C# Timer: Everything you need to know
Timers are very useful in C# for UI programming, games, and applications have logic based on time intervals.
Timers that C# has available through .NET make it easy to time intervals and also to perform specific tasks at specific intervals.
The C# Timer class is a .NET class that helps you create an event that will happen at a set interval. The interface makes it easy to start and stop a timer or enable and disable it.
Timer Usage
The key terms when using the timer class are:
- Interval: Time interval between two successive invocations of Elapsed function.
- Callback: The timer invokes this method at the end.
- AutoReset: Boolean that determines whether the timer will raise the Tick event each time the specified interval has elapsed.
To use a timer in C#, follow these steps:
Setup the timer with your desired interval in milliseconds.
C#System.Timers.Timer timer = new (interval: 1000);
Define the
Elapsed
event handler. The timer will call this handler every time the interval milliseconds have elapsed.C#void HandleTimer() { Console.WriteLine("Interval Called"); }
Setup the timer to call your event handler:
C#timer.Elapsed += ( sender, e ) => HandleTimer();
Call the Start() method to start the timer.
C#timer.Start();
Once done with the timer, call the Dispose() method to free up the resources used by the Timer.
C#timer.Dispose();
Putting it all together:
using System;
public class Program
{
public static void Main()
{
void HandleTimer()
{
Console.WriteLine("Interval Called");
}
System.Timers.Timer timer = new (interval: 1000 );
timer.Elapsed += ( sender, e ) => HandleTimer();
timer.Start();
Console.ReadLine(); // To make sure the console app keeps running.
System.Threading.Thread.Sleep(10000);
timer.Dispose();
}
}
Plus equal event
The += (plus equal) syntax in C# is a shortcut for defining an event handler. It tells the timer to subscribe to the event handler.
We can also use -= (minus equal) to unsubscribe if needed.
Interval limitation
The C# Timer depends on the system clock.
The resolution of the system clock is how often the Elapsed event will fire. If you set the Interval property to be less than resolving the system clock, then it won't fire at the desired interval.
For most use cases that won't be a problem. But if you are looking for high-resolution timer, then consider using the Stopwatch class.
Disposing
Timer class implements System.IDisposable interface to release the system resources allocated by it when disposed.
Best practices when working in C# say that you should clean up your resources if the class implements IDisposable interface.
There are two ways to dispose the Timer:
- Dispose the timer by calling the Dispose() method inside a try/catch block to avoid exception.
- Use the
using
directive to automatically call the Dispose method when a particular scope is clean up. This will avoid the extra try/catch block and code lines to clean up properly.
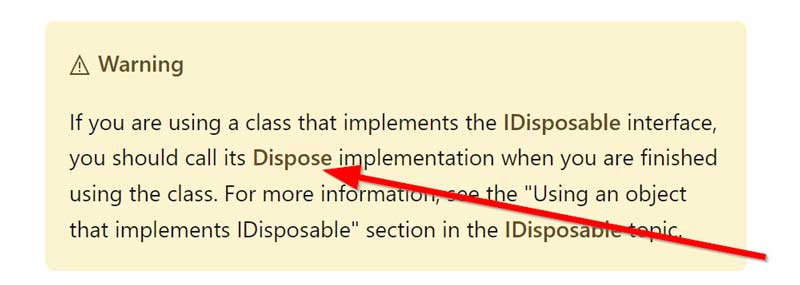
Async timer
The System.Timers.Timer class support async event handler to fire the event at the desired time interval. To define an async event handler, use the async
modifier before your signature for Elapsed event handler.
Example:
using System;
using System.Threading.Tasks;
Task HandleTimerAsync()
{
Console.WriteLine("Interval Called");
// Your async logic here.
return Task.CompletedTask;
}
System.Timers.Timer timer = new (interval: 1000 );
timer.Elapsed += async ( sender, e ) => await HandleTimerAsync();
timer.Start();
System.Console.WriteLine("Start");
System.Threading.Thread.Sleep(5000);
Keep in mind that the async callback must return a Task. If the async method runs longer than the interval, you might have some unexpected results.
→ Read more: C# Async vs sync
Different timers in .NET
In .NET, there are 4 different timers depending on the way you want to use them:
- System.Windows.Forms.Timer
- The System.Web.UI.Timer
- System.Timers.Timer
- System.Threading.Timer
System.Windows.Forms.Timer
The System.Windows.Forms.Timer class is specifically designed for rich client user interfaces.
Programmers can drag it into a form as a nonvisual control and regulate the behavior from within the Properties window. It will always safely fire an event from a thread that can interact with the user interface.
System.Web.UI.Timer
The System.Web.UI.Timer can perform Web page post backs at a defined interval, either asynchronously or synchronously. It's part of the ASP.NET framework, so we can use it only in ASP.NET Web applications.
This timer is useful for creating a real-time display of information on an ASP.NET application.
System.Threading.Timer
The System.Threading.Timer provides the ability to schedule a single callback on a background thread, either asynchronously or synchronously.
System.Threading.Timer is thread-safe.
System.Timers.Timer
System.Timers.Timer is the best way to implement a timer in C#.
System.Timers.Timer is a wrapper for System.Threading.Timer, abstracting and layering on functionality.
You cannot use System.Threading.Timer as a component within a component container, something that implements System.ComponentModel.IContainer, because it does not derive from System.ComponentModel.Component.
System.Timers.Timer extends the capabilities of the System.Timers.Timer to include features necessary for component container applications.
System.Timers.Timer and System.Threading.Timer are both designed for use in server-type processes, but System.Timers.Timer includes a synchronization object to allow it to interact with the user interface, which is helpful for applications that need to keep track of user input or updates.
When to use which timer?
Follow these guidelines to choose which one to use:
- System.Windows.Forms.Timer - For Windows Forms application, runs the delegate on a form's UI thread.
- System.Web.UI.Timer - For ASP.NET component. It allows you to perform asynchronous or synchronous web page post backs at regular intervals.
- System.Threading.Timer - great for background tasks on a thread pool.
- System.Timers.Timer - wraps the System.Threading.Timer with the simpler API. We use this one most of the time.
C# Timer Accuracy
The C# Timer is not accurate. The timer, on average, takes 9.07% longer than it is supposed to, according to research from Lawrence Technological University.
To get accurate time, we need to use the .NET StopWatch class.
System.Diagnostics.Stopwatch
The stopwatch class is used to time code execution. It can be started, stopped/paused, and reset.
The main difference between C# Stopwatch and C# Timers is that while the Timer triggers an event when a certain interval has elapsed, Stopwatch calculates how much time has passed since it started. Moreover, the Stopwatch is much more accurate and can measure time to a greater level of precision.
If you want a high-resolution timer, consider using the Stopwatch instead of the Timer.
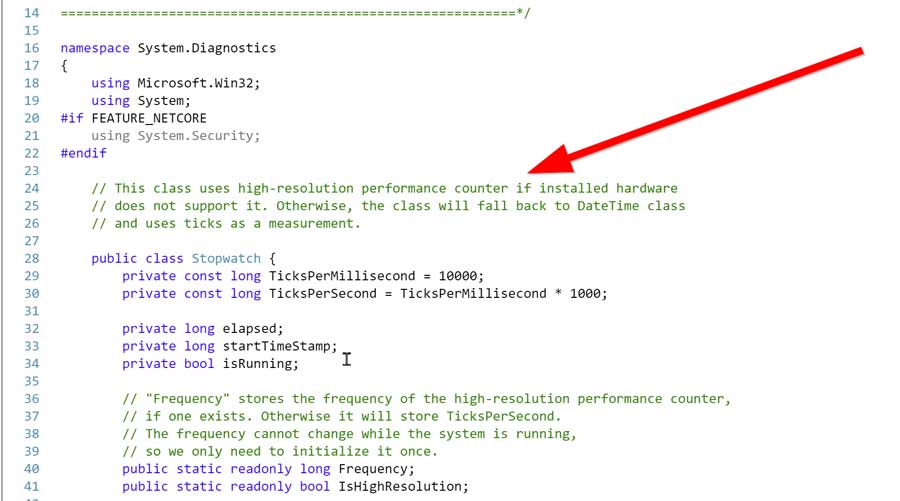
EventHandler vs ElapsedEventHandler
The main difference between EventHandler and ElapsedEventHandler is that EventHandler is more generic and ElapsedEventHandler works with a delegate that has a specific signature.
ElapsedEventHandler is a delegate that passes ElapsedEventArgs which extends EventHandler's arguments by adding FileTime
and SignalTime
. The added logic makes the ElapsedEventHandler delegate compatible with ElapsedEventArgs.
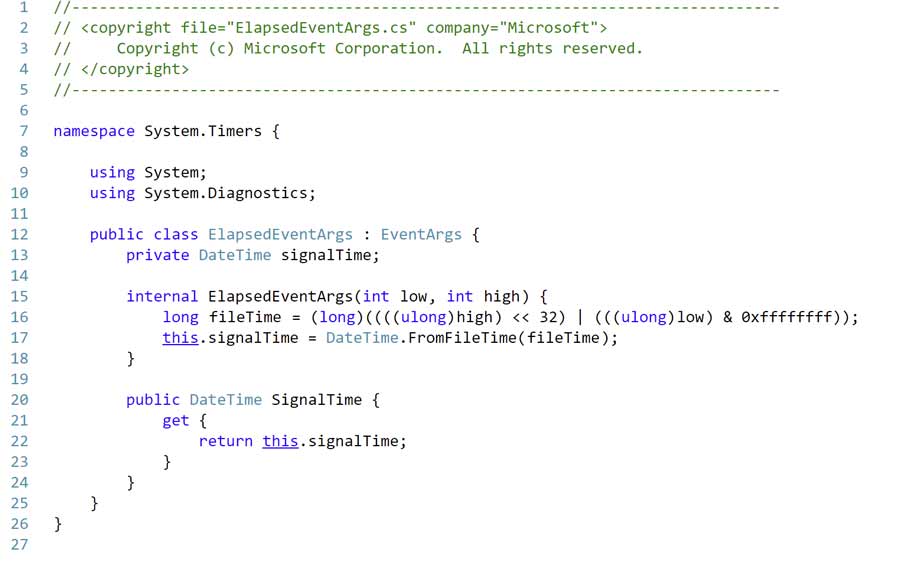
FAQ
What is an event in C#?
An event in C# is a way for a class to let clients know when something interesting happens with an object. We declare events using delegates.
Terms subscribe and unsubscribe are sometimes used to show that a class is interested in a particular event and wants to know when it occurs.
We often don't need event arguments, so we can implement that as an optional argument in the event handler.
How to name events in C#?
The naming convention suggest naming events in the past tense. For example, OrderPlaced, OrderShipped, etc.
The convention also suggest naming event handler as a combination of event name and EventHandler
suffix. For the events above, the event handler would be named OrderPlacedEventHandler
, OrderShippedEventHandler
.
Conclusion
Timers are a great way to handle time-based operations in C#. They can be used for everything from UI programming to game logic and more.
The timer class is easy to use, and makes it simple to set up an event that will happen at a specific interval.
In order to use a timer in C#, you must first setup the timer with your desired interval and then define the Elapsed event handler. Once the timer is started, it will call the event handler every time the specified interval has elapsed.
If you need a high-resolution timer, consider using the Stopwatch class. When you're done using the timer, be sure to dispose of it properly by calling the Dispose() method.
The .NET provides a variety of timers to suit your needs, depending on how you want to use them. System.Windows.Forms.Timer is specifically designed for rich client user interfaces, and can be regulated from within the Properties window. System.Web.UI.Timer can be used in ASP.NET Web applications to create real-time displays of information, while System.Threading.Timer is great for background tasks on a thread pool.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →Last modified on:
- Timer Usage
- Plus equal event
- Interval limitation
- Disposing
- Async timer
- Different timers in .NET
- System.Windows.Forms.Timer
- System.Web.UI.Timer
- System.Threading.Timer
- System.Timers.Timer
- When to use which timer?
- C# Timer Accuracy
- System.Diagnostics.Stopwatch
- EventHandler vs ElapsedEventHandler
- FAQ
- What is an event in C#?
- How to name events in C#?
- Conclusion
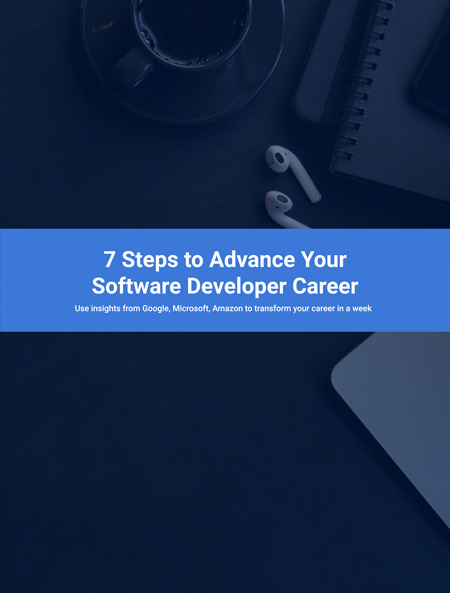
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.