How to use one-line if-else in C#?
How to use one-line if-else in C#?
To use a one-line if-else statement in C#, follow this syntax:
condition ? true_expression : false_expression;
int result = isTrue ? 1 : 0;
This is equivalent to the following if-else statement:
int result;
if (isTrue)
{
result = 1;
}
else
{
result = 0;
}
Ternary Operator
A one-line if-else statement is also called the ternary operator in C#.
It's called ternary because it has three operands:
- the condition,
- the expression that is evaluated if the condition is true, and
- the expression that is evaluated if the condition is false.
The ternary operator is a useful feature in C# that can help you write more concise and readable code in a variety of scenarios.
However, it's important to avoid too many condition chains to prevent your code from being overly complex or hard to read.
When to use the one-line if-else?
You can use one-line if-else statements in C# to write more concise code for simple conditional operations. For example, setting the value of a variable based on a single condition. However, for more complex scenarios, you should use a regular if-else statement or a switch statement to make your code more readable and maintainable.
Examples
Here are some examples of one-line if-else statements (ternary operators) in C#:
Null-Check
In this example we use IsNullOrEmpty to conditionally set the message
variable:
string message = !string.IsNullOrEmpty(name) ? "Hello, " + name : "Hello, stranger";
Method Invocation
int value = 10;
bool isPositive = value > 0 ? IsPositive(value) : false;
Throwing Exceptions
You can also throw an exception in C# using a one-line if-else statement:
int value = -1;
string message = value >= 0 ? "Value is positive" : throw new ArgumentException("Value must be non-negative");
Conditions chaining
You can also chain multiple conditions together using nested ternary operators:
int value = 5;
string message = value < 0 ? "Value is negative" : value == 0 ? "Value is zero" : "Value is positive";
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →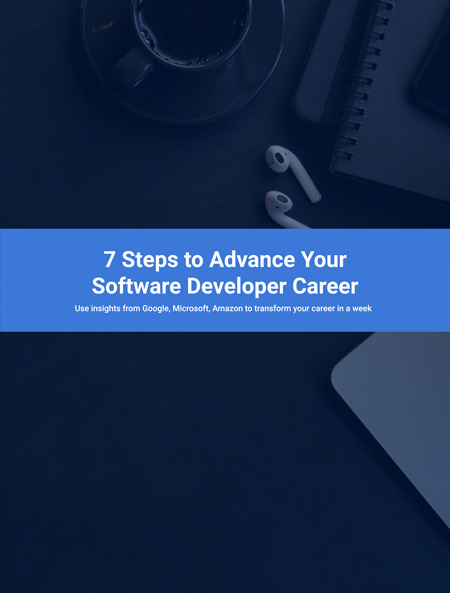
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.