How to generate a Guid in C#?
C#: Guid Struct
In C#, the Guid struct is the built-in type from the System
namespace that represents a globally unique identifier.
Guid consists of a 128-bit integer value that aims to always be unique in a given system. In fact, the number of possible unique combinations is 2128, or 3.4 x 1038. So it's extremely unlikely that you'll ever generate the same Guid value.
How to generate a unique Guid?
To generate a unique Guid in C#, use the static Guid.NewGuid
method:
Console.WriteLine(Guid.NewGuid()); // 4e19c13d-cab0-467b-8776-423eaee61f2a
In the example above, we generate a new Guid and print it as a string. When represented as a string, a Guid is formatted as a 32-digit hexadecimal number, with optional hyphens after the 8th, 12th, 16th, and 20th digits. This format is known as the standard format and it's represented as "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx" where x is a hexadecimal digit.
When should I use Guids in C#?
In C#, globally unique identifiers (Guids) are often used as keys in databases or as unique identifiers for resources in distributed systems.
Here are some common scenarios where you might use Guids in C#:
- Primary key in a database: Extremly likely to be unique which makes them ideal for use as keys in a distributed database where multiple devices may be adding records concurrently.
- Unique identifier for resources in a distributed system: Makes it to easy identify resources across different services.
- Unique identifier for files: Guids can be used as unique identifiers for files rather than timestamps to prevent concurrent writes to the same file.
- COM (Component Object Model): Guids are used to identify and locate the component.
How to generate an empty Guid?
Since Guid is a struct, it cannot be null (unless wrapped in nullable). Instead, a common way to represent an empty Guid is to use all zeros.
To generate an empty Guid, use the static Guid.Empty
property.
Console.WriteLine(Guid.Empty); // 00000000-0000-0000-0000-000000000000
Â
How to generate a Guid from byte array?
To generate a Guid from byte array, follow these steps:
- Use the
Guid(byte[]
constructor. - Make sure the length of the byte array is 16, otherwise, it will throw an exception.
- Ensure the byte array in a specific order: the first 4 bytes represent the first integer, the next 2 bytes represent the first short, the next 2 bytes represent the second short, and so on.
Full example:
byte[] bytes = new byte[] { 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F, 0x10 };
Guid guid = new Guid(bytes);
Console.WriteLine(guid); // 04030201-0605-0807-090a-0b0c0d0e0f10
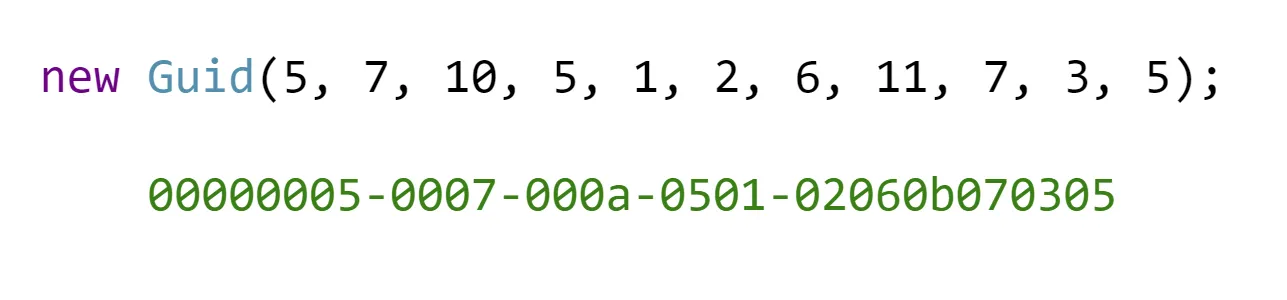
How to parse a string into Guid?
In C#, you can use the Guid(string s)
constructor to parse a string into a Guid struct. The constructor accepts a string that represents a valid Guid and creates a Guid struct that contains the parsed value.
Here is an example of how to use the Guid(string s)
constructor:
string guidString = "f06c3c8d-b2c2-4cc6-9a1a-8b3b3c82b9f0";
Guid guid = new Guid(guidString);
The Guid(string s) constructors throws a FormatException
if the string is not in the correct format.
To avoid this exception, you can use the TryParse()
method, which returns a boolean indicating whether the string was successfully parsed.
string guidString = "f06c3c8d-b2c2-4cc6-9a1a-8b3b3c82b9f0";
if (Guid.TryParse(guidString, out Guid guid))
{
// use the Guid
}
else
{
// handle error
}
Supported Guid styles
The string representation of a Guid can be represented in many different ways (with brackets, hyphens, parenthesis, etc.)
If you have a specific type you want to parse, Guid struct exposes a static method TryParseGuid(String g, GuidStyles flags, ref GuidResult result)
that accepts a GuidStyles
flags enumeration of GuidStyles
that specifies the format of the Guid string. This parameter allows you to specify whether the string should contain hyphens, brackets, or braces and whether it should have a specific format such as hexadecimal or digit format.
The table below describes all the Guid styles that can be parsed in C#/.NET:
Guid Format | Description |
---|---|
None | No specific format is required. |
AllowParenthesis | Allows the Guid to be enclosed in parentheses. |
AllowBraces | Allows the Guid to be enclosed in braces. |
AllowDashes | Allows the Guid to contain dash group separators. |
AllowHexPrefix | Allows the Guid to contain 221. |
RequireParenthesis | Requires the Guid to be enclosed in parentheses. |
RequireBraces | Requires the Guid to be enclosed in braces. |
RequireDashes | Requires the Guid to contain dash group separators. |
RequireHexPrefix | Requires the Guid to contain 221. |
HexFormat | Requires the Guid to be enclosed in braces and contain 221. |
NumberFormat | No specific format is required. |
DigitFormat | Requires the Guid to contain dash group separators. |
BraceFormat | Requires the Guid to be enclosed in braces and contain dash group separators. |
ParenthesisFormat | Requires the Guid to be enclosed in parentheses and contain dash group separators. |
Any | Allows any format: parentheses, braces, dashes, and hex prefix. |
How does C#/.NET generate Guids?
Windows
On Windows, C#'s Guid.NewGuid()
method generates a new globally unique identifier (GUID) using the CoCreateGuid
Windows API function.
CoCreateGuid
is a C++ function imported from the Windows OLE32 DLL that creates a 128-bit integer that is guaranteed to be unique across all devices and in all time. This makes it useful for creating persistent identifiers in distributed systems. Moreover, it uses the UuidCreate function to generate the new Guid, which ensures that the Guid generated can not be traced to the ethernet address of the computer on which it was generated and also it cannot be associated with other Guids created on the same computer.
Unix
On UNIX-based systems, the Guid.NewGuid()
method uses a different approach to generate a new globally unique identifier. The method uses the Interop.GetRandomBytes()
method to generate a random byte array of the same size as the Guid struct. Then it converts this byte array to the struct and modifies the bits indicating the type of the GUID.
This method ensures that the Guid generated is unique and random, but it does not use the ethernet address or any other unique hardware identifier, so it might not be as unique as the method used on Windows.
Are Guids random in C#/.NET?
C# Guid generation cannot be considered random on the Windows platform because the Guid.NewGuid()
method generates globally unique identifiers (GUIDs) using the CoCreateGuid
C++ function that keeps persistent identifiers across environments. In other words, it tries to create a unique value (not necessarily random).
On UNIX-based systems, the Guid.NewGuid() generates a random byte array of the same size as the Guid struct. Because of that, Guids can be considered random in .NET applications running on UNIX-based systems.
C# Guid Summary
The table below summarizes key points about Guid struct in C#:
Guid Key Point | Description |
---|---|
Definition | A globally unique identifier (GUID) is a 128-bit integer value that is guaranteed to be unique across all devices and in all time |
Size | 128-bit integer value. |
Format | When represented as a string, a |
Generation | The Guid struct provides a |
Use cases | Commonly used as keys in databases or as unique identifiers for resources in distributed systems, user sessions, or files. |
Randomness | The probability of having a collision is extremely low, but it's still possible. The best practice is to ensure that Guids are unique in the context of the system they're used in. |
Comparison | Guids can be compared using the equal operator (==) or the not equal operator (!=) |
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →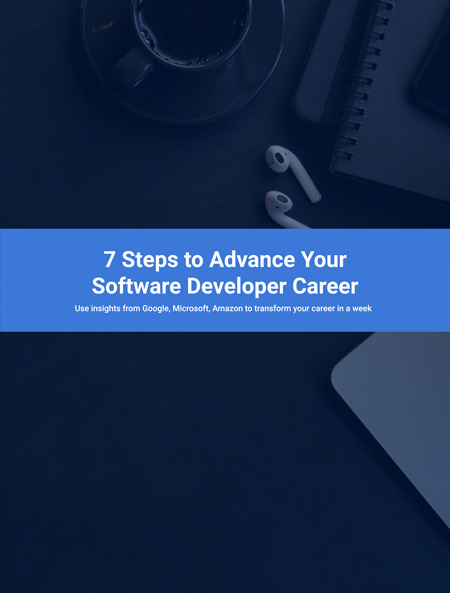
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.