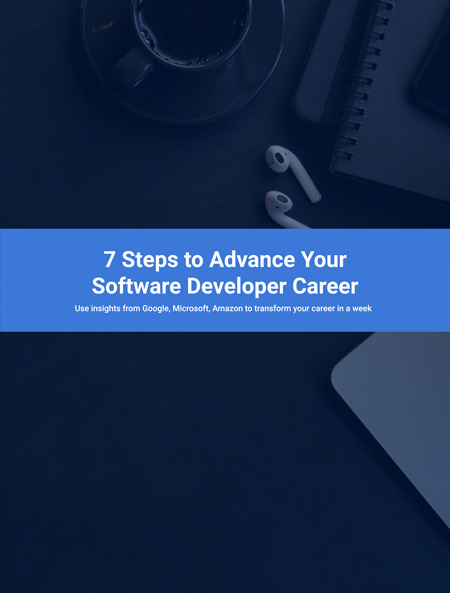
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.