React: Force Component to Re-Render | 4 Simple Ways ⚛️
Quick Tip: Force Re-Render in React
To force React to rerender a specific component update the key
property.
const Home = () => {
const [isHelpMeFind, setIsHelpMeFind] = useState(false);
const [key, setKey] = useState(0)
return (<div key={key}>
<SideBar />
<button onClick={() => setKey(currentKey => currentKey+1)}>Force Render Sidebar</button>
</div>
)
}
Why to force a re-render in React?
The main reason why we want to force a re-render is to update the tree of the React component.
Sometimes it's just easier to reset all the components to the initial state rather than having to reset all the values manually.
Also, forcing a re-render is useful when working with libraries that we don't have control of. Recently, I used the headless UI component for an accordion. The library only lets you specify the default open or close state. After that, there's no way to manually close it. I had to use the force re-render approach to programmatically close the accordion.
Shallow vs Deep Re-Render
Before we learn how to force a re-render, we need to understand the two types of re-rendering in React:
- Shallow re-render involves updating only the parent component and its immediate children, without propagating updates to nested child components.
- Deep re-render involves updating the parent component as well as all its nested child components, propagating the updates through the entire component tree.
In the video below, I show you how changing the component key
prop is different than just updating the state.
Updating the state forces the component to re-render. However, it doesn't reset the inner state of child components. But when we update the key, the entire child tree of components updates to the initial state.
How to force a render in React?
In React, there are 3 main ways to force a component to rerender:
1. Update the key
Prop
To force the entire component tree to re-render, we need to update the key
prop:
const App = () => {
const [key, setKey] = useState(0);
console.log('App rendered');
return (
<div key={key}>
<button onClick={() => setKey((k) => k + 1)}>Force Re-Render</button>
</div>
);
};
We use the useState
hook to manage the key
state variable and its updater function setKey
.
The useState hook initializes "key" to 0. The component renders a button with an onClick
event handler. When we click the button, the state is incremented by 1. Since the key
prop changes, the entire component tree re-renders, forcing an update.
2. Use the useReducer
Hook
const App = () => {
const [_, forceUpdate] = useReducer(x => x + 1, 0);
console.log('App rendered');
return (
<button onClick={() => forceUpdate()}>Shallow Re-Render</button>
);
};
This method uses the useReducer
hook to create a forceUpdate
function.
The useReducer
hook takes two arguments:
- a reducer function (x => x + 1)
- an initial state value (0).
The reducer function simply returns the current state incremented by 1. The forceUpdate
function triggers the reducer when called.
When the state managed by the useReducer
hook changes, the entire component re-renders.
3. Create a Custom Hook
Some devs might not be familiar with how forcing re-render works. Updating the state might look confusing.
One way to make the intent easier to understand is to create a custom hook that re-renders a component:
function useForceUpdate() {
const [, setToggle] = useState(false);
return () => setToggle(toggle => !toggle);
}
const App = () => {
const forceUpdate = useForceUpdate();
console.log('App rendered');
return (
<button onClick={() => forceUpdate()}>Re-Render</button>
);
};
The useForceUpdate
hook toggles state, causing a re-render whenever it's called.
4. Call the forceUpdate
function (Class components only)
If you are still using class components instead of function components, you can call this.forceUpdate();
:
class App extends React.Component {
render() {
console.log('Render')
return <button onClick={() => this.forceUpdate()}>Render</button>
}
}
What are the downsides of forcing rendering of a component?
The biggest downside of forcing rendering is a performance impact.
Forcing a render contradicts React's principles of efficient rendering through state and prop changes.
With each state or prop update, React tries to optimize the DOM tree. It only renders what's necessary.
Even though forcing a re-render is not encouraged, it's not a big deal. In most cases, your app will be fine!
Josip Miskovic is a software developer at Americaneagle.com. Josip has 10+ years in experience in developing web applications, mobile apps, and games.
Read more posts →- Quick Tip: Force Re-Render in React
- Why to force a re-render in React?
- Shallow vs Deep Re-Render
- How to force a render in React?
- 1. Update the key Prop
- 2. Use the useReducer Hook
- 3. Create a Custom Hook
- 4. Call the forceUpdate function (Class components only)
- What are the downsides of forcing rendering of a component?
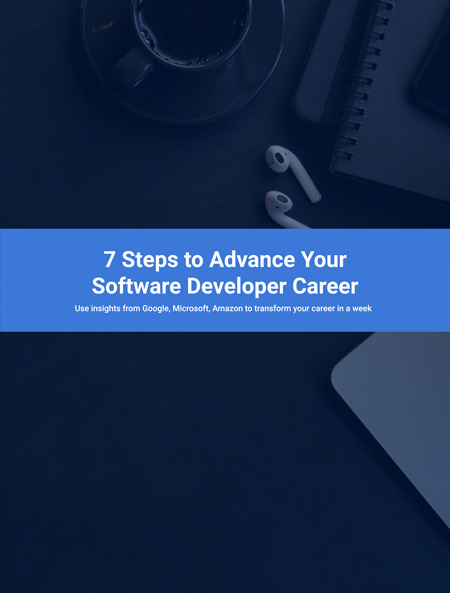
I've used these principles to increase my earnings by 63% in two years. So can you.
Dive into my 7 actionable steps to elevate your career.