Difference between VAR and TYPE in C#
VAR vs Type
The main difference between VAR and type in C# is that the var
keyword defines an implicitly typed local
variable, while using a type explicitly
sets the type of a variable. When you use var
in place of the
type declaration you let the compiler infer the type. But when you use
explicit type, the variable declaration must be of the type you specified.
We use var
to make our developer experience better, simplify, and to increase code readability. On the other hand, explicit typing is closer to the strongly-typed nature of C#.
Var is a language feature that lets us use the var
keyword in place of
variable types. The compiler infers the variable type and replaces it with the
proper type.
C# introduced the var
keyword in C# 3.0 back in 2007. Since then, there has been a heated debate over if we should use VAR or explicit type.
In this article, we'll explore best practices around using VAR vs TYPE.
Should I use VAR or type C#?
Here's my guideline for choosing VAR vs type.
Use VAR when you:
- Immediately assign hard-coded values.
- Set your variable to a newly initialized object (
new
keyword). - Use anonymous types to store temporary data.
- Have declarations that use casting.
Use explicit type when you:
- Want to improve code readability when the type is not clear when looking at the variable declaration.
- Assign a variable based on the return type of a method.
// It's easy to read the type:
var lucky = 3;
var date = DateTime.Now;
var name = "Super";
var items = new Dictionary<int, string>();
// This is redundant:
int lucky = 3;
DateTime date = DateTime.Now;
string name = "Super";
Dictionary<int, string> items = new Dictionary<int, string>();
var person = new { Name = "Mark", Age = 56 };
var product = _databaseContext
.Products
.FirstOrDefault(p => new
{
Id = p.Id,
Title = p.Name
});
Person person = new Person(); // redundant
var person = new Person(); // better
Person person = new(); // the best
new
keyword.var vehicle = (Vehicle)car;
var vehicle = car as Vehicle;
var x = int.Parse("5");
// Don't do this:
var rand = new Random();
var myRandom = rand.Next(); // <-- what's the type?
// This is better:
var rand = new Random();
int myRandom = rand.Next(); // <-- Explicit type int.
When should I not use VAR?
Don't use VAR in the following scenarios because it's difficult to infer the type from usage:
Scenario | Example |
---|---|
Loop conditions | for (var data in countries) |
Inline conditions | var country = countryA ?? countryB; |
Index | var cool = myList[i]; |
Property | var country = croatia.name; |
Method return | var country = GetCountry(); |
Expressions | var result = a + b; |
Popularity of VAR vs Type
A study from Notre Dame University–Louaize (NDU) analyzed 10 different C# projects to compare the usage of implicit and explicit tying (VAR vs TYPE).
The analysis looked at 16,500,000 lines of code and more than 930,000 variables.
They found out that explicit typing is overall used more than implicit typing:
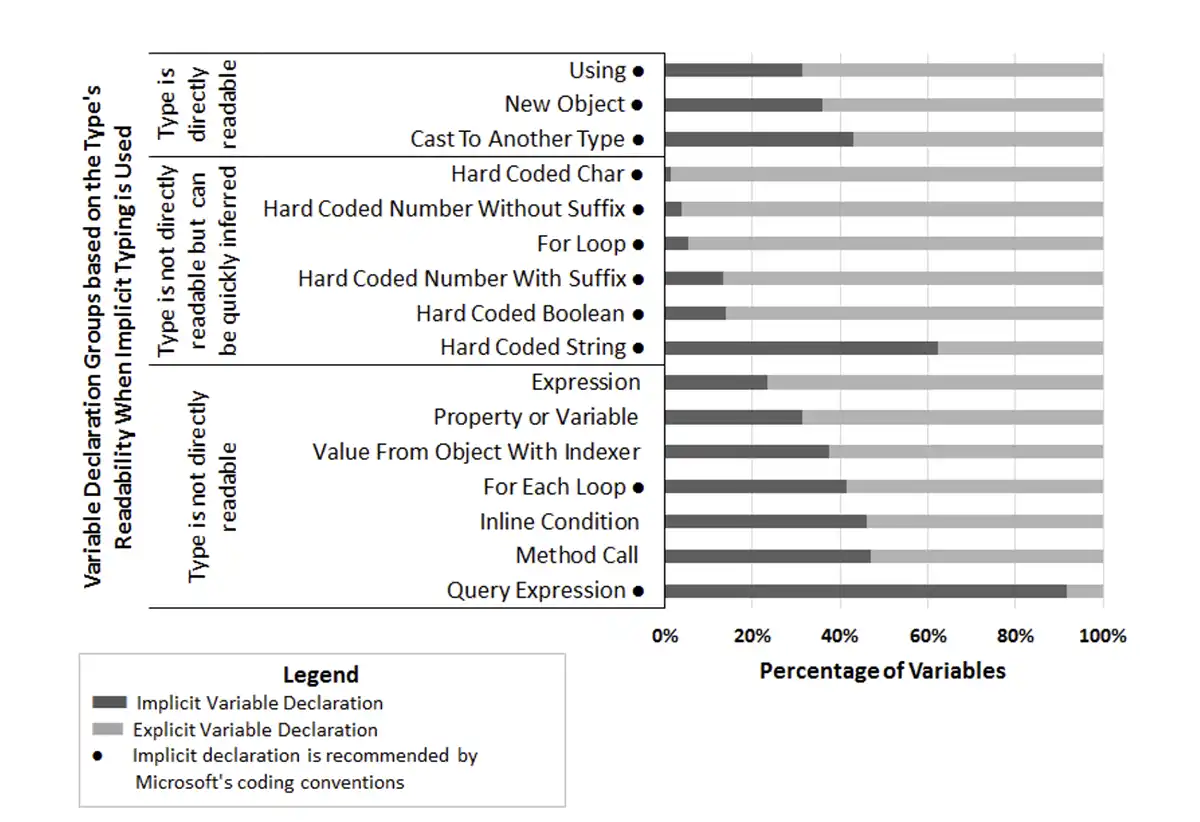
Implicit vs explicit typing usage analysis from
To var or not to var: how do C# developers use and misuse implicit and explicit typing?
.
In the cases where the type is directly readable with implicit typing, 37.29% of the declarations were implicitly typed and 62.71% were explicitly typed. In the cases where the type is not directly readable but can be quickly inferred, 31.06% of the variable declarations were implicitly typed and 68.94% were explicitly typed. In the cases where the type is not directly readable with implicit typing, 42.77% of the declarations were implicitly typed while 57.23% were explicitly typed.
FAQ: VAR vs Type
Does VAR affect performance C#?
Using the var
keyword instead of type doesn't affect performance because compiled code is identical if we use var
or explicit type.
The compiler infers the type anywhere you use the var
keyword.
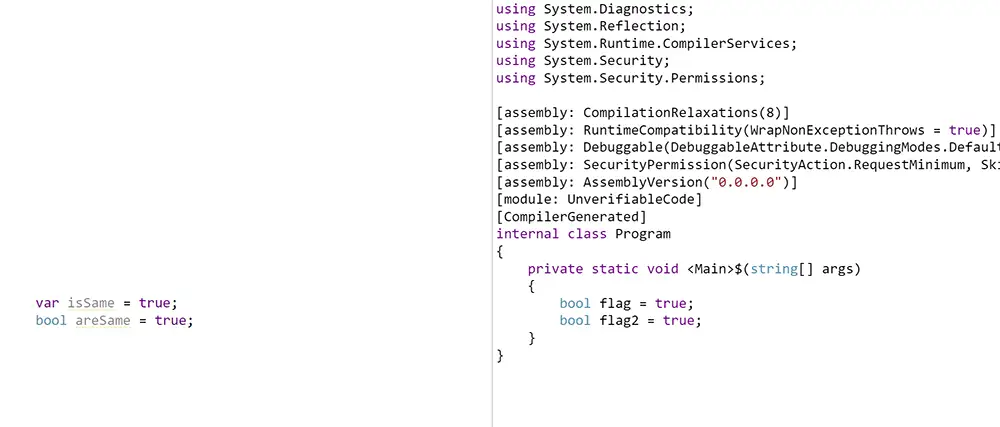
Example showing that we get the same compiled code when we use VAR and TYPE.
Can VAR change type?
Variable declared using var
keyword cannot change the type in C#
If we try to change the type of a variable declared using the var
keyword we'll get a compile error because the C# compiler tries to infer types during compile time.
Why do we use VAR?
VAR lets us omit the variable for local variables. That makes our developer experience better, removes clutter, and increases code readability. VAR lets us focus on the meaning of our code rather than its syntax.
In addition, VAR helps us work with anonymous types because we don't need to create explicit models for the data we receive.
Conclusion
Using VAR over explicit type in C# can help you because it lets you omit the variable type. That can clean up your code and make it more readable.
However, we should be mindful not to overuse it because it can make our code more difficult to understand.
It's best to use VAR where the type is immediately clear or when working with anonymous types.
Remember, VAR does not affect performance, as the code is identical whether explicit typing is used or not.
Check out my article on Object vs Dynamic in C# for more details on types.
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →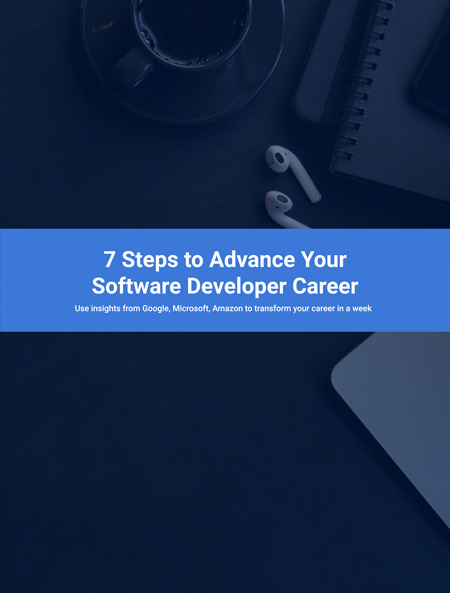
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.