How to get time from DateTime in C#?
There are three ways to get time form DateTime in C#:
- Using TimeSpan - Best way for code cleaness.
- Using DateTime - Best if you need to use DateTime type.
- Using string format - Best way for displaying in an interface.
How to get time from DateTime in C#?
The best way to get time from DateTime in C# is to:
- Access the
TimeOfDay
property ofDateTime
to getTimeSpan
value for yourDateTime
. - Access the
Hours
property ofTimeSpan
to get the hour of theDateTime
. - Access the
Minutes
property ofTimeSpan
to get the minutes of theDateTime
. - Access the
Seconds
property ofTimeSpan
to get the seconds of theDateTime
.
The code below shows how to get time from DateTime:
TimeSpan currentTime = DateTime.Now.TimeOfDay;
Console.WriteLine(currentTime); // 19:10:30.1729426
Console.WriteLine(currentTime.Hours); // 19
Console.WriteLine(currentTime.Minutes); // 10
Console.WriteLine(currentTime.Seconds); // 30
TimeOfDay property
The source code of the DateTime struct shows how the TimeOfDay
property is calculated:
// Returns the time-of-day part of this DateTime. The returned value
// is a TimeSpan that indicates the time elapsed since midnight.
//
public TimeSpan TimeOfDay {
get {
return new TimeSpan(InternalTicks % TicksPerDay);
}
}
What is TimeSpan?
TimeSpan data type represents time of the day.
The key properties for getting time using the TimeSpan type are:
- Hours - Gets the hours component of the time interval. From 0 to 23.
- Minutes - Gets the minutes component of the time interval.
- Seconds - Gets the seconds component of the time interval.
See the source code of TimeSpan for more details
Get time from DateTime using DateTime type
Alternative for getting time from DateTime is to use the DateTime type with date of 1/1/0001.
The idea is to ignore the date portion of the time.
The code below shows how to get time from current DateTime:
DateTime timeOnly = new DateTime(DateTime.Now.TimeOfDay.Ticks);
Console.WriteLine(timeOnly); // 1/1/0001 7:10:30 PM
We can still access the hour, minutes, and seconds properties, but the intent is not as clear as using TimeSpan.
Get time from DateTime using ToString method
Another way to get only time from DateTime is to use ToString
method:
DateTime currentTime = DateTime.Parse("6/19/2022 12:34:12 AM");
Console.WriteLine(currentTime.ToString("HH:mm")); // 00:34
Console.WriteLine(currentTime.ToString("hh:mm tt")); // 12:34 AM
Console.WriteLine(currentTime.ToString("H:mm")); // 0:34
Console.WriteLine(currentTime.ToString("h:mm tt")); // 12:34 AM
This method is the best way of getting time from DateTime if your main goal is to display time in an interface because it gives you the most flexibility in choosing a human-readable format.
FAQ
What are some ways to represent time in .NET?
The System
namespace in .NET has three immutable structs to represent time:
- DateTime
- DateTimeOffset
- TImeSpan
What are Ticks in C#?
In C#, tick is the value of 100-nanosecond intervals since 1/1/0001 12:00am.
There are 10,000,000 ticks per second. With so many ticks per second, the underlying data structure needs to be very large. .NET uses UInt64 value type with values ranging from 0 to 18,446,744,073,709,551,615.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →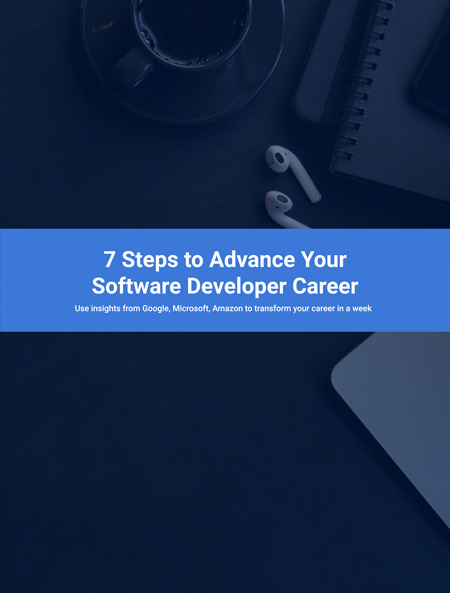
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.